1. Printer Automation Operations
(1) Windows System Using<span>win32print</span>
Module (Requires Installation of<span>pywin32</span>
Library)
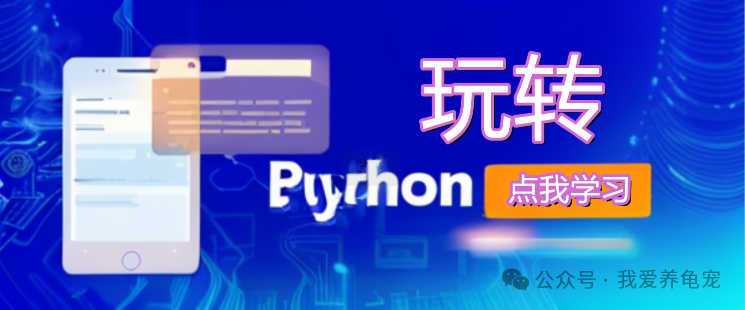
-
Install <span>pywin32</span>
Library
-
Use the <span>pip</span>
command to install the<span>pywin32</span>
library by entering<span>pip install pywin32</span>
in the command line. This library provides access to Windows API, including printer-related operations.
import win32print
default_printer = win32print.GetDefaultPrinter()
print("Default Printer:", default_printer)
def print_text_file(file_path):
try:
hPrinter = win32print.OpenPrinter(default_printer)
try:
hJob = win32print.StartDocPrinter(hPrinter, 1, (file_path, None, "RAW"))
try:
win32print.StartPagePrinter(hPrinter)
with open(file_path, 'r') as file:
text_to_print = file.read()
win32print.WritePrinter(hPrinter, text_to_print.encode('utf-8'))
win32print.EndPagePrinter(hPrinter)
finally:
win32print.EndDocPrinter(hPrinter)
finally:
win32print.ClosePrinter(hPrinter)
except Exception as e:
print("Print Error:", e)
file_path = "test.txt"
print_text_file(file_path)
-
In this function, the default printer is opened using <span>OpenPrinter</span>
, then a print job is started using<span>StartDocPrinter</span>
. The<span>StartPagePrinter</span>
indicates the start of a page print, and after reading the text file content, it is sent to the printer using<span>WritePrinter</span>
. Finally, the page and document printing is concluded with<span>EndPagePrinter</span>
and<span>EndDocPrinter</span>
, and the printer connection is closed with<span>ClosePrinter</span>
.
-
This function can be called to print a text file (assuming the file name is <span>test.txt</span>
):
-
Assuming we have a simple text file to print. Here’s a basic function to print a text file (assuming the text file content is small enough to read the entire content directly):
-
Then, get the name of the default printer:
-
First, import the <span>win32print</span>
module:
(2) Cross-Platform (Including Linux and Mac) Using<span>cups</span>
(Common Unix Printing System)
-
Install <span>pycups</span>
Library (On Systems Supporting<span>cups</span>
)
-
On some Unix or Linux-based systems and Mac systems, you can use <span>cups</span>
to manage print jobs. For Python, you can install the<span>pycups</span>
library to interact with<span>cups</span>
. Use the<span>pip</span>
command to install<span>pycups</span>
(on systems with<span>cups</span>
support):<span>pip install pycups</span>
.
import cups
conn = cups.Connection()
printers = conn.getPrinters()
for printer in printers.keys():
print("Available Printers:", printer)
def print_pdf_file(file_path):
try:
conn = cups.Connection()
default_printer = conn.getDefault()
conn.printFile(default_printer, file_path, "Print Job Name", {})
except Exception as e:
print("Print Error:", e)
file_path = "test.pdf"
print_pdf_file(file_path)
-
In this function, a connection to the <span>cups</span>
server is established, the default printer name is obtained, and then the<span>printFile</span>
method is used to send the specified file to the printer for printing.
-
This function can be called to print a PDF file (assuming the file name is <span>test.pdf</span>
):
-
Assuming you want to print a file (here taking a PDF file as an example, printing other format files is similar), here is a simple print function:
-
Connect to the local <span>cups</span>
server and get the printer list:
-
First, import the <span>cups</span>
module:
2. Scanner Automation Operations
(1) Windows System Using<span>wia</span>
(Windows Image Acquisition)
-
Install <span>pypiwin32</span>
Library (Includes Access to<span>wia</span>
Module)
-
Use <span>pip</span>
to install the<span>pypiwin32</span>
library by entering<span>pip install pypiwin32</span>
in the command line. This library provides access to Windows Image Acquisition (WIA) related functions.
import win32com.client
def scan_image():
try:
wia = win32com.client.Dispatch("WIA.ImageAcquisition")
device = wia.CreateDevice()
item = device.Items[1]
image = item.Transfer()
image.SaveFile("scanned_image.jpg")
except Exception as e:
print("Scan Error:", e)
scan_image()
-
In this function, a <span>WIA.ImageAcquisition</span>
object is created using<span>win32com.client.Dispatch</span>
, then the scanner device is obtained, and one scanning item from the device is selected (assuming it is the second item, which may need to be adjusted according to the actual device). The scanned image is obtained using the<span>Transfer</span>
method, and finally, the image is saved as<span>scanned_image.jpg</span>
.
-
Call this function to scan and save the image:
-
Here’s a simple scanning function:
-
First, import the relevant modules:
(2) Cross-Platform (Partially Supported) Using<span>sane</span>
(Scanner Access Now Easy)
-
Install <span>sane</span>
related Python libraries (if any) and system support (on Linux)
-
On Linux systems, you need to install the <span>sane</span>
package and related library support. For Python, there may be some libraries that can interact with<span>sane</span>
, such as<span>python-sane</span>
(if available). To install the<span>sane</span>
package (on Debian/Ubuntu systems), use the<span>sudo apt-get install sane</span>
command.
try:
import sane
sane.init()
devices = sane.get_devices()
if len(devices) > 0:
scanner = sane.open(devices[0][0])
scanner.start()
image = scanner.snap()
# Here, the scanned image data needs to be saved or processed appropriately, for example, saving as a file
# Assuming saving it as PNG format, additional image processing libraries may be needed to save it correctly
# Below is a simple saving concept example
import png
with open('scanned_image.png', 'wb') as f:
png_writer = png.Writer(width=image.width, height=image.height)
png_writer.write(f, image.get_pixels())
else:
print("No scanner device found")
sane.exit()
except Exception as e:
print("Scan Error:", e)
-
In this code, the <span>sane.init</span>
is called to initialize<span>sane</span>
, retrieve the list of scanner devices, open the first device, start scanning, obtain the scanned image, and attempt to save the image as<span>scanned_image.png</span>
(a simple example using<span>png</span>
module, actual implementation may require a more complete image processing library), and finally, exit<span>sane</span>
with<span>sane.exit</span>
.
-
Here’s a simple example code framework (assuming the <span>python-sane</span>
library has similar functionality):
Notes:
-
Different models of printers and scanners may have different functionalities and configuration requirements. In practical applications, the code may need to be adjusted based on the specific documentation and characteristics of the devices. -
For cross-platform operations, although <span>cups</span>
and<span>sane</span>
provide some universality, there may still be issues with device compatibility and system configuration differences. When deploying automation code for office equipment drivers, thorough testing should be conducted on the target systems and devices.