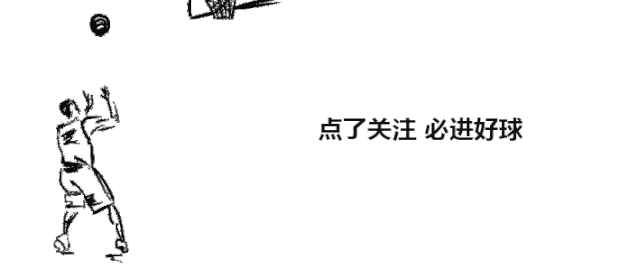
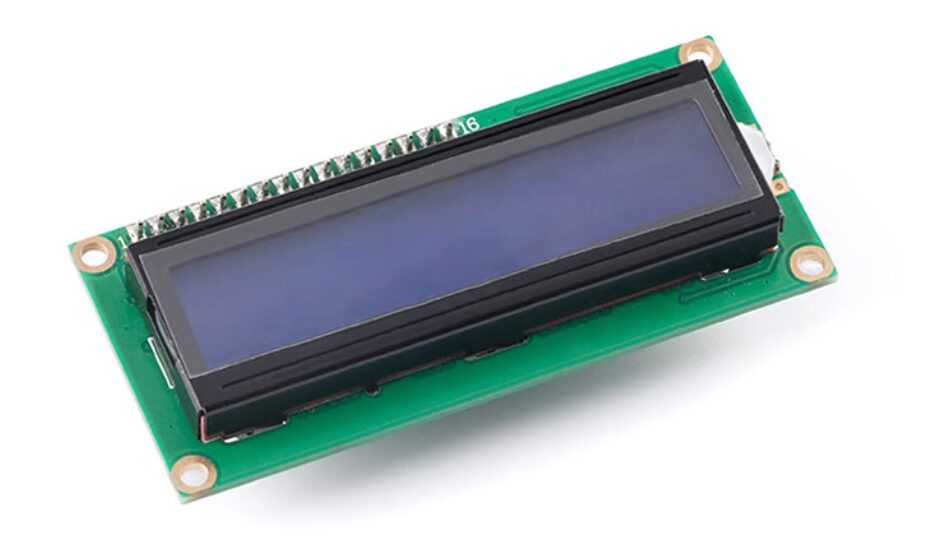
01
Pin Description
02
Software Flowchart
03
Attached Source Code
/**********************************Author: Turnas Electronics Website: https://www.mcude.com Contact: 46580829(QQ) Taobao Store: Turnas Electronics**********************************//**********************************Include Header File**********************************/#include "lcd1602.h"/**********************************Function Definitions**********************************//***********1602 Check Busy Function *****/void lcd1602_check_busy(){ P0=0xff; do { LCD1602_RS=0; LCD1602_RW=1; LCD1602_E=0; LCD1602_E=1; } while(LCD1602_BUSY==1); LCD1602_E=0;}/***********1602 Write Data Busy Function *****/void lcd1602_write_date(uchar date){ lcd1602_check_busy(); LCD1602_E=0; LCD1602_PORT=date; LCD1602_RS=1; LCD1602_RW=0; LCD1602_E=1; LCD1602_E=0;}/***********1602 Write Command Function *****/void lcd1602_write_com(uchar com){ lcd1602_check_busy(); LCD1602_E=0; LCD1602_PORT=com; LCD1602_RS=0; LCD1602_RW=0; LCD1602_E=1; LCD1602_E=0;}/***********LCD1602 Display Character Function *******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* dat: Character to be displayed, input format ' '*****/void lcd1602_display_char(uchar hang, uchar add, uchar dat){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line lcd1602_write_date(dat);}/***********LCD1602 Display String Function *******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* dat: String to be displayed, input format " "*****/void lcd1602_display_str(uchar hang, uchar add, uchar *dat){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line while(*dat != '\0') { lcd1602_write_date(*dat++); }}/***********LCD1602 Display Time Function (Display Format: **:**:**)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* time: Time to be displayed, in seconds*****/void lcd1602_display_time(uchar hang, uchar add, uint time){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line lcd1602_write_date(time/3600/10%10+48); //Hour Tens lcd1602_write_date(time/3600/1%10+48); //Hour Units lcd1602_write_date(':'); // : lcd1602_write_date(time%3600/60/10%10+48); //Minute Tens lcd1602_write_date(time%3600/60/1%10+48); //Minute Units lcd1602_write_date(':'); // : lcd1602_write_date(time%60/10%10+48); //Second Tens lcd1602_write_date(time%60/1%10+48); //Second Units}/***********LCD1602 Display Temperature Function (Display Format: **.*℃)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* temp: Temperature to be displayed*****/void lcd1602_display_temp(uchar hang, uchar add,uint temp){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line lcd1602_write_date(temp/100%10+48); lcd1602_write_date(temp/10%10+48); lcd1602_write_date('.'); lcd1602_write_date(temp/1%10+48); lcd1602_write_date(0xdf); //Display Temperature Degree Symbol, 0xdf is the address code of this symbol in the LCD character library lcd1602_write_date(0x43); //Display "C" Symbol, 0x43 is the address code of Capital C in the LCD character library}/***********LCD1602 Display Humidity Function (Display Format: **.*%)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* humi: Humidity to be displayed*****/void lcd1602_display_humi(uchar hang, uchar add,uint humi){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line lcd1602_write_date(humi/100%10+48); lcd1602_write_date(humi/10%10+48); lcd1602_write_date('.'); lcd1602_write_date(humi/1%10+48); lcd1602_write_date('%');}/***********LCD1602 Display Light Intensity Function (Display Format: **.*lx)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* light: Light Intensity to be displayed*****/void lcd1602_display_light(uchar hang, uchar add,uint light){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line lcd1602_write_date(light/100%10+48); lcd1602_write_date(light/10%10+48); lcd1602_write_date('.'); lcd1602_write_date(light/1%10+48); lcd1602_write_date('L'); lcd1602_write_date('x');}/***********LCD1602 Display Distance Function (Display Format: ***cm)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* distance: Distance to be displayed*****/void lcd1602_display_distance(uchar hang, uchar add,uint distance){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line if(distance > 99) lcd1602_write_date(distance/100%10+48); if(distance > 9) lcd1602_write_date(distance/10%10+48); lcd1602_write_date(distance/1%10+48); lcd1602_write_date('c'); lcd1602_write_date('m'); lcd1602_write_date(' '); lcd1602_write_date(' ');}/***********LCD1602 Display Gas Concentration (Display Format: 000ppm)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* gas_value: Gas Concentration to be displayed*****/void lcd1602_display_gas(uchar hang, uchar add,uint gas_value){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line if(gas_value > 999) lcd1602_write_date(gas_value/1000%10+48); if(gas_value > 99) lcd1602_write_date(gas_value/100%10+48); if(gas_value > 9) lcd1602_write_date(gas_value/10%10+48); lcd1602_write_date(gas_value/1%10+48); lcd1602_write_date('p'); lcd1602_write_date('p'); lcd1602_write_date('m'); lcd1602_write_date(' '); lcd1602_write_date(' '); lcd1602_write_date(' ');}/***********LCD1602 Display PM2.5 (Display Format: 000ug/m3)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* pm25_value: PM2.5 to be displayed*****/void lcd1602_display_pm25(uchar hang, uchar add,uint pm25_value){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line if(pm25_value > 999) lcd1602_write_date(pm25_value/1000%10+48); if(pm25_value > 99) lcd1602_write_date(pm25_value/100%10+48); if(pm25_value >= 9) lcd1602_write_date(pm25_value/10%10+48); lcd1602_write_date(pm25_value/1%10+48); lcd1602_write_date('u'); lcd1602_write_date('g'); lcd1602_write_date('/'); lcd1602_write_date('m'); lcd1602_write_date('3'); lcd1602_write_date(' ');}/***********1602 Display Number (2 Digits)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* num: Data to be displayed, 2 digits*****/void lcd1602_display_num_2(uchar hang, uchar add, uint num){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line lcd1602_write_date(num/10%10+48); //Tens lcd1602_write_date(num/1%10+48); //Units}/***********LCD1602 Display Number Function (Maximum 4 Digits)*******Parameter Definition: hang: Input Display Line 1 First Line 2 Second Line******* add: Offset, 0-15******* num: Data to be displayed, maximum 4 digits*****/void lcd1602_display_num(uchar hang, uchar add, uint num){ if(hang==1) lcd1602_write_com(0x80+add); //First Line else lcd1602_write_com(0x80+0x40+add); //Second Line if(num > 999) lcd1602_write_date(num/1000%10+48); //Thousands if(num > 99) lcd1602_write_date(num/100%10+48); //Hundreds if(num > 9) lcd1602_write_date(num/10%10+48); //Tens lcd1602_write_date(num/1%10+48); //Units lcd1602_write_date(' '); lcd1602_write_date(' '); lcd1602_write_date(' ');}/***********LCD1602 Display Time Function*******Format: First Line: Year-Month-Day*************Second Line: Hour:Minute:Second Week (Abbreviation in English)*******Parameter Definition: time_buf[8]: Time Array*****/void lcd_display_alltime(uchar time_buf[8]){ uint nian; uchar yue,ri,shi,fen,miao,xingqi; nian = time_buf[0]/0x10*1000 + time_buf[0]%0x10*100 + time_buf[1]/0x10*10 + time_buf[1]%0x10; yue = time_buf[2]/0x10*10 + time_buf[2]%0x10; ri = time_buf[3]/0x10*10 + time_buf[3]%0x10; shi = time_buf[4]/0x10*10 + time_buf[4]%0x10; fen = time_buf[5]/0x10*10 + time_buf[5]%0x10; miao = time_buf[6]/0x10*10 + time_buf[6]%0x10; xingqi = time_buf[7]/0x10*10 + time_buf[7]%0x10; lcd1602_display_num(1,0,nian); //Display Year lcd1602_write_com(0x84); lcd1602_write_date('-'); //Display - lcd1602_display_num_2(1,5,yue); //Display Month lcd1602_write_date('-'); //Display - lcd1602_display_num_2(1,8,ri); //Display Day lcd1602_display_num_2(2,2,shi); //Display Hour lcd1602_write_date(':'); //Display : lcd1602_display_num_2(2,5,fen); //Display Minute lcd1602_write_date(':'); //Display : lcd1602_display_num_2(2,8,miao); //Display Second if(xingqi == 1) { lcd1602_display_str(2,11,"Mon "); //Display Monday abbreviation } else if(xingqi == 2) { lcd1602_display_str(2,11,"Tues"); //Display Tuesday abbreviation } else if(xingqi == 3) { lcd1602_display_str(2,11,"Wed "); //Display Wednesday abbreviation } else if(xingqi == 4) { lcd1602_display_str(2,11,"Thur"); //Display Thursday abbreviation } else if(xingqi == 5) { lcd1602_display_str(2,11,"Fri "); //Display Friday abbreviation } else if(xingqi == 6) { lcd1602_display_str(2,11,"Sat "); //Display Saturday abbreviation } else { lcd1602_display_str(2,11,"Sun "); //Display Sunday abbreviation }} /***********LCD1602 Clear Screen Function *****/void lcd1602_clean(){ lcd1602_write_com(0x01);}/***********LCD1602 Initialization Function *****/void Lcd1602_Init(){ lcd1602_write_com(0x38); lcd1602_write_com(0x0c); lcd1602_write_com(0x06); lcd1602_write_com(0x01);}
/**********************************Author: Turnas Electronics Website: https://www.mcude.com Contact: 46580829(QQ) Taobao Store: Turnas Electronics**********************************/#ifndef _LCD1602_H_#define _LCD1602_H_/**********************************Include Header File**********************************/#include "main.h"/**********************************PIN Definition**********************************/#define LCD1602_PORT P0 //Data Bus P0.0-P0.7 sbit LCD1602_RS=P2^5; //Data/Command Selection Pin P2.5 sbit LCD1602_RW=P2^6; //Read/Write Selection P2.6 sbit LCD1602_E=P2^7; //Enable Signal Pin P2.7 sbit LCD1602_BUSY=P0^7; //Status Detection Pin P0.7/**********************************Function Declarations**********************************/void lcd1602_display_char(uchar hang, uchar add, uchar dat); //LCD1602 Display Character Function void lcd1602_display_str(uchar hang, uchar add, uchar *dat); //LCD1602 Display String Function void lcd1602_display_time(uchar hang, uchar add, uint time); //LCD1602 Display Time Function (Display Format: **:**:**) void lcd_display_alltime(uchar time_buf[8]); //LCD1602 Display Time Function (Display Year Month Day Hour Minute Second Week) void lcd1602_display_temp(uchar hang, uchar add,uint temp); //LCD1602 Display Temperature Function (Display Format: **.*℃) void lcd1602_display_humi(uchar hang, uchar add,uint humi); //LCD1602 Display Humidity Function (Display Format: **.*%) void lcd1602_display_light(uchar hang, uchar add,uint light); //LCD1602 Display Light Intensity Function (Display Format: **.*Lx) void lcd1602_display_distance(uchar hang, uchar add,uint distance); //LCD1602 Display Distance Function (Display Format: ***cm) void lcd1602_display_gas(uchar hang, uchar add,uint gas_value); //LCD1602 Display Gas Concentration (Display Format: 000ppm) void lcd1602_display_pm25(uchar hang, uchar add,uint pm25_value); //LCD1602 Display PM2.5 (Display Format: 000ug/m3) void lcd1602_display_num(uchar hang, uchar add,uint num); //LCD1602 Display Number Function (Maximum 4 Digits) void lcd1602_clean(); //LCD1602 Clear Screen Function void Lcd1602_Init(); //LCD1602 Initialization Function void lcd1602_display_num_2(uchar hang, uchar add, uint num);#endif
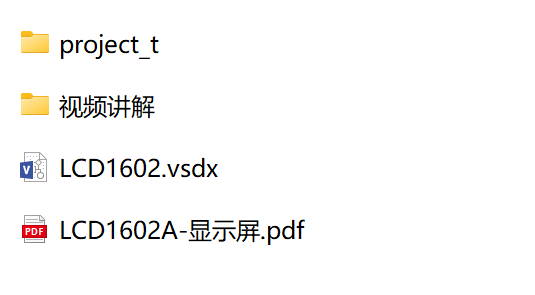
Click to read the original text to obtain the article.


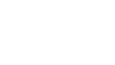
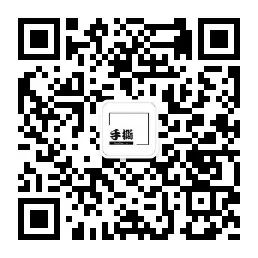
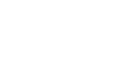
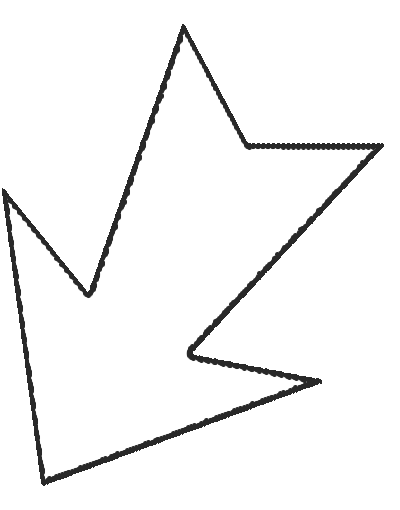
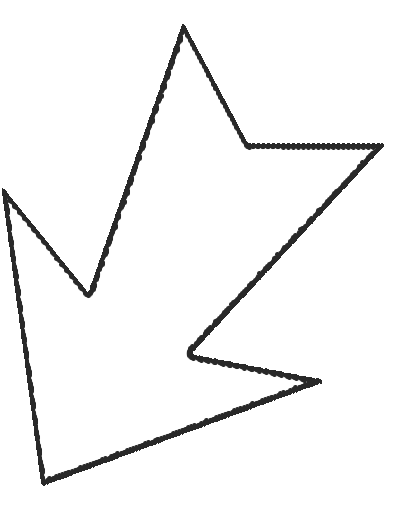