What! You also like planting vegetables? I’m not talking about these:
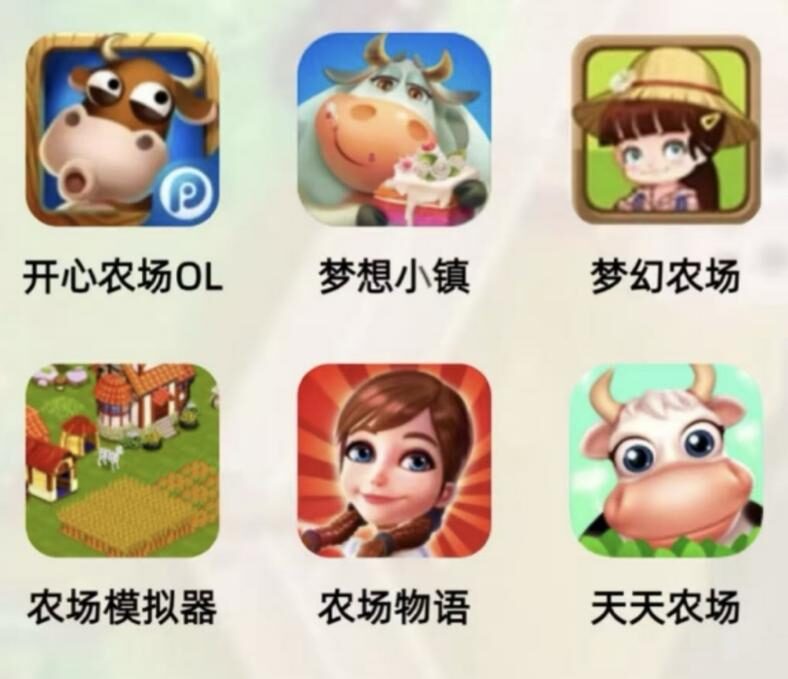
Who doesn’t have hundreds of acres in the game? Roll up your sleeves and get to work, but it’s never enough, it can never be enough.
However, in real life, when most of us face a small plant:
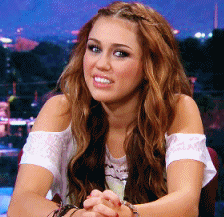
As we all know……
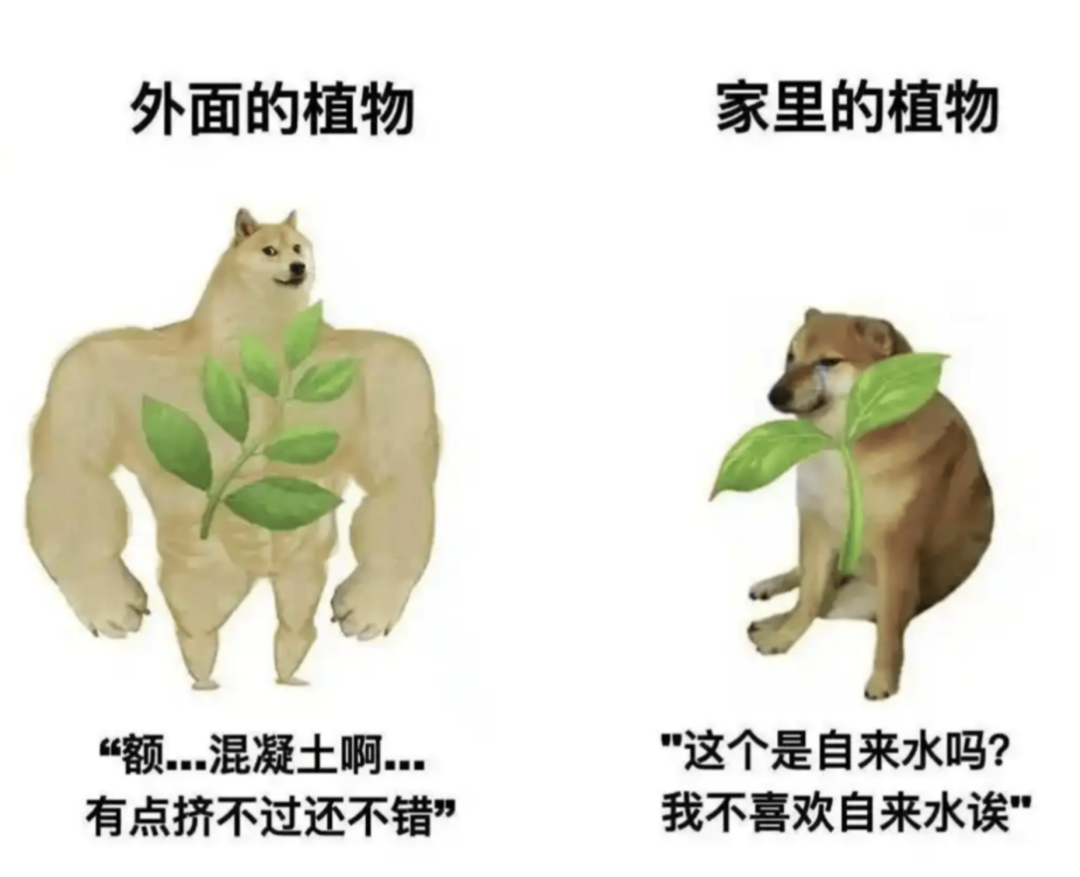
If you can’t raise it well, then don’t raise it……
No……
Then raise it well!
Let’s build a greenhouse for them.
Hardware & Tools
-
Arduino Nano R3 x1 -
Arduino 4-channel relay expansion board x1 -
Ultrasonic Sensor – HC-SR04 (Universal) x1 -
PIR Motion Sensor (Universal) x1 -
LED (Universal) x1 -
Adafruit Standard LCD – 16×2 Blue Background White Characters (I2C interface soldered on top) x1 -
DFRobot Gravity: Analog Capacitive Soil Moisture Sensor – Corrosion Resistant x2 -
RobotGeek DC Liquid Pump – Large x2 -
Liquid Valve x1 -
Water Tank x1 -
DHT22 Temperature Sensor x1 -
Soldering Iron (Universal)
Main Structure: Cabinet (Add shelves as needed)
The image below shows a portable wardrobe from IKEA, wrapped inside and outside with foam insulation. It has three layers, with the lowest layer mainly for seed starting: there is a heating pad, and the other two layers are for the plants.
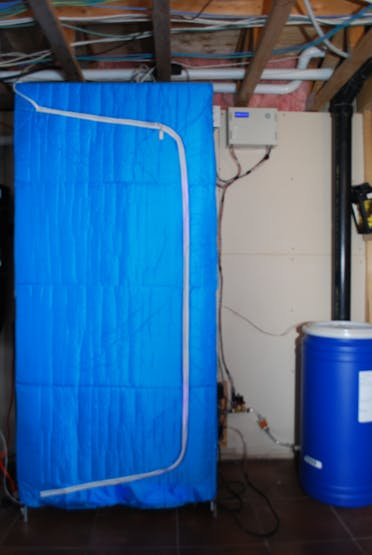
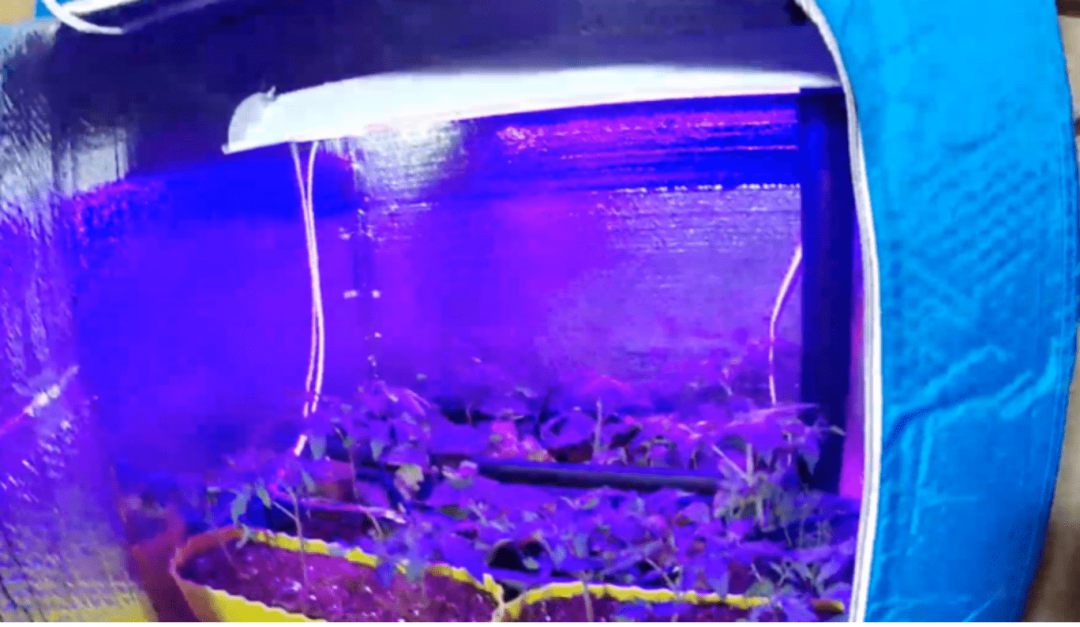
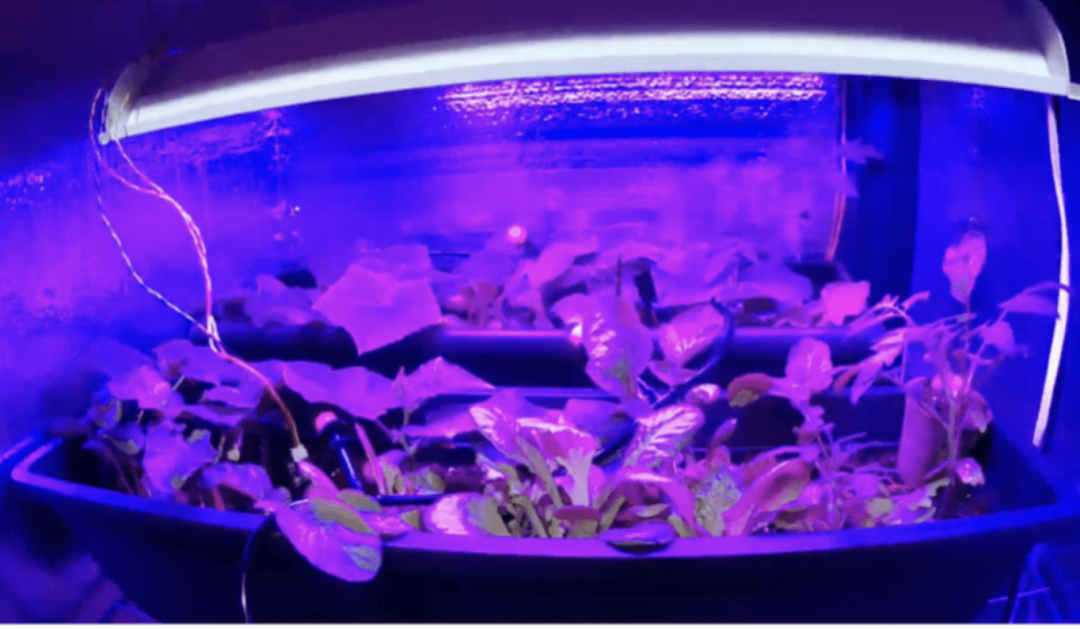
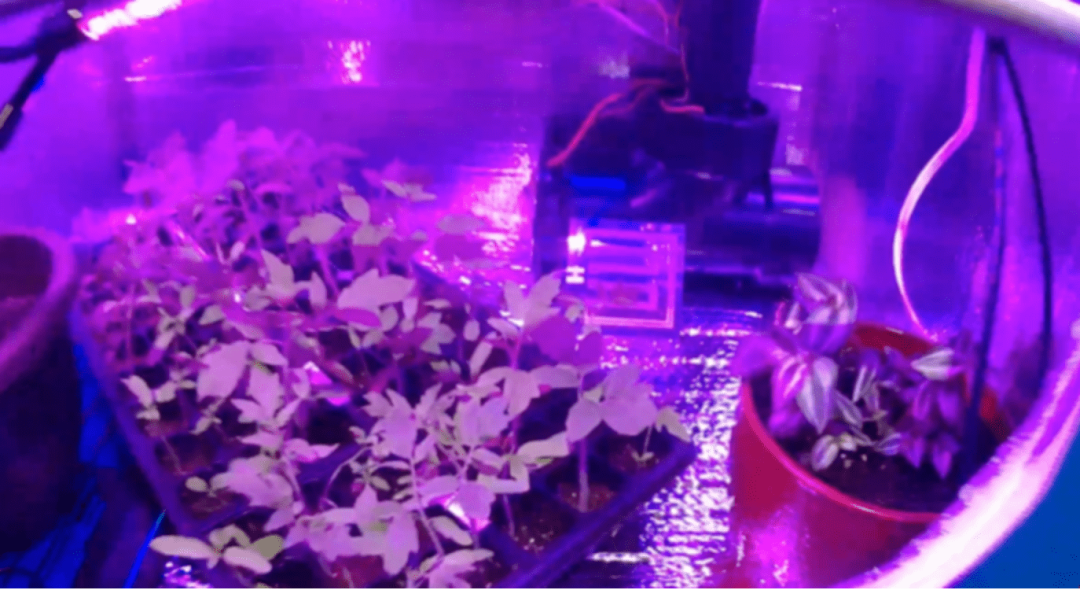
Air circulation, heating, and temperature and humidity measurement are all done through an ABS pipe “heater”. The DHT22 sensor is used to measure temperature and humidity.
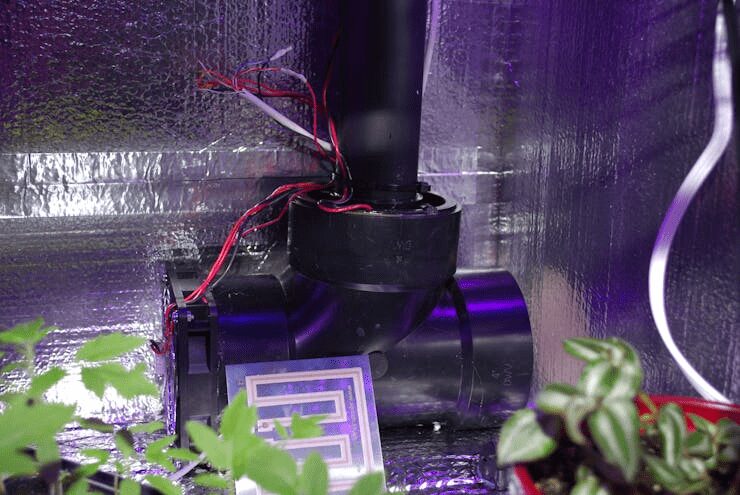
Humidity control is done by a small 12V “squirrel cage” fan.
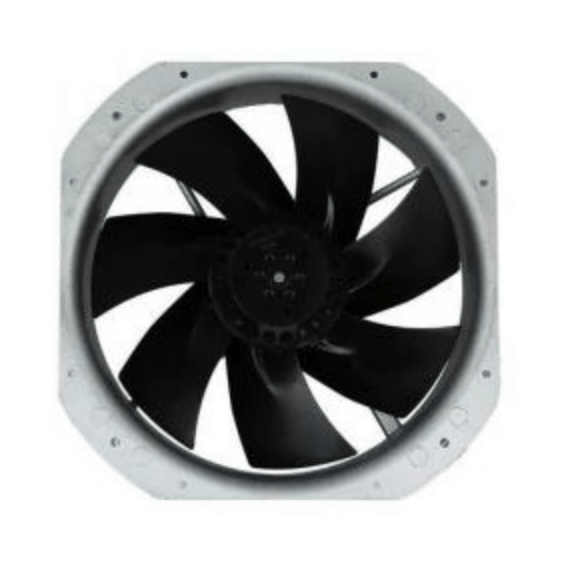
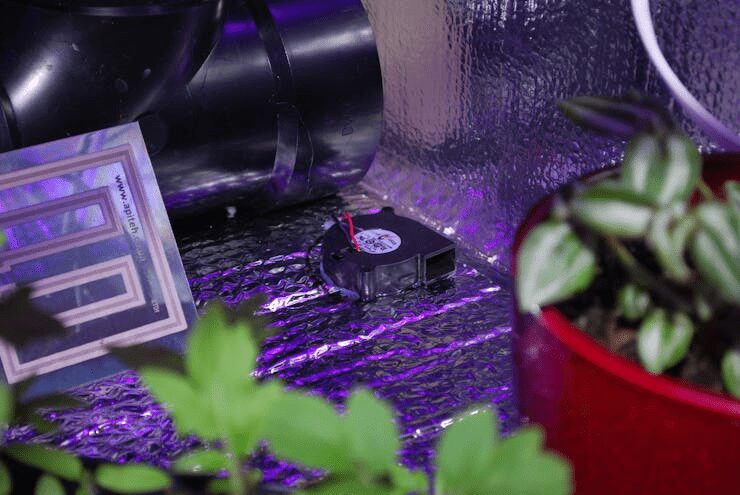
An Arduino Nano (compatible board) serves as the core of the control unit. The system is equipped with an I2C interface LCD to display all necessary parameters and statuses. When someone enters the area, the infrared motion sensor triggers the backlight of the LCD screen.
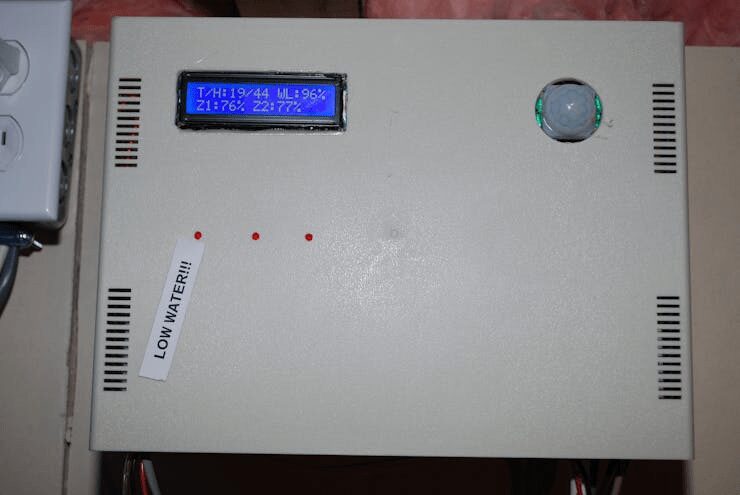
The system has an RTC relay clock that provides a “day-night” reference signal for the entire system through a digital pin. The internal temperature is set with “night” and “day” set points to simulate real environmental conditions. During the “day” period, the lights will remain on for about 15 hours.
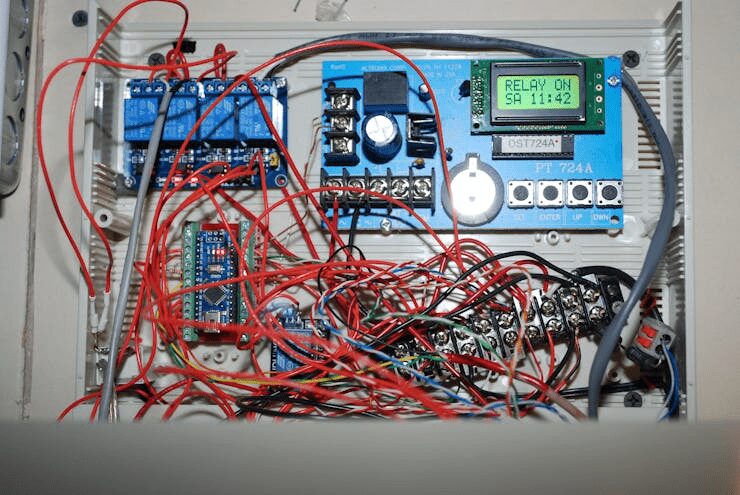
Irrigation System
A rainwater (snowmelt) barrel is connected to a dual pump group through an electromagnetic valve and filter. The water level is measured by an ultrasonic sensor. Two capacitive soil moisture sensors monitor the humidity of their respective areas independently and trigger the water pump to operate. When the water level in the barrel drops to a critical point, irrigation automatically stops, and an LED light displays a “low water level” warning.
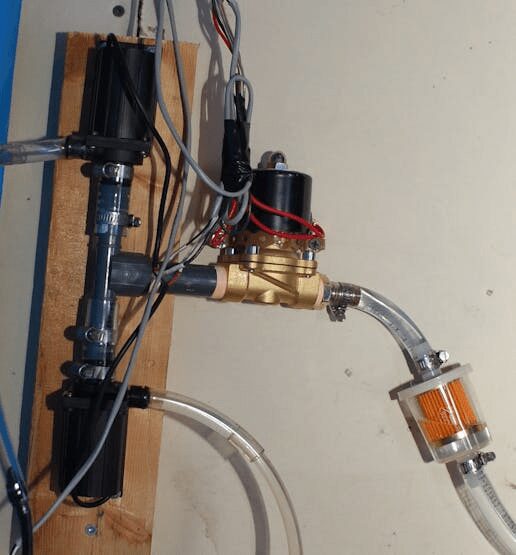
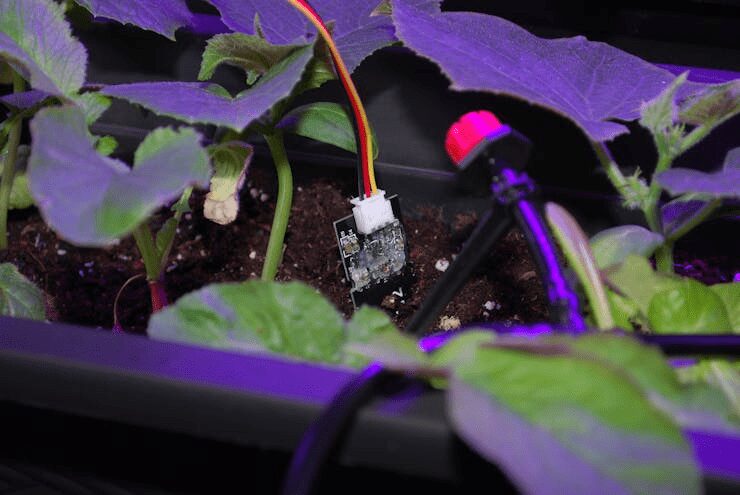
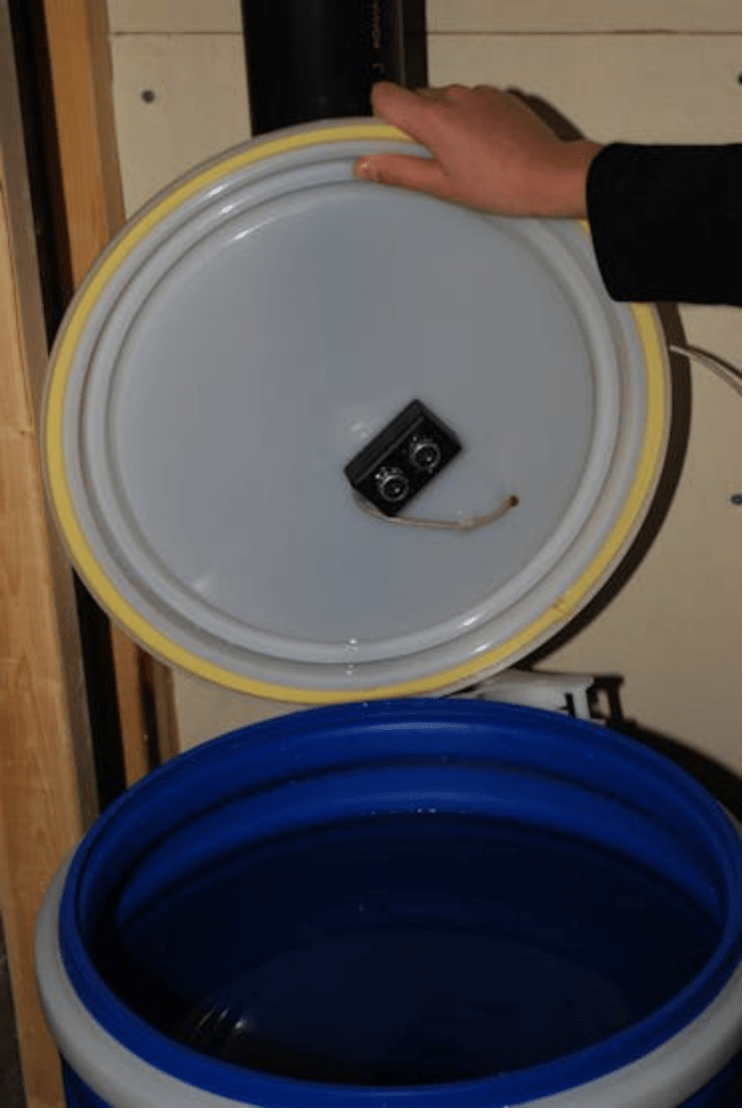
Code
//===GreenHouse sketch created by Victor Onofrei. 2019===
#include <DHT.h>
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <HCSR04.h>
#include <SandTimer.h>
#define DHTPIN 2
#define DHTTYPE DHT22
DHT dht(DHTPIN, DHTTYPE);
LiquidCrystal_I2C lcd(0x27,20,4);
HCSR04 watersens(5,6);
SandTimer timersensor;
SandTimer backlightimer;
SandTimer pump1timer;
SandTimer pump2timer;
const int lowaterled=4;
const int venthum=7;
const int irsensor=8;
const int pump2=9;
const int heaterpin=10;
const int lightpin=11;
const int pump1=12;
const int timerpin=13;
int h=0;
int t=0;
int Soil1=0; //lower soil moisture sensor
int Soil2=0;
int settemp;
int daytemp=26;
int nightemp=23;
int timervalue;
int motiondetect;
int waterlevel;
void setup() {
dht.begin();
lcd.init();
timersensor.start(2000);
backlightimer.start(30000);
pump1timer.start(10000);
pump2timer.start(10000);
pinMode(heaterpin, OUTPUT);
pinMode(timerpin, INPUT);
pinMode(lightpin, OUTPUT);
pinMode(lowaterled, OUTPUT);
pinMode(venthum, OUTPUT);
pinMode(irsensor, INPUT);
pinMode(pump1, OUTPUT);
pinMode(pump2, OUTPUT);
digitalWrite(pump1, HIGH);
digitalWrite(pump2, HIGH);
}
void loop() {
sensorcheck();
lowater();
settempset();
humidcontrol();
runheat();
runlights();
lcdprint();
lcdbacklight();
watering();
}
//===========Functions=============
void sensorcheck(){
if (timersensor.finished()){
h=dht.readHumidity();
t=dht.readTemperature();
timervalue=digitalRead(timerpin);
Soil1=analogRead(6);
Soil2=analogRead(7);
Soil1=map(Soil1,560,270,01,99);
Soil2=map(Soil2,545,270,01,99);
motiondetect=digitalRead(irsensor);
waterlevel=watersens.dist();
waterlevel=map(waterlevel,3,57,99,01);
timersensor.startOver();
}
}
void lowater(){
if (waterlevel<=3){
digitalWrite(lowaterled, HIGH);
}
else{
digitalWrite(lowaterled, LOW);
}
}
void watering(){
if ((waterlevel>=3)&&(Soil1<=24)){
digitalWrite(pump1, LOW);
// if (pump1timer.finished()){
// digitalWrite(pump1, HIGH);
// pump1timer.startOver();
// }
}
else{
digitalWrite(pump1, HIGH);
}
if ((waterlevel>=3)&&(Soil2<=24)){
digitalWrite(pump2, LOW);
// if (pump2timer.finished()){
// digitalWrite(pump2, HIGH);
// pump2timer.startOver();
// }
}
else {
digitalWrite(pump2, HIGH);
}
}
void settempset(){
if (timervalue==HIGH){
settemp=daytemp;
}
else{
settemp=nightemp;
}
}
void lcdbacklight(){
if (motiondetect==1){
lcd.backlight();
}
else if ((motiondetect==0)&&(backlightimer.finished())){
lcd.noBacklight();
backlightimer.startOver();
}
}
void humidcontrol(){
if(h>=72){
digitalWrite(venthum, HIGH);
}
else if (h<=65){
digitalWrite(venthum, LOW);
}
}
void runheat(){
if(t>=settemp){
digitalWrite(heaterpin, HIGH);
}
else{
digitalWrite(heaterpin, LOW);
}
}
void runlights(){
if (timervalue==LOW){
digitalWrite(lightpin, HIGH);
}
else{
digitalWrite(lightpin, LOW);
}
}
void lcdprint(){
lcd.setCursor(0,0);
lcd.print("T/H:");
lcd.print(t);
lcd.print("/");
lcd.print(h);
lcd.setCursor(10,0);
lcd.print("WL:");
lcd.print(waterlevel);
lcd.print("%");
lcd.setCursor(0,1);
lcd.print("Z1:");
lcd.print(Soil1);
lcd.print("%");
lcd.print(" ");
lcd.print("Z2:");
lcd.print(Soil2);
lcd.print("%");
}
Go save your plants now.
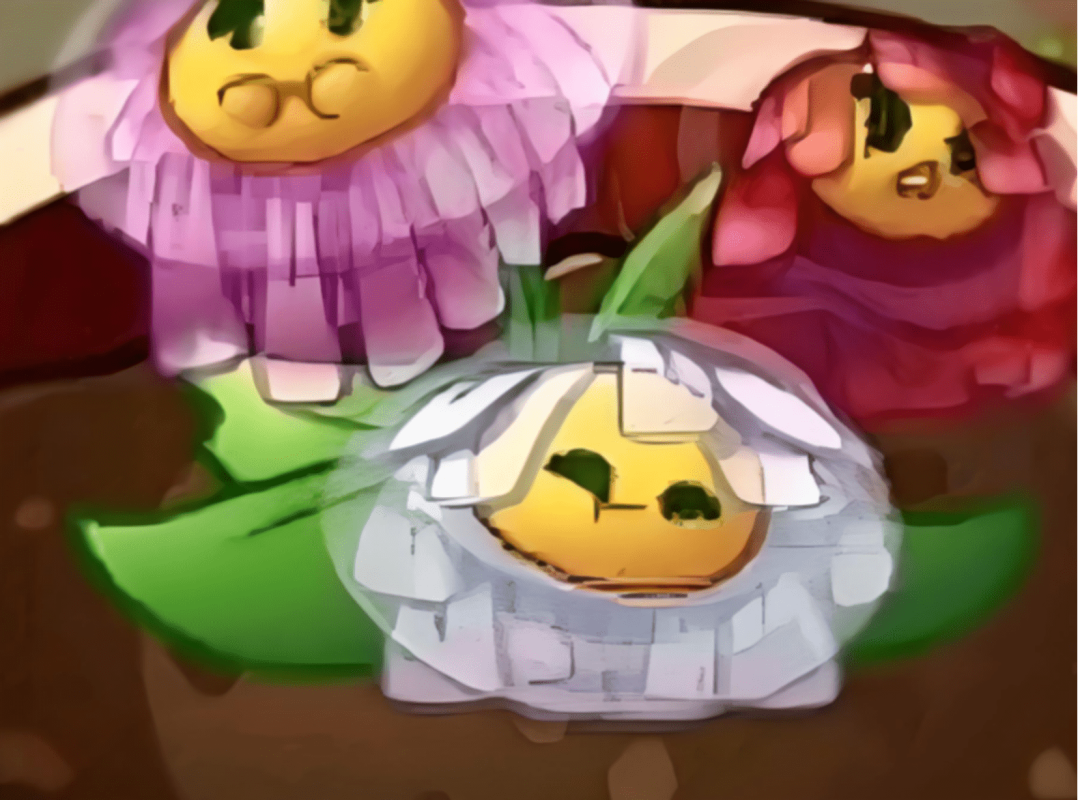
Original article: https://www.hackster.io/vinikon/autonomous-indoor-greenhouse-mature-real-working-project-946f6e
Project Author: Victor Onofrei
Translation first published in: DF Maker Community
Please indicate the source when reprinting
Hardware Arsenal
Long press to scan the QR code for details👆
DFRobot Official Brand Store https://dfrobot.taobao.com/
DFRobot Official Flagship Store https://dfrobot.jd.com/
If anyone has something to say, feel free toleave a message!
Past Project Review
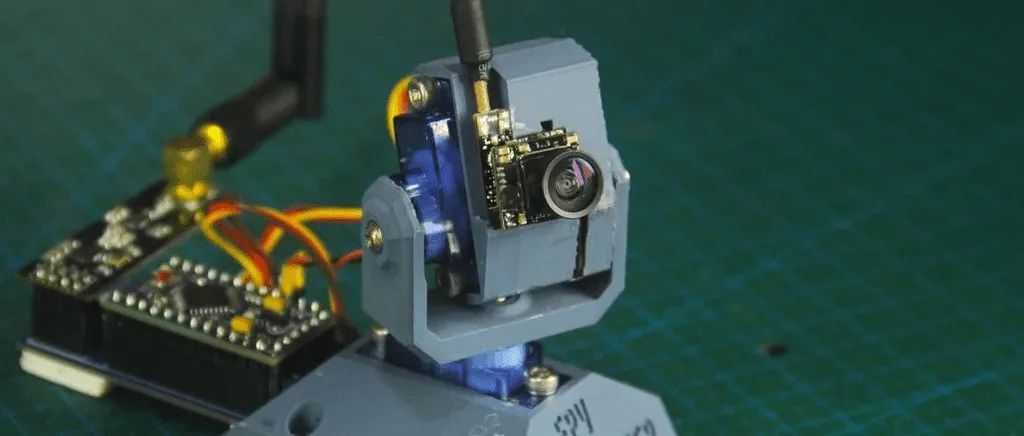
FPV Head Tracking Camera System, Cat Night Light, Smart Robot that Tells You Room Health Status|DF Maker Weekly (Issue 110)
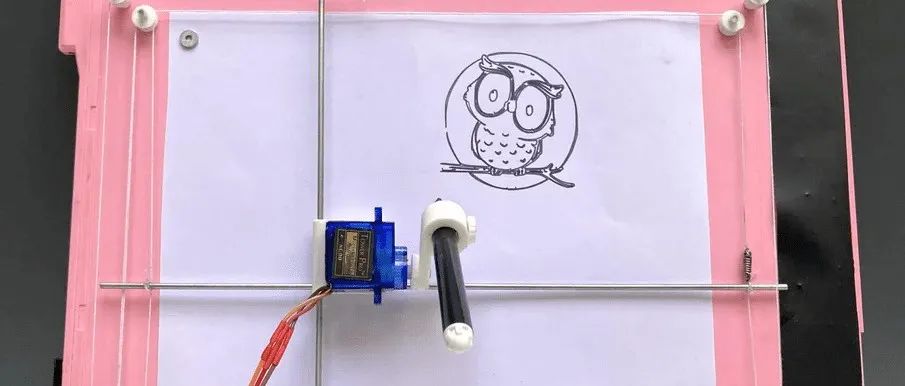
The keyboard is the computer, Raspberry Pi 500 is here!
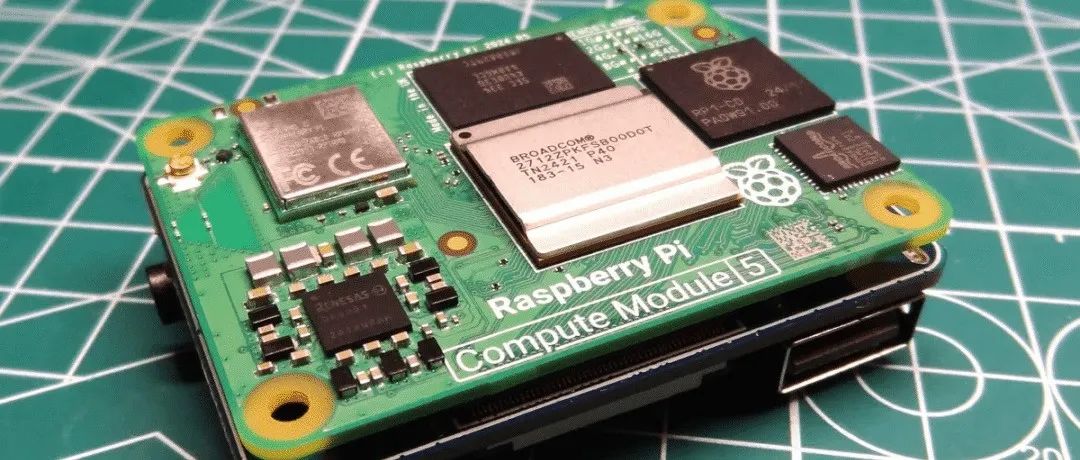
Homemade Phosphorescent Detector, Small Plotter Controlled by Arduino Nano, Starry Sky Projector|DF Maker Weekly (Issue 109)
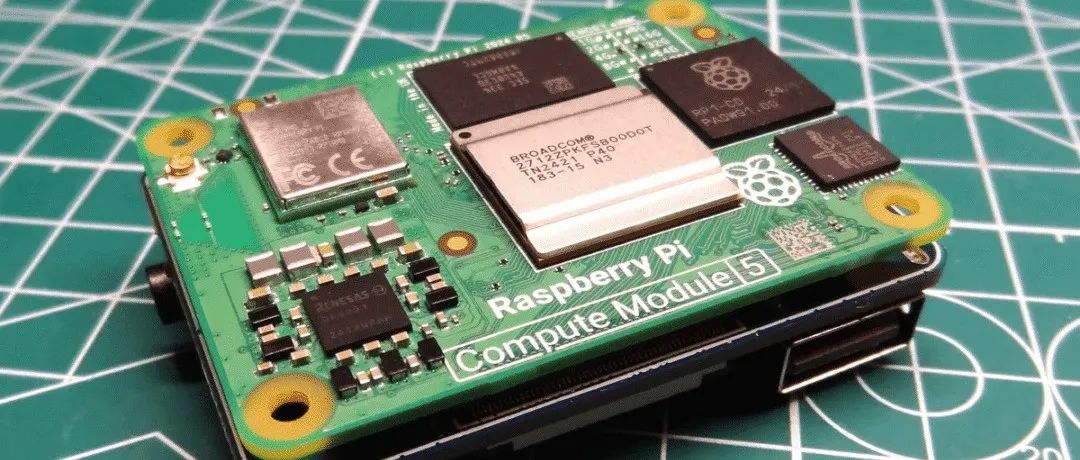
Raspberry Pi CM5 Review: Same Appearance, Stronger Performance
Click to read👆