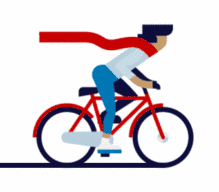
Click on the blue text above to follow us
If you’re interested, please visit CSDN to check out the in-depth FreeRTOS series of articles
Currently, there are three common embedded software system architectures: polling system architecture, front and back-end system architecture, and multi-task system architecture.
1
Polling System Architecture
The polling system architecture is the simplest software structure, where the main program is an infinite loop of code that sequentially checks various conditions, executing corresponding operations if satisfied.
The advantage of this solution is its simple implementation and clear logic, making it easy for developers to grasp. However, the time for querying and processing each event is indeterminate; if the previous operation takes a long time, the subsequent operations will inevitably be delayed.
As shown in the figure below, if step 1 takes a long time, then step 2 will not be processed in a timely manner. If step 2’s work is very important or urgent, then the system’s performance and responsiveness will be poor.
The sample pseudocode is as follows:
int main(void){ HwInit(); // Initialize peripherals while(1) { statement_1; // Statement 1 statement_2; // Statement 2 statement_3; // Statement 3 ...... } return 0;}
2
Front and Back-End System Architecture
Compared to the polling system architecture, the front and back-end system architecture optimizes the handling of external events and is interrupt-driven.
The main program is still an infinite loop of code, referred to as the back-end program, while the response to events is completed by interrupts, referred to as the front-end program.
When the back-end program is executing, if an external event occurs, the front-end interrupt program will interrupt the back-end program. After completing the necessary event response, the front-end interrupt program exits and notifies the back-end program to continue operations, with the back-end program completing the subsequent processing of the event.
Functionally, the response and handling of events are divided into two parts. Since interrupts have priority and nesting capabilities, higher-priority events can receive timely responses. However, the back-end program still needs to process the subsequent transactions of each event in order.
There is a concept of priority among interrupt sources, where the ISR will respond to events first. Simple events can be handled directly in the ISR, while complex cases will record necessary data and status flags, to be processed in order by the back-end main function after all interrupts are handled. It can also be understood that while the response to events supports priorities, the final handling of events is sequential.
Using interrupts instead of polling for event queries significantly improves the responsiveness to events.
The sample pseudocode is as follows:
int main(void){ HwInit(); // Initialize peripherals while(1) { statement_1; // Statement 1 statement_2; // Statement 2 statement_3; // Statement 3 ...... } return 0;}
void interrupt_event(void){ statement_4; // Statement 4 return 0;}
3
Multi-Task System Architecture
Relative to the front and back-end system architecture, the multi-task system architecture also completes event response through multiple interrupt handlers. However, the subsequent operations of events are handled by multiple tasks, meaning each task handles the events it is responsible for.
In a priority-based multi-task system architecture, due to the existence of high and low priority relationships between tasks, higher-priority tasks can interrupt the execution of lower-priority tasks to gain priority access to the CPU, allowing high-priority events to be processed in a timely manner. In a time-sharing multi-task system, tasks take turns using the processor in proportion.
Because the multi-task system architecture allows specific application systems to be divided into several relatively independent tasks for management, using a multi-task operating system can simplify application program design, making the system simpler and easier to maintain and expand. Events with strict real-time requirements can be processed reliably and promptly. However, multi-task operating systems themselves consume more processor, memory, and other hardware resources, which is the necessary cost of introducing multi-task mechanisms.
The sample pseudocode is as follows:
int main(void){ HwInit(); // Initialize peripherals OS_Init(); // Initialize system OS_Start(); // Run kernel scheduling while(1) { ...... } return 0;}
// Task 1
void task_1(void){ task_statement_1; task_statement_2; task_statement_3; OS_delay();}
// Task 2
void task_2(void){ task_statement_1; task_statement_2; task_statement_3; OS_delay();}
// Task 3
void task_3(void){ task_statement_1; task_statement_2; task_statement_3; OS_delay();}
4
Differences
Finally, let’s compare the characteristics and differences of the three embedded software system architectures, as shown in the table below:
This column will begin the study of the FreeRTOS multi-task operating system; interested friends, please stay tuned~
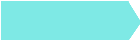
-
What is automotive-grade chips?
-
In-depth analysis of CAN bus: CAN protocol layered structure and functions
-
LabVIEW water tank flow control system
-
LabVIEW color matching for color recognition and inspection
-
How to design a bridge sensor driver circuit?
-
Working with a 12-year-old on maker development: two treasures for detecting button states
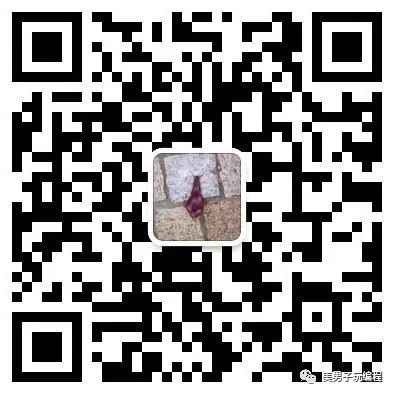
