In C/C++ cross-platform development, we know that we can perform step-by-step breakpoint debugging on Windows using VS, which is very convenient. But how can we track the process and various stages of a compiled dynamic library (SO), such as tracking the internal demux process of libraries like FFmpeg/VLC, or the Codec process? Today, we will debug the C/C++ code of the library file through a small demo.
1. Download NDK and Build Tools
To compile and debug native code, you need the following components:
-
1. The Android Native Development Kit (NDK): A toolkit that allows you to use C and C++ code on Android.
-
2. CMake external build tools. If you only plan to use ndk-build, you can skip this.
-
3. LLDB: The craftsman for debugging native code on Android Studio.
Note: To use CMake or ndk-build in Android Studio, you need to use Android Studio 2.2 or higher, and you also need to work with Android Plugin for Gradle version 2.2.0 or above.
Install the above components:
-
If SDK Tools do not display LLDB, CMake, and NDK, it indicates that you have configured a domestic mirror site!
-
You need to access the Google official website for them to display; previously, I also used a domestic mirror, and they never showed up!
Tools > Android > SDK Manager > Click on the SDK Tools tab. Check LLDB, CMake, and NDK.
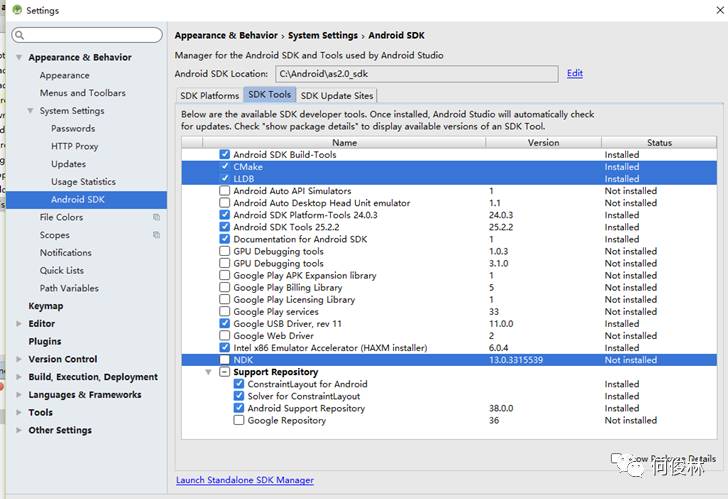
Since I have separately downloaded android-ndk-r11b, I will not download NDK here! (It is best to check and download the latest NDK) Click Apply, then click OK.
2. Create a New Project:
1. Check include C++ support
2. You can choose different C++ libraries in the last step
Note: Although it supports C/C++, it does not fully support all features of C/C++, and must follow the JNI conventions: specific references can be made to the JNI manual C++ Standard: Choose C++ Library
-
Toolchain Default: Default CMake settings
-
C++ 11: Supports C++11 features!
-
Exceptions Support (-fexceptions):
If you want to use support for C++ exception handling, check it. After checking, Android Studio will add the -fexceptions flag in the cppFlags of the build.gradle file at the module level.
-
Runtime Type Information Support (-frtti): If you want to support RTTI, check it. After checking, Android Studio will add the -frtti flag in the cppFlags of the build.gradle file at the module level.
Note:
-
native-lib.cpp: Automatically generated C++ source file
-
CMakeLists.txt: The CMake script compiles a C++ source file according to the instructions in the build script, which is native-lib.cpp, and throws the compiled product into a shared object library named libnative-lib.so, which Gradle then packages into the APK.
-
External Build Files: The place where CMake or ndk-build build scripts are stored. Similar to how the build.gradle file tells Gradle how to compile your APP, CMake and ndk-build also require a script to inform how to compile your native library.
1. Just configure the buildTypes under the android tag node
buildTypes{
debug{
jniDebuggable true
jniDebuggable = true
}
}
2. Configure the AndroidManifest attribute: android:debuggable=”true”
<application android:label="@string/app_name"
android:debuggable="true">
<activity android:name=".HelloJni"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
3. Configure Run/Debug configurations

-
a. Select the run dropdown item, select Edit Configurations, and a configuration window will pop up
-
b. Click + to create a new configuration, select the android native entry
-
c. Configure the native execution name, such as: hellojniNative.
-
d. Choose to debug on a real device or emulator.
Breakpoint debugging run
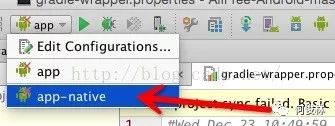
Figure 1
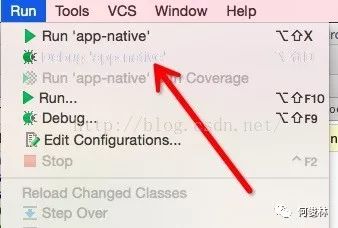
Figure 2
Figures 1 and 2 show two ways to run the app-native; after selecting app-native, debug -> app-native; then you can perform step-by-step debugging in C/C++ files.
Here, I will leave a question: if it is a compiled dynamic library (.so file) with a symbol table, how can it be debugged? Interested readers can leave comments for discussion.