-
Time system function zf_time -
Circular buffer function zf_buffer -
List function zf_list -
State machine function zf_fsm -
Event function zf_event -
Timer function zf_timer -
Task function zf_task
2 Setting Up the Embedded Environment
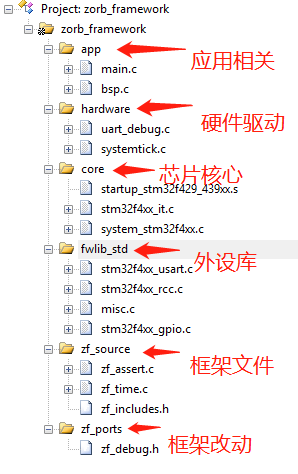
/****************************************************************************** * Description : Hardware environment initialization * Parameters : None * Returns : None******************************************************************************/void BSP_init(void){ /* Select nested vector interrupt controller group */ NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); /* Initialize debug serial port */ Debug_USART_init(); /* SysTick initialization */ SystemTick_init();}/****************************************************************************** * Description : Hardware low-level program * Parameters : None * Returns : None******************************************************************************/void BSP_process(void){}
3 Debug Output
/** ***************************************************************************** * @file zf_debug.h * @author Zorb * @version V1.0.0 * @date 2018-06-28 * @brief Header file for debug output ***************************************************************************** * @history * * 1. Date:2018-06-28 * Author:Zorb * Modification: Created the file * ***************************************************************************** */#ifndef __ZF_DEBUG_H__#define __ZF_DEBUG_H__#ifdef __cplusplusextern "C" {#endif#include "stdio.h"#include "stdbool.h"#define LOG_D 0; /* Information level: Normal */#define LOG_W 1; /* Information level: Warning */#define LOG_E 2; /* Information level: Error */#define _ZF_DEBUG /* Define debug functionality */#define ZF_DEBUG_ON true /* Enable debug functionality */#ifdef _ZF_DEBUG #if ZF_DEBUG_ON #define ZF_DEBUG(rank, x...) do \
{ \
char code[10] = "[rank=0]"; \
code[6] = '0' + (char)rank; \
if (code[6] != '0') \
{ \
printf("%s", code); \
} \
printf(x); \
} while(0) #else #define ZF_DEBUG(rank, x...) #endif /* ZF_DEBUG_ON */#endif /* _ZF_DEBUG */#ifdef __cplusplus}#endif#endif /* __ZF_DEBUG_H__ */ /******************************** END OF FILE ********************************/
4 Implementing Assertions
/** ***************************************************************************** * @file zf_assert.h * @author Zorb * @version V1.0.0 * @date 2018-06-28 * @brief Header file for assertions ***************************************************************************** * @history * * 1. Date:2018-06-28 * Author:Zorb * Modification: Created the file * ***************************************************************************** */#ifndef __ZF_ASSERT_H__#define __ZF_ASSERT_H__#ifdef __cplusplusextern "C" {#endif#include "stdint.h"#define _ZF_ASSERT /* Define assertion functionality */#define ZF_ASSERT_ON true /* Enable assertion functionality */#ifdef _ZF_ASSERT #if ZF_ASSERT_ON #define ZF_ASSERT(expression_) ((expression_) ?\n (void)0 : ZF_assertHandle((uint8_t *)__FILE__, (int)__LINE__)); #else #define ZF_ASSERT(expression_) #endif /* ZF_ASSERT_ON */#endif /* _ZF_ASSERT *//* Handling when an assertion occurs */void ZF_assertHandle(uint8_t *pFileName, int line);#ifdef __cplusplus}#endif#endif /* __ZF_ASSERT_H__ */ /******************************** END OF FILE ********************************/
/** ***************************************************************************** * @file zf_assert.c * @author Zorb * @version V1.0.0 * @date 2018-06-28 * @brief Implementation of assertions ***************************************************************************** * @history * * 1. Date:2018-06-28 * Author:Zorb * Modification: Created the file * ***************************************************************************** */#include "zf_assert.h"#include "zf_debug.h"/****************************************************************************** * Description : Handling when an assertion occurs * Parameters : (in)-pFileName File name * (in)-line Line number * Returns : None******************************************************************************/void ZF_assertHandle(uint8_t *pFileName, int line){ ZF_DEBUG(LOG_E, "file:%s line:%d:asserted\r\n", pFileName, line); while (1);}/******************************** END OF FILE ********************************/
5 Establishing a Time System
/****************************************************************************** * Description : SysTick interrupt service routine * Parameters : None * Returns : None******************************************************************************/void SysTick_Handler(void){ /* Provide timing for zorb framework */ ZF_timeTick();}
/** ***************************************************************************** * @file zf_time.h * @author Zorb * @version V1.0.0 * @date 2018-06-28 * @brief Header file for system time ***************************************************************************** * @history * * 1. Date:2018-06-28 * Author:Zorb * Modification: Created the file * ***************************************************************************** */#ifndef __ZF_TIME_H__#define __ZF_TIME_H__#ifdef __cplusplusextern "C" {#endif#include "stdbool.h"#include "stdint.h"/* System tick period (ms) */#define ZF_TICK_PERIOD 1/* Get system tick count */#define ZF_SYSTICK() ZF_getSystemTick()/* Get system time (ms) */#define ZF_SYSTIME_MS() ZF_getSystemTimeMS()/* System delay (ms) */#define ZF_DELAY_MS(ms_) do \{ \ if (ms_ % ZF_TICK_PERIOD) \ { \ ZF_delayTick((ms_ / ZF_TICK_PERIOD) + 1); \ } \ else \ { \ ZF_delayTick(ms_ / ZF_TICK_PERIOD); \ } \} while(0)/* Get system tick count */uint32_t ZF_getSystemTick(void);/* Get system time (ms) */uint32_t ZF_getSystemTimeMS(void);/* System delay */void ZF_delayTick(uint32_t tick);/* System tick program (needs to be hung in hardware time interrupt) */void ZF_timeTick (void);#ifdef __cplusplus}#endif#endif /* __ZF_TIME_H__ */ /******************************** END OF FILE ********************************/
6 Conclusion
Zorb Framework GitHub:https://github.com/54zorb/Zorb-Framework
[Paid] STM32 Embedded Material Package