Have you tried the built-in touch functionality of the ESP32? Starting from scratch: how to use the touch functionality of the ESP32. In this era full of possibilities, smart hardware development has become increasingly simple. As a “new star” in the hardware development world, the ESP32 not only has powerful Wi-Fi and Bluetooth capabilities but also hides many fascinating skills, such as today’s protagonist: touch sensing functionality. Indeed, this device is not just a chip; it is a “sensing” chip! If you haven’t experienced the touch functionality of the ESP32 yet, don’t worry, today we’ll start from scratch and teach you step by step how to use this feature while exploring its potential.
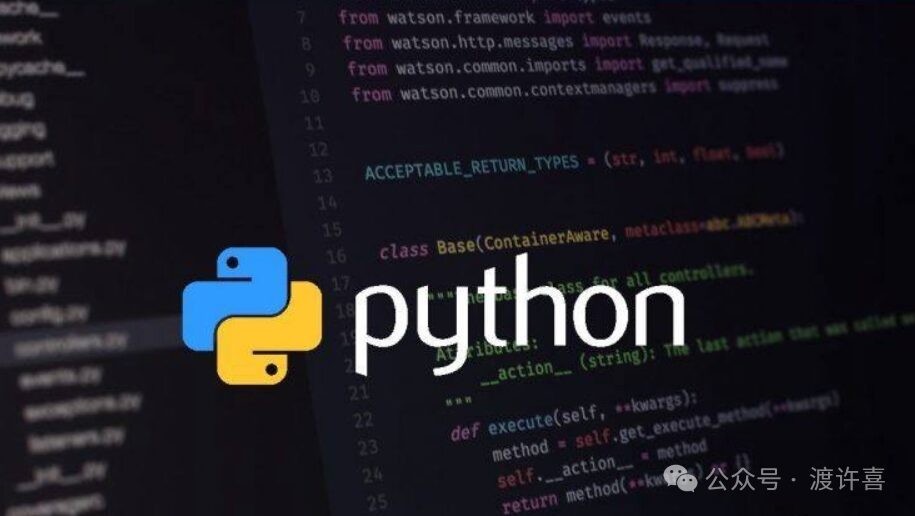
1. What is the touch functionality of the ESP32? The touch functionality of the ESP32 allows the chip to directly sense touch or proximity (Capacitive Sensing). It is based on the principle of capacitance change: when your finger or another conductor approaches the touch pin, the capacitance experiences a slight change, which the chip can detect through its internal touch sensor. The ESP32 has 10 built-in touch pins, which you can use to implement various interesting functions, such as touch light switches, contactless input, or even simple gesture recognition.
Using the touch functionality is particularly simple — no complex external circuits or advanced mathematical models are required; you can achieve it with just a few lines of code.
2. Preparation: Holding an ESP32, is it hard to get started? Before we begin, we need to prepare the following items:
1. An ESP32 development board: any model will do, as long as it has touch functionality (almost all ESP32s do). 2. A USB data cable: to connect the development board to the computer. 3. Arduino IDE or ESP-IDF environment: it is recommended to use Arduino IDE for its simplicity, suitable for beginners. 4. Some wires and touch testing tools: such as aluminum foil (which can serve as a touch pad) or simply using your finger for testing. Ready? Then let’s get started!
3. Quick start with ESP32 touch functionality
-
Find your touch pins. The ESP32 has a total of 10 touch sensing pins, which are:
T0: GPIO4 T1: GPIO0 T2: GPIO2 T3: GPIO15 T4: GPIO13 T5: GPIO12 T6: GPIO14 T7: GPIO27 T8: GPIO33 T9: GPIO32 You can directly implement touch functionality on these pins. Note that different models of development boards may have some pins occupied, such as certain GPIOs being used for onboard buttons or LEDs, so it’s best to check your development board’s pinout diagram.
-
Configure the Arduino IDE environment. If you are using the ESP32 for the first time, make sure your Arduino IDE has the ESP32 board support package installed. If not, follow these steps to install it:
Open the Arduino IDE, go to File > Preferences. In the “Additional Board Manager URLs” field, enter the following URL: https://dl.espressif.com/dl/package_esp32_index.json Then go to Tools > Board > Board Manager, search for ESP32 and install it. Once installed, select the corresponding development board, such as ESP32 Dev Module.
-
Your first touch code. Next, we’ll directly test the touch functionality with a simple code:
#define TOUCH_PIN T0 // Use T0 corresponding to GPIO4
void setup() { Serial.begin(115200); // Initialize serial communication delay(1000); Serial.println(“Starting touch detection…”); }
void loop() { int touchValue = touchRead(TOUCH_PIN); // Read touch value Serial.print(“Touch value: “); Serial.println(touchValue);
// If the touch value is below a certain threshold (e.g., 30), consider it touched if (touchValue < 30) { Serial.println(“Touch detected!”); }
delay(200); // Delay for 200 milliseconds } Let me explain this code:
touchRead(TOUCH_PIN) is the core function used to read the capacitance value of the touch pin. By default, the value when not touched is higher (e.g., 50~100), and when touched, it significantly decreases (e.g., 10~30). We simply judge whether the touch value is below a certain threshold (here it is 30) to determine if a touch has occurred. How to run this code:
Upload the code to the ESP32. Open the serial monitor in the Arduino IDE (set the baud rate to 115200) and check the output. Lightly touch the pin (or the connected touch pad) with your finger and observe the change in touch value. 4. Adjusting touch sensitivity. You may find that the change in touch value is not obvious or has some drift. In this case, you can optimize by:
Changing the threshold: The capacitance change value may vary in different environments, so try adjusting the threshold in if (touchValue < 30), for example, changing it to 20 or 50. Hardware improvements: Connect aluminum foil or metal sheets to the touch pin to increase the contact area. Using filters: Perform simple averaging on the read touch values to reduce noise interference. 4. Advanced play: Unlock more interesting functions
-
Multi-touch detection. The ESP32 supports using multiple touch pins simultaneously, try modifying the above code to detect multiple touch points:
#define TOUCH_PIN1 T0 // GPIO4 #define TOUCH_PIN2 T3 // GPIO15
void setup() { Serial.begin(115200); Serial.println(“Multi-touch detection…”); }
void loop() { int touchValue1 = touchRead(TOUCH_PIN1); int touchValue2 = touchRead(TOUCH_PIN2);
Serial.print(“Touch value 1: “); Serial.println(touchValue1); Serial.print(“Touch value 2: “); Serial.println(touchValue2);
if (touchValue1 < 30) { Serial.println(“Touch point 1 detected!”); } if (touchValue2 < 30) { Serial.println(“Touch point 2 detected!”); }
delay(200); } You can map multiple touch points to different functions, such as lighting different LEDs or playing different sounds.
-
Gesture recognition. If you have multiple touch points, you can also easily implement gesture recognition. For example:
Swipe left: touch T1, T2, T3 in order. Swipe right: touch T3, T2, T1 in order. This requires recording the timing and order of touch events, which can be further optimized into a touch gesture system.
-
Touch light switch. Touch sensing is very suitable for controlling light switches. For example, touch once to turn on the light, and touch again to turn it off:
#define TOUCH_PIN T0 #define LED_PIN 2 // Onboard LED
bool ledState = false;
void setup() { pinMode(LED_PIN, OUTPUT); digitalWrite(LED_PIN, LOW); Serial.begin(115200); }
void loop() { int touchValue = touchRead(TOUCH_PIN);
if (touchValue < 30) { ledState = !ledState; // Toggle LED state digitalWrite(LED_PIN, ledState ? HIGH : LOW); delay(500); // Prevent false touches } } 5. Conclusion. The touch functionality of the ESP32 makes creative development simpler and more interesting. Whether it’s basic touch detection or complex gesture recognition, this small chip can bring you endless possibilities. Moreover, using the touch functionality of the ESP32 generally requires no additional hardware investment; with just a development board and a bit of imagination, you can achieve many interesting projects.
Are you also eager to try this functionality? Quickly grab your ESP32 and start playing! Remember, on the road of smart hardware development, the most important thing is to enjoy the fun of creation. Have fun and happy coding!