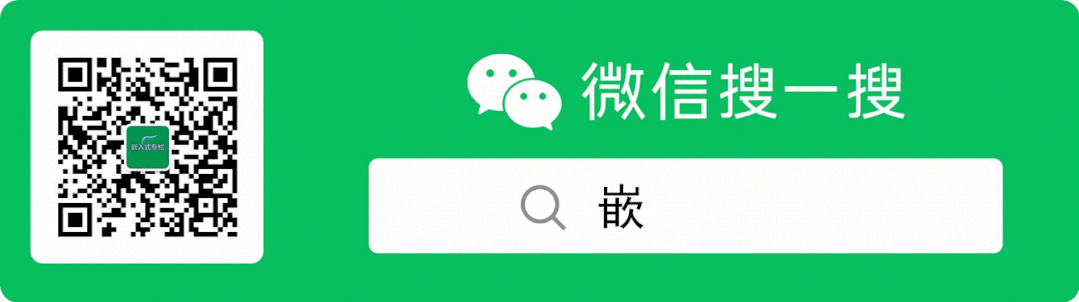
The C language tool code commonly used in embedded development is indeed very important. Below are some sword-level C language tool code examples and their brief explanations.
1. Circular Buffer:
typedef struct {
int buffer[SIZE];
int head;
int tail;
int count;
} CircularBuffer;
void push(CircularBuffer *cb, int data) {
if (cb->count < SIZE) {
cb->buffer[cb->head] = data;
cb->head = (cb->head + 1) % SIZE;
cb->count++;
}
}
int pop(CircularBuffer *cb) {
if (cb->count > 0) {
int data = cb->buffer[cb->tail];
cb->tail = (cb->tail + 1) % SIZE;
cb->count--;
return data;
}
return -1; // Buffer is empty
}
The circular buffer is an efficient data structure suitable for buffering and data stream applications, such as serial communication receive buffers.
2. Assertion:
#define assert(expression) ((void)0)
#ifndef NDEBUG
#undef assert
#define assert(expression) ((expression) ? (void)0 : assert_failed(__FILE__, __LINE__))
#endif
void assert_failed(const char *file, int line) {
printf("Assertion failed at %s:%d\n", file, line);
// Additional error handling or logging can be added here
}
Assertions are used to check whether specific conditions are met in the program, and if the condition is false, it triggers an assertion failure and outputs relevant information.
3. Bit Reversal:
unsigned int reverse_bits(unsigned int num) {
unsigned int numOfBits = sizeof(num) * 8;
unsigned int reverseNum = 0;
for (unsigned int i = 0; i < numOfBits; i++) {
if (num & (1 << i)) {
reverseNum |= (1 << ((numOfBits - 1) - i));
}
}
return reverseNum;
}
This function reverses the bits of a given unsigned integer and can be used for bit-level operations in certain embedded systems.
4. Fixed-Point Arithmetic:
typedef int16_t fixed_t;
#define FIXED_SHIFT 8
#define FLOAT_TO_FIXED(f) ((fixed_t)((f) * (1 << FIXED_SHIFT)))
#define FIXED_TO_FLOAT(f) ((float)(f) / (1 << FIXED_SHIFT))
fixed_t fixed_multiply(fixed_t a, fixed_t b) {
return (fixed_t)(((int32_t)a * (int32_t)b) >> FIXED_SHIFT);
}
In certain embedded systems, floating-point operations can be slow or unsupported. Therefore, using fixed-point arithmetic can provide an efficient floating-point approximation solution.
5. Endianness Conversion:
uint16_t swap_bytes(uint16_t value) { return (value >> 8) | (value << 8); }
A function used to convert between big-endian and little-endian byte orders.
6. Bit Masks:
#define BIT_MASK(bit) (1 << (bit))
A macro used to create a bit mask with only the specified bit set, which can be used for bit manipulation.
7. Timer Counting:
#include
void setup_timer() {
// Configure timer settings
}
uint16_t read_timer() {
return TCNT1;
}
In AVR embedded systems, timers are used to implement time measurement and timing tasks.
8. Binary Search:
int binary_search(int arr[], int size, int target) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Not found
}
A function used to perform binary search in a sorted array.
9. Bitset:
#include
typedef struct {
uint32_t bits;
} Bitset;
void set_bit(Bitset *bitset, int bit) {
bitset->bits |= (1U << bit);
}
int get_bit(Bitset *bitset, int bit) {
return (bitset->bits >> bit) & 1U;
}
A simple bitset data structure implementation for managing the state of a set of bits.
These code examples represent some sword-level C language tool codes commonly used in embedded development. They have wide applications in embedded system development, helping to optimize performance, save resources, and improve code maintainability.
Source address: https://zhuanlan.zhihu.com/p/653484840
———— END ————
● Column “Embedded Tools”
● Column “Embedded Development”
● Column “Keil Tutorial”
● Selected Tutorials from Embedded Column
Follow the public account reply “Join Group” to join the technical exchange group as per the rules, reply “1024” to see more content.
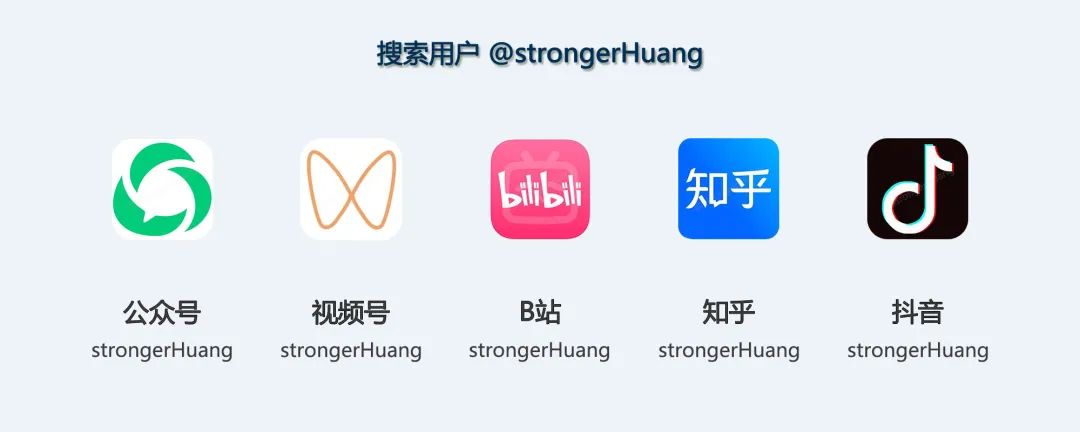
Click “Read Original” to see more shares.