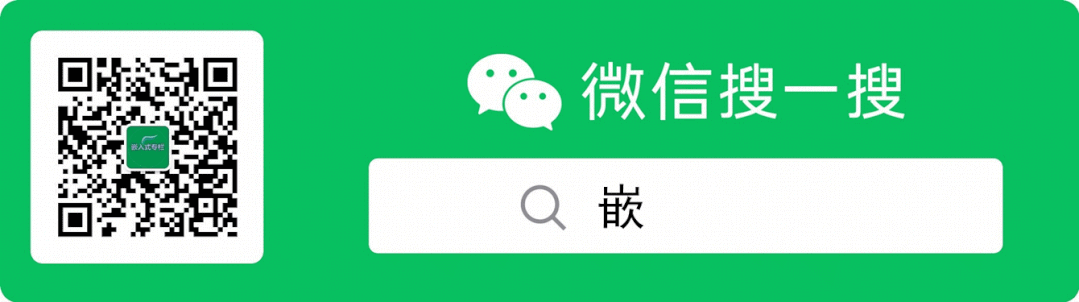
Similarities and Differences Between STM32, Arduino, and Raspberry Pi
1. Hardware Characteristics
Hardware Platform | STM32 | Arduino | Raspberry Pi |
---|---|---|---|
Type | Microcontroller | Open-source Electronic Prototyping Platform | Linux-based Single-board Computer |
Core | ARM Cortex-M Core | Atmel 8-bit Microprocessor | Broadcom ARM Architecture Processor |
Performance | High Performance, Low Power Consumption | Lower Power Consumption, Easy to Start | Higher Computing Power |
Peripheral Interfaces | Rich (e.g., UART, SPI, I2C, etc.) | Rich Interfaces, Easy to Expand | Multiple Interfaces and Communication Protocols |
2. Software and Programming
Hardware Platform | STM32 | Arduino | Raspberry Pi |
---|---|---|---|
Programming Language | C/C++ | Simplified C-like Language (based on Wiring) | Python, C++, Supports Various Linux Distributions |
Development Environment | Various Development Toolchains | Arduino IDE | Various Linux Development Environments, Official Raspberry Pi OS |
Software Ecosystem | Rich Software Libraries and Community Support | Rich Open-source Projects and Tutorials | Extensive Software Support and Community Resources |
3. Application Scenarios
Hardware Platform | STM32 | Arduino | Raspberry Pi |
---|---|---|---|
Applicable Fields | Embedded Systems, Industrial Automation, Wireless Communication, etc. | Education, Maker, Hobbies, Rapid Prototyping | IoT, Multimedia Center, Education, Development Learning, etc. |
Project Examples | Smart Cars, Drones, Smart Homes, etc. | Interactive Art Installations, Automation Control, etc. | IoT Applications, Smart Cars, Facial Recognition, etc. |
4. Usability and Learning Curve
-
STM32: Requires certain basic knowledge of electronics and embedded systems, has a steep learning curve, but is powerful and suitable for complex projects.
-
Arduino: Easy to get started, no complex configuration required, suitable for beginners and rapid prototyping, but has limited functionality and performance.
-
Raspberry Pi: Has high computing power, based on Linux system, easy to learn and use, suitable for various computer projects and IoT applications.
5. Price and Cost
-
STM32: Prices vary depending on model and configuration, but are generally affordable, suitable for mass production.
-
Arduino: Relatively low price, suitable for personal and small projects.
-
Raspberry Pi: Prices vary according to model and configuration, but are relatively low compared to traditional desktop computers.
Lighting Program for STM32, Arduino, and Raspberry Pi
// Assume LED is connected to Pin5 of GPIOA
#define LED_PIN GPIO_PIN_5
#define LED_PORT GPIOA
// GPIO initialization function (generated by STM32CubeMX)
void MX_GPIO_Init(void) {
GPIO_InitTypeDef GPIO_InitStruct = {0};
// Enable clock for GPIOA
__HAL_RCC_GPIOA_CLK_ENABLE();
// Configure GPIOA Pin5 as output mode
GPIO_InitStruct.Pin = LED_PIN;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(LED_PORT, &GPIO_InitStruct);
}
int main(void) {
// HAL library initialization
HAL_Init();
// Configure system clock
SystemClock_Config();
// Initialize all configured peripherals
MX_GPIO_Init();
while (1) {
// Turn on LED
HAL_GPIO_WritePin(LED_PORT, LED_PIN, GPIO_PIN_SET);
// Delay 500 milliseconds
HAL_Delay(500);
// Turn off LED
HAL_GPIO_WritePin(LED_PORT, LED_PIN, GPIO_PIN_RESET);
// Delay 500 milliseconds
HAL_Delay(500);
}
}
// Assume LED is connected to pin 13
const int ledPin = 13;
void setup() {
// Initialize digital pin as an output
pinMode(ledPin, OUTPUT);
}
void loop() {
// Turn on LED
digitalWrite(ledPin, HIGH);
// Delay 500 milliseconds
delay(500);
// Turn off LED
digitalWrite(ledPin, LOW);
// Delay 500 milliseconds
delay(500);
}
import RPi.GPIO as GPIO
import time
# Use BCM GPIO numbering
GPIO.setmode(GPIO.BCM)
# Assume LED is connected to GPIO17
LED_PIN = 17
# Set GPIO pin as output
GPIO.setup(LED_PIN, GPIO.OUT)
try:
while True:
# Turn on LED
GPIO.output(LED_PIN, GPIO.HIGH)
# Delay 500 milliseconds
time.sleep(0.5)
# Turn off LED
GPIO.output(LED_PIN, GPIO.LOW)
# Delay 500 milliseconds
time.sleep(0.5)
except KeyboardInterrupt:
# Catch Ctrl+C to clean up GPIO settings
pass
finally:
# Clean up all GPIO settings
GPIO.cleanup()
