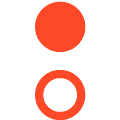
Development and Optimization of Embedded Systems
Hey, C++ friends, today Hui Xiaoqin wants to talk to you about the application of C++ in the Internet of Things (IoT), especially in the development and optimization of embedded systems. The IoT is like a giant network connecting various devices and sensors, and embedded systems are the brains of these devices. Imagine our C++ programs as a savvy commander, orchestrating the collaboration of these devices. Today, we will explore how to develop and optimize embedded systems using C++.
Introduction to IoT and Embedded Systems
The Internet of Things (IoT) is a technology that connects various devices to the internet, enabling them to collect and exchange data. Embedded systems are core components of the IoT; they are computer systems integrated into devices, responsible for controlling and managing the functionalities of the devices.
Advantages of C++ in Embedded Systems
C++ has unique advantages in embedded system development due to its efficiency, flexibility, and portability. It provides near-hardware control capabilities while supporting advanced features like object-oriented programming and templates.
Memory Management
In embedded systems, memory resources are often very limited. C++ offers various memory management techniques, such as manual memory allocation and smart pointers, to help us use memory efficiently.
#include <memory>
class Sensor {
public:
void readData() {
// Read sensor data
}
};
int main() {
// Use std::unique_ptr to manage Sensor object
std::unique_ptr<Sensor> sensor(new Sensor());
sensor->readData();
return 0;
}
This code demonstrates how to use <span>std::unique_ptr</span>
to manage resources in embedded systems, ensuring that memory is automatically released when the object is no longer in use.
Real-Time Performance
Embedded systems often need to meet real-time performance requirements. C++ provides various tools and techniques, such as priority scheduling and interrupt handling, to help us achieve efficient real-time control.
#include <iostream>
#include <chrono>
void timerCallback() {
std::cout << "Timer callback triggered" << std::endl;
// Execute timed task
}
int main() {
// Set up timer
std::chrono::seconds interval(1); // 1 second interval
// Start timer
// ...
return 0;
}
This code demonstrates how to set up a timer and execute a callback function when the timer is triggered.
Real-World Applications
Smart Home
In smart homes, embedded systems can be used to control smart devices such as smart bulbs, smart plugs, and smart locks. C++ can help us achieve communication and collaboration between devices.
Industrial Automation
In industrial automation, embedded systems can be used to control various devices and sensors on production lines. C++ can help us achieve efficient real-time control and data processing.
Exercise
Hui Mei has a small exercise for everyone: try to write a simple embedded system program in C++ to control the blinking of an LED light.
Conclusion
The combination of C++ and the IoT provides strong support for developing and optimizing embedded systems. Through today’s learning, you should have a deeper understanding of the application of C++ in embedded systems. Remember to practice coding, and feel free to ask questions in the comments section. I wish everyone a pleasant learning experience and continuous improvement in C++!
Friends, today’s journey in learning C++ ends here! Remember to practice coding, and feel free to ask questions in the comments section. I wish everyone a pleasant learning experience and continuous improvement in C++!