Hello everyone! I am Cat Brother, and today I will guide you to create a super cool project: the ESP32 E-Paper Weather Display! Are you excited? Don’t worry, even if you know nothing about Python and hardware, just follow me step by step, and I guarantee you can handle it easily!
Introduction: Light Up Your E-Paper
The little gadget we are going to make today can display weather information in real-time, and it has very low power consumption, making it perfect for placing on your bedside table or desk. The core components used are the ESP32 development board and an e-paper display. Don’t worry about these terms; I will explain them slowly later. Just think about it: every morning when you wake up, you can see the latest weather forecast without opening your phone. Isn’t that convenient?
Part One: Preparation
We need to prepare some materials:
-
One ESP32 development board
-
One e-paper display (recommended size: 2.9 inches)
-
Several connecting wires
-
One MicroUSB data cable
On the software side, we need to install:
-
Thonny IDE (recommended, beginner-friendly) or other Python IDEs
-
MicroPython firmware (needs to be flashed onto the ESP32)
Tip: Choosing the right e-paper display driver library is very important and can save you a lot of trouble!
Part Two: Flashing MicroPython Firmware
This step is like installing an operating system on the ESP32. After downloading the MicroPython firmware, use Thonny IDE or tools like esptool to flash it onto the ESP32 development board. You can refer to the official documentation for ESP32 and Thonny for specific operations.
Tip: During the flashing process, ensure that the ESP32 is correctly connected to the computer and that you have selected the correct serial port.
Part Three: Writing Python Code
The exciting moment has arrived! We are going to start writing code! Don’t be afraid; I will break down the code into small pieces for everyone to understand.
import network
import urequests
import json
from epd import EPD_2in9 # Import the corresponding library based on your e-paper display model
# Connect to Wi-Fi
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
wlan.connect("Your Wi-Fi Name", "Your Wi-Fi Password")
while not wlan.isconnected():
pass
# Get weather data
api_key = "Your Weather API Key" # You need to apply for a weather API key
city = "Your City"
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=metric" # Use metric units
response = urequests.get(url)
weather_data = json.loads(response.text)
# Parse weather data
temperature = weather_data["main"]["temp"]
description = weather_data["weather"][0]["description"]
# Display on e-paper screen
epd = EPD_2in9()
epd.init()
epd.draw_string_at(10, 10, f"Temperature: {temperature}°C", 15) # Display temperature
epd.draw_string_at(10, 30, f"Weather: {description}", 15) # Display weather description
epd.display()
# Deep sleep, save power!
epd.sleep()
Code Explanation:
-
We connect to the Wi-Fi network.
-
We use the weather API to get weather data; here you need to replace it with your own API key and city.
-
Next, we parse the obtained JSON-formatted weather data to extract the temperature and weather description.
-
We use the e-paper display library to show the weather information on the screen.
-
epd.sleep()
puts the ESP32 into deep sleep mode to save power as much as possible.
Note: Remember to install the necessary libraries, such as urequests
and your e-paper display library.
Part Four: Running the Program
Save the code onto the ESP32 and then run it. The moment of witnessing the miracle has arrived! Your e-paper weather display should start working!
Conclusion
Friends, today’s Python learning journey ends here! Remember to get hands-on coding, and feel free to ask Cat Brother in the comments if you have any questions. I wish everyone a happy learning experience, and may your Python skills improve day by day!
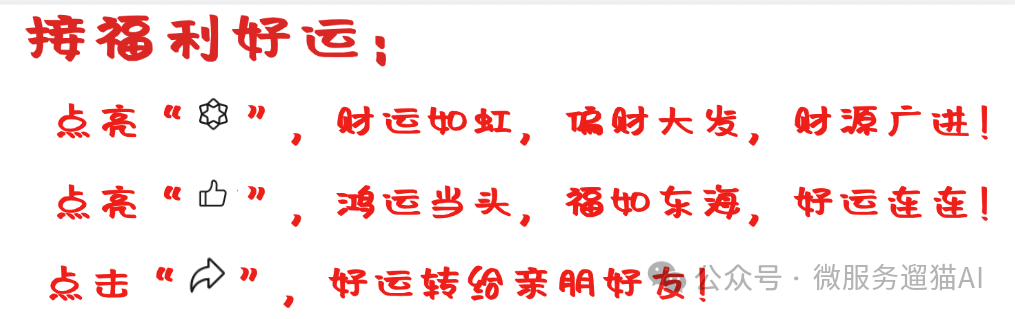