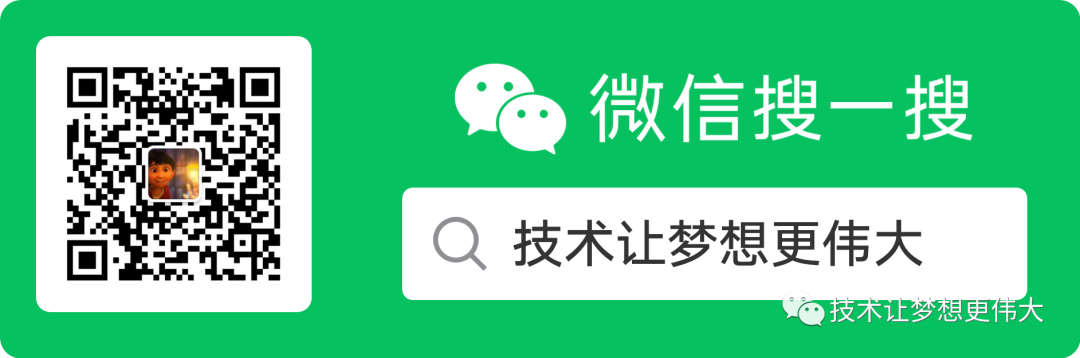
ID: Embedded Cloud IoT Technology Circle
Author: Yang Yuanxin
Portal:【Architecture】How to Layer the Structure of Code in Embedded Programming
No more nonsense, talking too much theory doesn’t feel right. These rules are essentially based on some theories related to object-oriented design patterns. Design patterns are a set of useful frameworks summarized from the practices of predecessors. So let’s get straight to the point and get to the useful stuff! Taking the SPI OLED driver on the Bear Pi as an example, we will make some simple modifications to the LCD driver in the original development package. Then, based on our needs, we design the following driver model framework, divided into three parts: model, driver, and device. We won’t consider too many details at first, nor will we make this thing too complicated at the beginning, as that would hinder understanding. Thus, we construct the following framework mind map:
1. LCD Driver Framework Data Structure
What capabilities does the framework provide? Here’s how I did it, very simple:
This provides the capability to interface the driver framework with the driver. The lcd_driver
is a structure that has been defined and assigned in the driver file. Thus, when I define a variable of LCD_Driver_Model
elsewhere, I can interface this variable with the driver structure, allowing me to operate the interfaces in the driver structure through this variable.
2. LCD Driver Data Structure
The function of the LCD driver data structure is to provide the interface for operating the LCD driver, and this interface design is independent of the hardware.
As mentioned in the previous section, the driver framework relies on the driver interface. Therefore, we need to implement the methods in the driver interface. In the corresponding methods, we will call the interfaces related to the LCD device to operate the LCD device. The following are the implementations corresponding to the interfaces:
3. LCD Device Data Structure
The LCD device needs to connect the functional functions in this data structure with the actual LCD driver interfaces. For example, let’s look at the implementation of the LCD_Init
interface, which actually calls the real hardware operations of the LCD:
4. Usage Method
int main(void)
{
/* USER CODE BEGIN 1 */
LCD_Driver_Model lcd_model ;
LCD_Ascii_Show_Para ascii_para[] =
{
{80, 100, 240-80, "RED", BLACK, RED, 32},
{80, 100, 240-80, "GREEN", BLACK, GREEN, 32},
{80, 100, 240-80, "BLUE", BLACK, BLUE, 32},
};
LCD_Fill_Para fill_para[] =
{
{ascii_para[0].x,ascii_para[0].max_width,ascii_para[0].y,ascii_para[0].y+32},
{ascii_para[1].x,ascii_para[1].max_width,ascii_para[1].y,ascii_para[1].y+32},
{ascii_para[2].x,ascii_para[2].max_width,ascii_para[2].y,ascii_para[2].y+32},
} ;
/* USER CODE END 1 */
/* MCU Configuration--------------------------------------------------------*/
/* Reset of all peripherals, Initializes the Flash interface and the Systick. */
HAL_Init();
/* USER CODE BEGIN Init */
/* USER CODE END Init */
/* Configure the system clock */
SystemClock_Config();
/* USER CODE BEGIN SysInit */
/* USER CODE END SysInit */
/* Initialize all configured peripherals */
MX_GPIO_Init();
MX_I2C1_Init();
MX_USART1_UART_Init();
MX_SPI2_Init();
/* USER CODE BEGIN 2 */
/* Add this delay after serial port initialization to prevent garbled printf prints later */
HAL_Delay(200);
/* Register driver model */
Register_Driver_Model(&lcd_model);
/* Call LCD initialization */
lcd_model.lcd_driver->lcd_init();
/* Call LCD to display ASCII string */
lcd_model.lcd_driver->lcd_show_ascii_str(ascii_para[0]);
/* USER CODE END 2 */
/* Infinite loop */
/* USER CODE BEGIN WHILE */
while (1)
{
/* USER CODE END WHILE */
/* USER CODE BEGIN 3 */
/* Loop to call LCD to display ASCII string */
for(int i = 0 ; i < 3 ; i++)
{
lcd_model.lcd_driver->lcd_fill(fill_para[i]);
lcd_model.lcd_driver->lcd_show_ascii_str(ascii_para[i]);
HAL_Delay(100);
}
}
/* USER CODE END 3 */
}
Thus, we have completed the simplest layered design of the LCD driver. Of course, our software framework still needs to be continuously improved to make it more robust. This way, whenever we have a new LCD, we can design a similar fixed template according to the actual business needs, defining suitable interfaces for design. In the future, whenever we switch to another project using the same LCD, it will be very simple!
5. Reflection
Earlier, I open-sourced a gas detector project based on TencentOS tiny
. Can you continue to optimize and improve that project?
The code for this section has been synchronized to the code repository on Gitee. Here’s how to obtain it:
1. Create a new folder
2. Use git clone to remotely obtain the code repository where the Bear Pi example is stored
Project open-source repository:
https://gitee.com/morixinguan/bear-pi.git

Embedded Programming Collection
Linux Learning Collection
C/C++ Programming Collection
Qt Advanced Learning Collection
Follow the WeChat public account "Technology Makes Dreams Greater", reply "m" to see more content; scan the WeChat below, add the author's WeChat to join the technical exchange group, please introduce yourself first.