Introduction
This task sheet is part of the new course content for “2.4 Sensing and Control” and serves as a prerequisite for the “Indoor Environment Real-Time Monitoring System” project. It aims to let students experience the process of hardware programming with micro:bit through several simple practical tasks over two class sessions, allowing them to initially grasp the basic methods of using a computer to write programs to control micro:bit and other smart terminals, thereby laying a solid foundation for the subsequent “Indoor Environment Real-Time Monitoring System” project.
The task sheet includes a total of 4 cases with 8 sub-tasks. Teachers can provide different levels of semi-finished code based on students’ actual needs, combining teaching and practice to strive for the integration of core subject competencies and the learning of knowledge and skills in project practice.
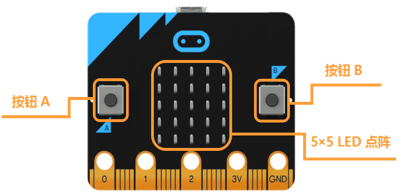
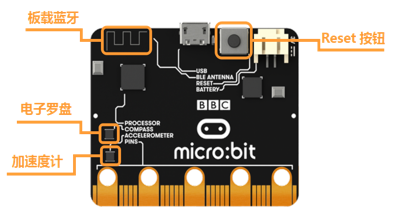
Micro:bit Practical Operation Task Sheet:
Task 1. Scrolling Text Display on Dot Matrix Screen.
1, Connect the micro:bit to the computer using a USB cable and confirm the connection is correct.
2, Open the BXY programming environment, go to the file menu – example – Basic – “01.hello.py”, as shown in the figure below:
Observe the code, then upload it to the micro:bit board and observe the result. (Press the reset button to restart the program)
from microbit import display
display.scroll("hello world!") # Scroll text
3, Modify the code to implement text scrolling display in two different ways.
Note: After modifying the code, please save it as a different file name (e.g., 1.1.py).
display.scroll("hello world!", loop=True)
while True:
display.scroll("hello world!")
(3) You can add other functional statements based on (2): such as displaying various built-in images.
while True:
display.scroll("hello world!") # Scroll text
display.show(Image.HAPPY) # Show happy face
sleep(2000)
Note: First check for syntax errors. If no errors, save and upload.
Task2: Use Onboard Temperature Sensor to Acquire Environmental Data
1, Create a new file, write code to acquire environmental data using the onboard temperature sensor, and scroll the current temperature on the dot matrix screen.
First check for syntax errors, then upload to the micro:bit board and observe the result.
from microbit import *
while True:
display.scroll(str(temperature()))
sleep(2000)
2, Try adding code to view serial data using a serial debugging tool.
print(temperature())
sleep(2000)
3, Cover the micro:bit board with your hand and breathe on it to observe the changes in serial data.
Task3:Smart Terminal Responds Differently Based on Environmental Temperature
1, Create a new file, write code to acquire environmental temperature using the onboard temperature sensor, display the current temperature using a serial tool. At the same time, when the temperature is below 20 degrees, the LED array displays “OK”, otherwise it displays “NO”.
The reference code is as follows. Check for syntax errors, save, and then upload to the micro:bit board and observe the result.
from microbit import *
while True:
print(temperature())
if temperature() < 20:
display.scroll("ok")
else:
display.scroll("no")
sleep(2000)
2, Try modifying the code to display a “happy face” on the LED array when the temperature is below 26 degrees, otherwise display a “sad face”. The reference code is as follows:
Task4:Use LM35 Analog Temperature Sensor to Acquire Environmental Data.
1, Connect the micro:bit board to the expansion board, and connect the LM35 analog temperature sensor to the pin0 port of the expansion board.
2, Connect the micro:bit to the computer using a USB cable and confirm the connection is correct.
3, Open the BXY programming environment, create a new file, write code to acquire environmental data using the external temperature sensor, scroll the current temperature on the dot matrix screen, and display the current temperature using a serial tool.
from microbit import *
while True:
val = pin0.read_analog() # Get the temperature analog value
temp = round(val / 1024 * 3000 / 10.24, 1) # Convert to actual temperature and keep one decimal
display.scroll(temp) # Scroll the actual temperature on the LED matrix
print(temp) # Output the actual temperature in the serial monitor
sleep(2000) # Get temperature data every 2 seconds
4, Cover the LM35 analog temperature sensor with your hand and breathe on it to observe the changes in serial data.
Task5:Use Python Programming to Acquire Serial Data.
1, Connect the hardware according to Task 4 requirements, write the hardware program, and upload it to the micro:bit board.
2, Write the following program in IDLE:
import serial
ser = serial.Serial()
ser.baudrate = 115200 # Set the communication baud rate, which needs to match the rate set in micro:bit
ser.port = 'COM3' # Serial port number should be based on the actual value
ser.open() # Output serial data
while True:
print(ser.readline())
Note: Before running the Python program, close the BXY editor.
3, Save the data acquired from the serial port to a text file. Replace the code that outputs the serial data with the following code:
# Save the data acquired from the serial port to a text file
f = open('temp.txt', 'wb')
n = 10
while n > 0:
n -= 1
line = ser.readline()
f.write(line)
print(line)
f.close()
ser.close()
In addition to the necessary hardware, this project also requires downloading the BXY_Python_Editor software and installing the pyserial module in Python. To facilitate students’ experience and save class time, teachers can pre-install the relevant modules and send the source code to students, allowing them to run the program directly.
This task sheet consists of four cases that gradually increase in difficulty. Teachers can first demonstrate and introduce related knowledge points, then give students enough time to run the program, experience the complete process of hardware programming, and simply modify the program functions according to their needs.
If you need the Word document, source code, and answers to the post-class reflection for this article, you can join the “Python Algorithm Journey” knowledge community to participate in discussions and download files. The “Python Algorithm Journey” knowledge community gathers numerous like-minded individuals, where more interesting topics are discussed and more useful materials are shared.
We focus on Python algorithms, and if you’re interested, join us!
Related Excellent Articles:
Reading Code and Writing Better Code
The Most Effective Learning Method
Python Algorithm Journey Article Categories
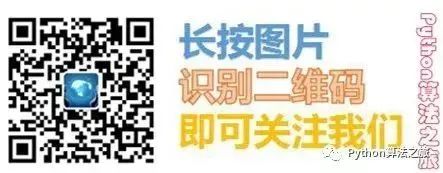