Click the “blue words” to follow us
I was just lying on the office couch, chewing on a nearly cold pancake, when suddenly I received a WeChat message: “Bro, help! The online service is down again, this is the third time! Are our failure drills really useful?”
Seeing this message, I couldn’t help but smile. As a veteran of the internet for 15 years, I fully understand this anxiety. Today, let’s talk about how to use Cursor, this AI tool, to tackle chaos engineering and fault injection.
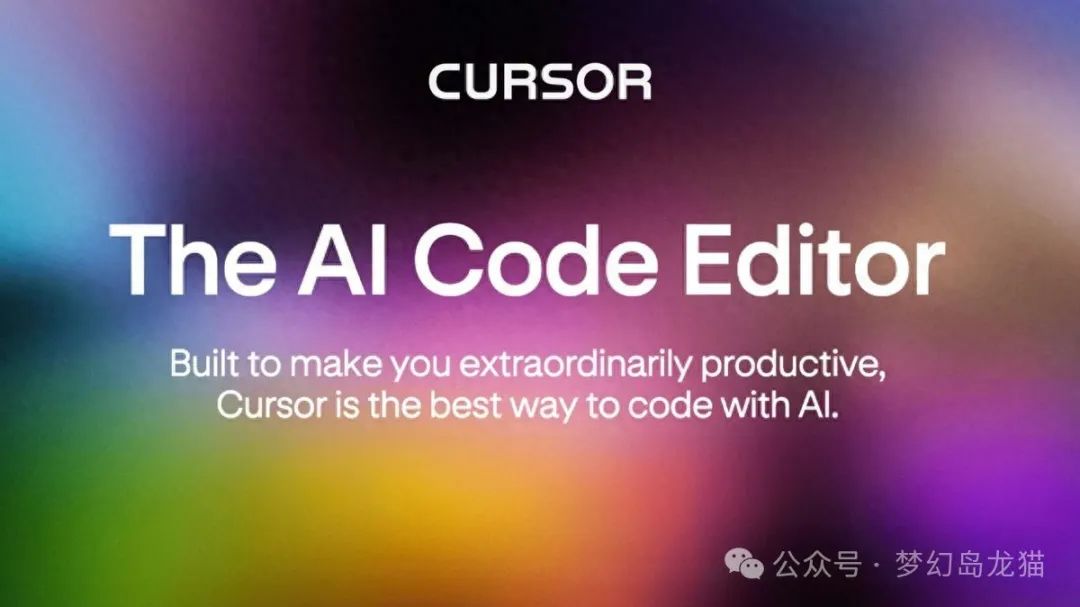
To be honest, when I first saw Cursor, I thought it was just an ordinary code editor, but it completely blew my mind.
This tool not only writes code but also helps you design test cases, find bugs, and optimize performance. It is simply a reliable engineer’s best friend.
Let’s look at a simple example:
# Fault injection test generated by Cursor
def inject_latency(service_call):
@wraps(service_call)
def wrapper(*args, **kwargs):
if random.random() < 0.1: # 10% chance to trigger delay
time.sleep(random.uniform(1, 3))
return service_call(*args, **kwargs)
return wrapper
@inject_latency
def process_order(order_id):
# Business logic for processing order
pass
This code hides an interesting mechanism that randomly adds some “spice” to your service, allowing you to discover potential performance issues in advance.
🎯 Chaos Engineering Is Not Just Random Messing Around
A recent graduate asked me: “Bro, why do we need to create trouble for the system?”
I told him a joke: Is the barbell in the gym making things hard for you? No, it is making you stronger! Chaos engineering is similar; through planned fault injection, it makes the system more robust.
# Chaos experiment assisted by Cursor
class ChaosExperiment:
def __init__(self, target_service):
self.service = target_service
self.experiments = []
def add_failure(self, failure_type, probability):
self.experiments.append({
'type': failure_type,
'probability': probability
})
def run(self):
for exp in self.experiments:
if random.random() < exp['probability']:
self._inject_failure(exp['type'])
Friendly reminder: Don’t just rush into production; always test in a staging environment first!
💡 AI Assistance Makes It Twice as Effective
Honestly, writing test cases with Cursor is incredibly satisfying. In the past, writing a test case would take forever; now? I just tell it: “Help me write a test that simulates a database timeout scenario,” and it generates it in no time.
# Database fault test generated by Cursor
def test_database_timeout():
with patch('database.connect') as mock_db:
mock_db.side_effect = TimeoutError("Connection timed out")
response = service.process_request()
assert response.status_code == 503
assert "Service Temporarily Unavailable" in response.body
Don’t just talk without practice; let’s do a hands-on exercise. Suppose you want to test the fault recovery capability of an order system:
# Fault recovery test designed with Cursor
class OrderSystemResilience:
def __init__(self):
self.chaos = ChaosExperiment(OrderService())
def test_resilience(self):
self.chaos.add_failure('network_delay', 0.2)
self.chaos.add_failure('db_connection_loss', 0.1)
success_rate = self._run_benchmark(1000) # Run 1000 tests
print(f"System recovery score: {success_rate}%")
Seeing this code reminds me of a young man who asked me a couple of days ago: “Bro, why do our tests always fail to find issues?”
Brother, testing is not just a game; it requires strategy. You need to consider: network delays, service timeouts, database connection drops, memory saturation, disk IO exceptions… these are all real pitfalls.
Having written code for so many years, I’ve learned a truth: system stability is improved by digging and filling pits. That’s all for today; I need to deal with an online issue now. If you have any questions, just @ me!
Remember: No matter how good the tool is, you have to know how to use it. Practice more and think critically; don’t just copy and paste. Coding is not a feast; it requires brain work!
Tap “View” to recommend it to others