“Starred Public Account” Let’s progress together!
Source: https://gitee.com/MacRsh/mr-library
Introduction to mr-library
The MR
framework is a lightweight framework designed specifically for embedded systems. It fully considers the resource and performance needs of embedded systems. By providing standardized device management interfaces, it greatly simplifies the difficulty of embedded application development, helping developers quickly build embedded applications.
The framework provides developers with standardized interfaces for opening (open
), closing (close
), controlling (ioctl
), reading (read
), and writing (write
). It decouples the application from the underlying hardware drivers. Applications do not need to understand the implementation details of the drivers.
When the hardware changes, only the underlying driver needs to be adapted, and the application can seamlessly migrate to the new hardware. This greatly improves the reusability of the software and the scalability to new hardware.
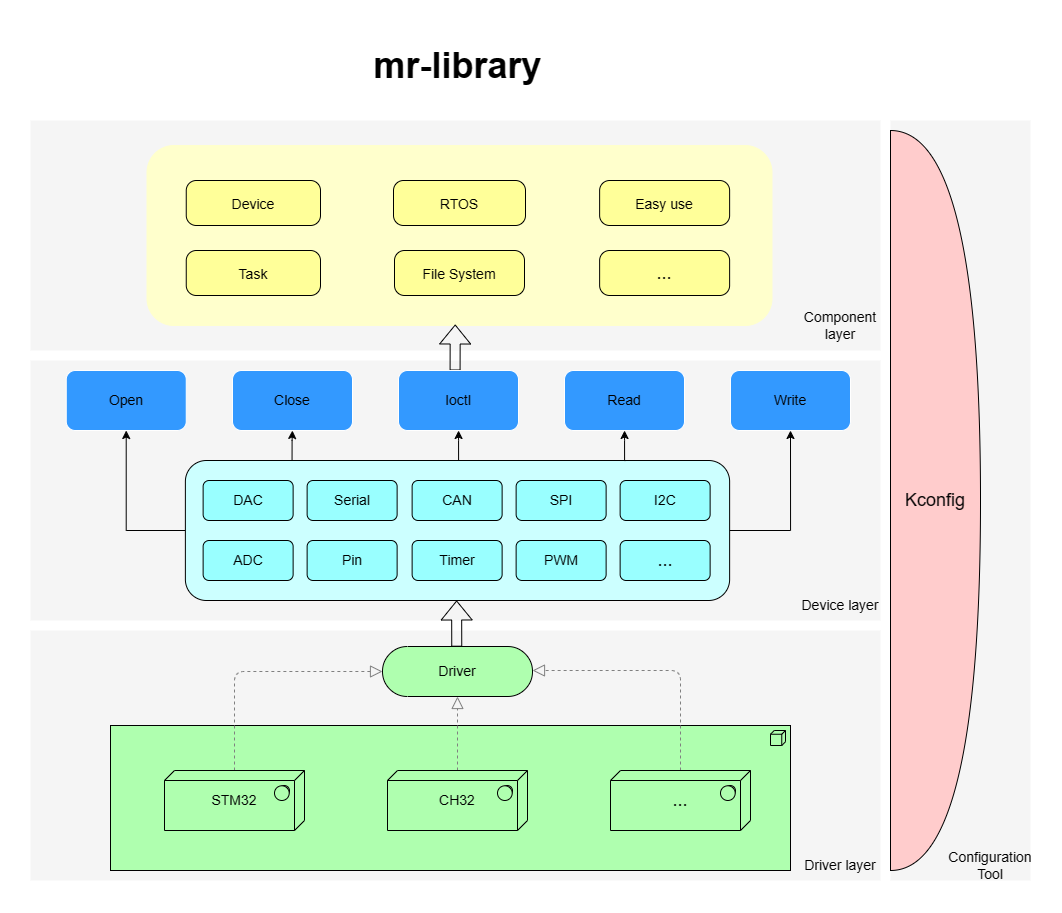
Key Features
-
Standardized device access interfaces -
Decoupling of application and driver development -
Simplified development of low-level drivers and applications -
Lightweight and easy to use, low resource consumption -
Modular design, decoupled and independently developed parts, extremely low hardware migration costs -
Supports use in bare-metal and operating system environments
Main Components
-
Device Framework: Provides standard interfaces for device access -
Memory Management: Dynamic memory management -
Utilities: Common data structures such as linked lists, queues, balanced trees, etc. -
Various functional components
Standardized Device Interfaces
All operations on devices can be accomplished through the following interfaces:
Interface | Description |
---|---|
mr_dev_register | Register device |
mr_dev_open | Open device |
mr_dev_close | Close device |
mr_dev_ioctl | Control device |
mr_dev_read | Read data from device |
mr_dev_write | Write data to device |
Example:
struct mr_spi_dev spi_dev;
int main(void)
{
/* Register SPI10 device (CS active low) to SPI1 bus */
mr_spi_dev_register(&spi_dev, "spi1/spi10", 0, MR_SPI_CS_ACTIVE_LOW);
/* Open SPI10 device on SPI1 bus */
int ds = mr_dev_open("spi1/spi10", MR_OFLAG_RDWR);
/* Send data */
uint8_t wr_buf[] = {0x01, 0x02, 0x03, 0x04};
mr_dev_write(ds, wr_buf, sizeof(wr_buf));
/* Receive data */
uint8_t rd_buf[4] = {0};
mr_dev_read(ds, rd_buf, sizeof(rd_buf));
/* Close device */
mr_dev_close(ds);
}
Configuration Tool
The MR
framework provides a Kconfig
visual configuration tool, allowing developers to configure without deep understanding of the source code.
Kconfig
will automatically generate a configuration options interface based on the configuration file. Developers can easily select the required functional components and set related parameters through simple operations.
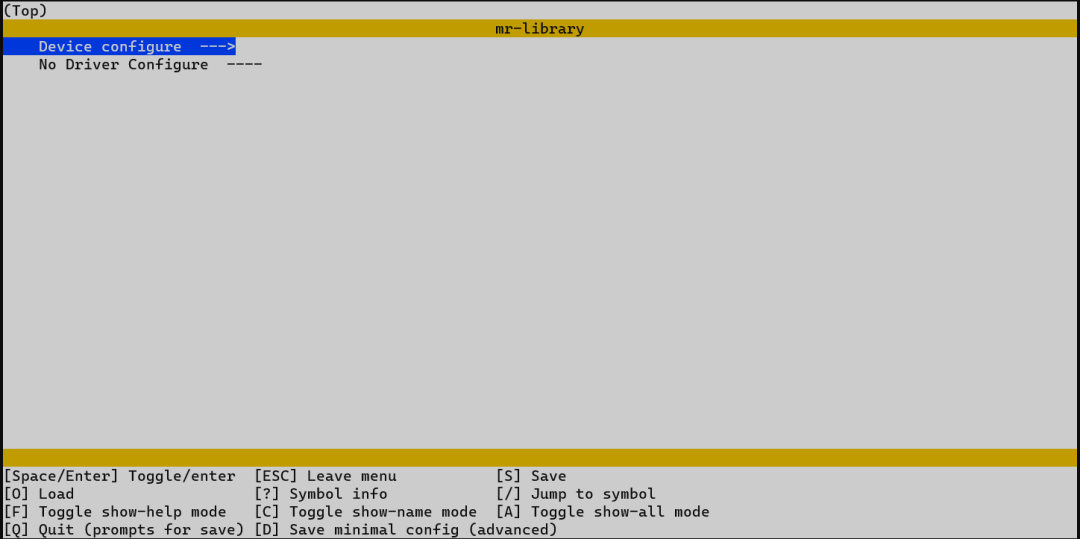
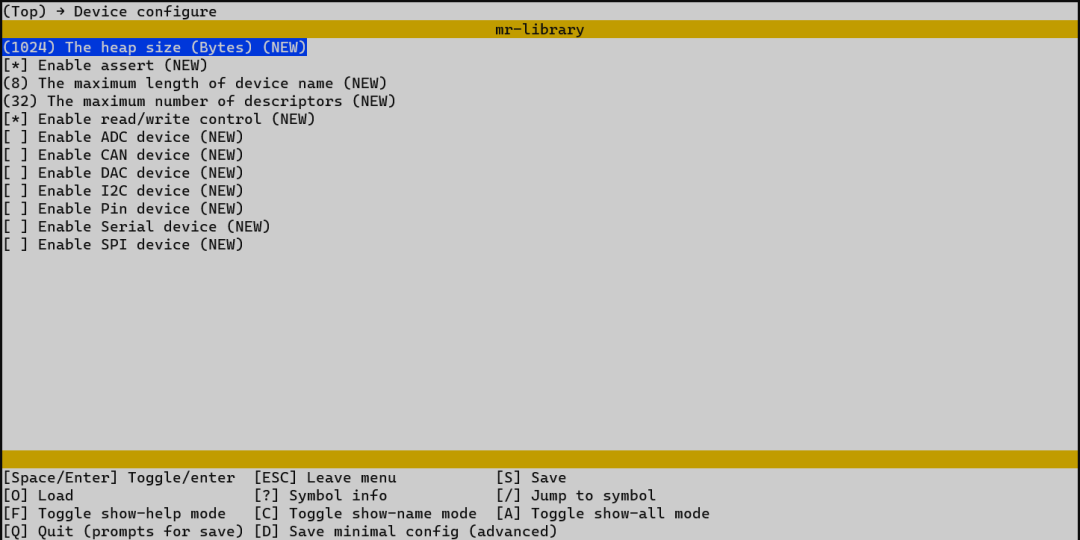
By modifying parameters, quickly trim the required functions. After configuration is complete, a Python
script automatically generates the configuration file.
Directory Structure
Name | Description |
---|---|
bsp | Board Support Package |
components | Components |
device | Device Files |
document | Documentation |
driver | Driver Files |
include | Library Header Files |
source | Library Source Files |
Kconfig | Configuration Files |
kconfig.py | Automatic Configuration Script |
LICENSE | License |
Getting Started
Configuring the Kconfig
Environment
Note: Kconfig
is not mandatory, but recommended (installation and configuration are very quick, and subsequent tutorials will also take Kconfig
as an example).
-
Verify that Python is installed on the system. Run
python --version
in the command line to check the Python version (Kconfig
depends onpython
, ifpython
is not installed, please install it yourself). -
Use the command shown to install
Kconfig
in the command line:python -m pip install windows-curses python -m pip install kconfiglib
-
Run
menuconfig -h
in the command line to verify if the installation was successful.
Importing the Framework into the Project
-
Download the latest version of the source code from the
Gitee
orGithub
repository to your local machine. -
Import the source code into the directory where your project is located. For example, in an STM32 project:
Project Directory -
If the chip you are using has already been adapted with
BSP
, please refer to the configuration tutorial in the correspondingBSP
to complete theBSP
configuration. -
Remove unnecessary files in the
bsp
,document
, andmodule
directories (ifGIT
is not needed, you can also remove the.git
files). After completion, the directory structure is as follows:Project Directory 1 -
Add files to the IDE (most IDEs can automatically recognize files under the project path, this step is not necessary). For example, in
keil
:Project Directory Keil Add all files from the
source
,device
, anddriver
directories.
Configuring Menu Options
-
Open the command line tool in the
mr-library
directory, runmenuconfig
for menu configuration.Project Directory 2 Note: When adding the corresponding chip driver,
Device configure
andDriver configure
will be displayed. Please refer to the tutorial inBSP
for correspondingDriver configure
. -
Select
Device configure
and press enter to enter the menu, configure functions as needed.Project Directory 3 -
After configuration is complete, press
Q
to exit the menu configuration interface, pressY
to save the configuration.
Generating Configuration Files
-
Open the command line tool in the mr-library
directory, runpython kconfig.py
, and automatically generate the configuration filemr_config.h
.
Adding Include Paths
-
Add the include path of
mr-library
in the compiler, for example inkeil
:Project Directory 4 -
Configure automatic initialization (GCC environment), look for the linker script file with the suffix
.ld
in your project (usuallylink.ld
), and add the following code in the script file: Note: If you are in an environment likekeil
that can automatically generate linker scripts, please skip this step./* mr-library auto init */ . = ALIGN(4); _mr_auto_init_start = .; KEEP(*(SORT(.auto_init*))) _mr_auto_init_end = .;
Example:
Project Directory 5 -
Configure GNU syntax. If you are using a non-
GCC
compiler, please enable GNU syntax. For example inkeil
:AC5:
Project Directory 6 AC6:
Project Directory 7 -
Include
#include "include/mr_lib.h"
in your project. -
Add
mr_auto_init();
automatic initialization function in themain
function.
Let’s Light Up
#include "include/mr_lib.h"
/* Define LED pin (PC13)*/
#define LED_PIN_NUMBER 45
int main(void)
{
/* Automatic initialization */
mr_auto_init();
/* Open PIN device */
int ds = mr_dev_open("pin", MR_OFLAG_RDWR);
/* Set to LED pin */
mr_dev_ioctl(ds, MR_CTL_PIN_SET_NUMBER, mr_make_local(int, LED_PIN_NUMBER));
/* Set LED pin to push-pull output mode */
mr_dev_ioctl(ds, MR_CTL_PIN_SET_MODE, mr_make_local(int, MR_PIN_MODE_OUTPUT));
while(1)
{
/* Light up LED */
mr_dev_write(ds, mr_make_local(uint8_t, MR_PIN_HIGH_LEVEL), sizeof(uint8_t));
mr_delay_ms(500);
mr_dev_write(ds, mr_make_local(uint8_t, MR_PIN_LOW_LEVEL), sizeof(uint8_t));
mr_delay_ms(500);
}
}
Hello World
#include "include/mr_lib.h"
int main(void)
{
/* Automatic initialization */
mr_auto_init();
/* Open Serial-1 device */
int ds = mr_dev_open("serial1", MR_OFLAG_RDWR);
/* Output Hello World */
mr_dev_write(ds, "Hello World\r\n", sizeof("Hello World\r\n"));
while(1);
}
This article is sourced from the internet, free to convey knowledge, and copyright belongs to the original author. If there are copyright issues regarding the work, please contact me for deletion.
Previous Recommendations
Application of Flexible Arrays in Practical Projects?
“Comprehensive Guide to Embedded Linux Drivers”
Leave a Comment
Your email address will not be published. Required fields are marked *