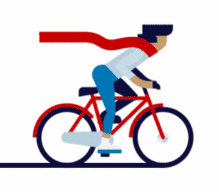
Click the blue text above to follow us
This article uses the ESP32-S3 to set up a network camera. Compared to a local area network camera, this article will share how to set up a network camera that can be accessed from the external network.
This mainly uses the technology of network penetration, which is to ensure that data packets with a specific source IP address and source port number (here referring to the local area network camera) are correctly routed to the internal network host without being blocked by the network address translation device.
The main process is divided into two steps:
1. First, realize access to the network camera in the local area network;
2. On this basis, use the method of network penetration to set up a network camera that can be accessed from the external network.
1
Local Area Network Camera
The method to achieve a local area network camera with ESP32 is relatively simple, the driver code is as follows:
#include "esp_camera.h"
#include
//// WARNING!!! PSRAM IC required for UXGA resolution and high JPEG quality// Ensure ESP32 Wrover Module or other board with PSRAM is selected// Partial images will be transmitted if image exceeds buffer size//// You must select partition scheme from the board menu that has at least 3MB APP space.// Face Recognition is DISABLED for ESP32 and ESP32-S2, because it takes up from 15 // seconds to process single frame. Face Detection is ENABLED if PSRAM is enabled as well
// ===================// Select camera model// ===================//#define CAMERA_MODEL_WROVER_KIT // Has PSRAM// #define CAMERA_MODEL_ESP_EYE // Has PSRAM//#define CAMERA_MODEL_ESP32S3_EYE // Has PSRAM//#define CAMERA_MODEL_M5STACK_PSRAM // Has PSRAM//#define CAMERA_MODEL_M5STACK_V2_PSRAM // M5Camera version B Has PSRAM//#define CAMERA_MODEL_M5STACK_WIDE // Has PSRAM//#define CAMERA_MODEL_M5STACK_ESP32CAM // No PSRAM//#define CAMERA_MODEL_M5STACK_UNITCAM // No PSRAM//#define CAMERA_MODEL_AI_THINKER // Has PSRAM//#define CAMERA_MODEL_TTGO_T_JOURNAL // No PSRAM//#define CAMERA_MODEL_XIAO_ESP32S3 // Has PSRAM// ** Espressif Internal Boards **//#define CAMERA_MODEL_ESP32_CAM_BOARD//#define CAMERA_MODEL_ESP32S2_CAM_BOARD//#define CAMERA_MODEL_ESP32S3_CAM_LCD#define CAMERA_MODEL_DFRobot_FireBeetle2_ESP32S3 // Has PSRAM//#define CAMERA_MODEL_DFRobot_Romeo_ESP32S3 // Has PSRAM
#include "camera_pins.h"
#include "DFRobot_AXP313A.h"
DFRobot_AXP313A axp;
// ===========================// Enter your WiFi credentials// ===========================const char* ssid = "";const char* password = "";
void startCameraServer();
void setupLedFlash(int pin);
void setup() { Serial.begin(115200); Serial.setDebugOutput(true); Serial.println();
while(axp.begin() != 0){ Serial.println("init error"); delay(1000); }
axp.enableCameraPower(axp.eOV2640); // Power the camera
camera_config_t config; config.ledc_channel = LEDC_CHANNEL_0; config.ledc_timer = LEDC_TIMER_0; config.pin_d0 = Y2_GPIO_NUM; config.pin_d1 = Y3_GPIO_NUM; config.pin_d2 = Y4_GPIO_NUM; config.pin_d3 = Y5_GPIO_NUM; config.pin_d4 = Y6_GPIO_NUM; config.pin_d5 = Y7_GPIO_NUM; config.pin_d6 = Y8_GPIO_NUM; config.pin_d7 = Y9_GPIO_NUM; config.pin_xclk = XCLK_GPIO_NUM; config.pin_pclk = PCLK_GPIO_NUM; config.pin_vsync = VSYNC_GPIO_NUM; config.pin_href = HREF_GPIO_NUM; config.pin_sccb_sda = SIOD_GPIO_NUM; config.pin_sccb_scl = SIOC_GPIO_NUM; config.pin_pwdn = PWDN_GPIO_NUM; config.pin_reset = RESET_GPIO_NUM; config.xclk_freq_hz = 20000000; config.frame_size = FRAMESIZE_UXGA; config.pixel_format = PIXFORMAT_JPEG; // for streaming //config.pixel_format = PIXFORMAT_RGB565; // for face detection/recognition config.grab_mode = CAMERA_GRAB_WHEN_EMPTY; config.fb_location = CAMERA_FB_IN_PSRAM; config.jpeg_quality = 12; config.fb_count = 1;
// if PSRAM IC present, init with UXGA resolution and higher JPEG quality // for larger pre-allocated frame buffer. if(config.pixel_format == PIXFORMAT_JPEG){ if(psramFound()){ config.jpeg_quality = 10; config.fb_count = 2; config.grab_mode = CAMERA_GRAB_LATEST; } else { // Limit the frame size when PSRAM is not available config.frame_size = FRAMESIZE_SVGA; config.fb_location = CAMERA_FB_IN_DRAM; } } else { // Best option for face detection/recognition config.frame_size = FRAMESIZE_240X240;#if CONFIG_IDF_TARGET_ESP32S3 config.fb_count = 2;#endif }
#if defined(CAMERA_MODEL_ESP_EYE) pinMode(13, INPUT_PULLUP); pinMode(14, INPUT_PULLUP);#endif
// camera init esp_err_t err = esp_camera_init(&config); if (err != ESP_OK) { Serial.printf("Camera init failed with error 0x%x", err); return; }
sensor_t * s = esp_camera_sensor_get(); // initial sensors are flipped vertically and colors are a bit saturated if (s->id.PID == OV3660_PID) { s->set_vflip(s, 1); // flip it back s->set_brightness(s, 1); // up the brightness just a bit s->set_saturation(s, -2); // lower the saturation } // drop down frame size for higher initial frame rate if(config.pixel_format == PIXFORMAT_JPEG){ s->set_framesize(s, FRAMESIZE_QVGA); }
#if defined(CAMERA_MODEL_M5STACK_WIDE) || defined(CAMERA_MODEL_M5STACK_ESP32CAM) s->set_vflip(s, 1); s->set_hmirror(s, 1);#endif
#if defined(CAMERA_MODEL_ESP32S3_EYE) s->set_vflip(s, 1);#endif
// Setup LED FLash if LED pin is defined in camera_pins.h#if defined(LED_GPIO_NUM) setupLedFlash(LED_GPIO_NUM);#endif
WiFi.begin(ssid, password); WiFi.setSleep(false);
while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected");
startCameraServer();
Serial.print("Camera Ready! Use 'http://"); Serial.print(WiFi.localIP()); Serial.println("' to connect");}
void loop() { // Do nothing. Everything is done in another task by the web server delay(10000);}
There are a few points to note in the code:
1. Macro definitions select the appropriate camera model.
// ===================// Select camera model// ===================//#define CAMERA_MODEL_WROVER_KIT // Has PSRAM// #define CAMERA_MODEL_ESP_EYE // Has PSRAM//#define CAMERA_MODEL_ESP32S3_EYE // Has PSRAM//#define CAMERA_MODEL_M5STACK_PSRAM // Has PSRAM//#define CAMERA_MODEL_M5STACK_V2_PSRAM // M5Camera version B Has PSRAM//#define CAMERA_MODEL_M5STACK_WIDE // Has PSRAM//#define CAMERA_MODEL_M5STACK_ESP32CAM // No PSRAM//#define CAMERA_MODEL_M5STACK_UNITCAM // No PSRAM//#define CAMERA_MODEL_AI_THINKER // Has PSRAM//#define CAMERA_MODEL_TTGO_T_JOURNAL // No PSRAM//#define CAMERA_MODEL_XIAO_ESP32S3 // Has PSRAM// ** Espressif Internal Boards **//#define CAMERA_MODEL_ESP32_CAM_BOARD//#define CAMERA_MODEL_ESP32S2_CAM_BOARD//#define CAMERA_MODEL_ESP32S3_CAM_LCD#define CAMERA_MODEL_DFRobot_FireBeetle2_ESP32S3 // Has PSRAM//#define CAMERA_MODEL_DFRobot_Romeo_ESP32S3 // Has PSRAM
2. The SSID and password of the wireless router must be filled in correctly.
// ===========================// Enter your WiFi credentials// ===========================const char* ssid = "";const char* password = "";
3. Power the camera
axp.enableCameraPower(axp.eOV2640); // Power the camera
4. The board needs to connect an external antenna, otherwise it may not be able to connect to the router.
Compile and download the program to the board, ensuring that the local area network access to the network camera can be used normally.
2
Network Penetration for Network Camera
For network penetration, we use the service provided by the software Peanut Shell.
Download the app from the official website: https://hsk.oray.com/
After installation, click on the internal network penetration service to create a new mapping, as shown in the figure below:
Fill in the basic information for the new mapping. Please note that the internal network host and internal network port are the host and port of the local area network camera (the port defaults to 80), as shown in the figure below:
After completing the new mapping, you can see the newly added device list in the app, as shown in the figure below:
Copy the access URL and open it in the browser: http://2j90962r69.goho.co:47918/
Even if you are not on the same local area network, you can still access the camera normally.
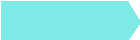
-
Learning HarmonyOS Development – Java UI Framework (Position and Adaptive Box Layout)
-
Python Data Visualization: How to Choose the Right Chart for Visualization?
-
Magnetic Coupling Resonant Wireless Power Supply Device
-
Python Qt GUI Design: Tables and Trees (Enhancement – Part 1)
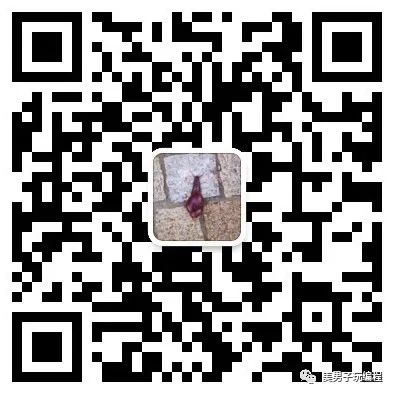
