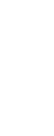
Click the “Blue Words” to Follow Us

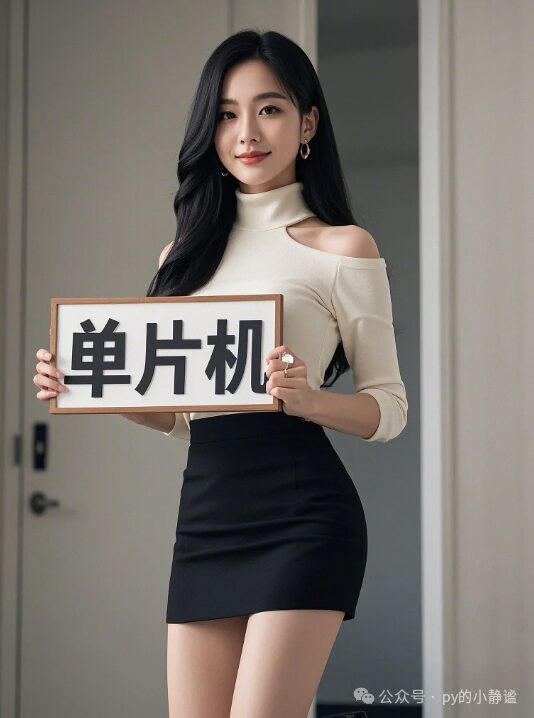
Have you ever thought about using a small Arduino board to achieve smart interconnection between devices? Today, we will unveil the “golden key” to IoT communication – the MQTT protocol! Let your microcontroller easily achieve remote control and data transmission.
What is MQTT? The “WeChat” of Communication

Imagine MQTT as an instant messaging tool between hardware devices. Traditional communication methods are like writing letters, while MQTT is the “hardware WeChat” that sends messages instantly. It is lightweight and efficient, especially suitable for resource-constrained embedded devices.
Key Features:
-
Lightweight communication -
Low power consumption -
Reliable transmission -
Real-time bidirectional communication
Prepare Your “Communication Toolbox”

Before we start, we need to prepare:
-
Arduino development board (such as UNO or NodeMCU) -
ESP8266/ESP32 WiFi module -
A computer -
MQTT server (recommended to use a free public server)
Hardware Connection: Building the Communication Bridge

// ESP8266 MQTT wiring example
#define WIFI_SSID "your_wifi_name"
#define WIFI_PASS "your_wifi_password"
#define MQTT_SERVER "broker.hivemq.com"
#define MQTT_PORT 1883
Tip: Choosing a development board that supports WiFi can greatly simplify the communication process!
Code Practice: Three Steps to Achieve MQTT Communication

1. Include Necessary Libraries
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
WiFiClient espClient;
PubSubClient client(espClient);
2. Connect to WiFi and MQTT Server
void setup() {
// Connect to WiFi
WiFi.begin(WIFI_SSID, WIFI_PASS);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
// Configure MQTT server
client.setServer(MQTT_SERVER, MQTT_PORT);
client.setCallback(messageReceived);
}
3. Publish and Subscribe to Messages
void loop() {
if (!client.connected()) {
reconnect();
}
// Publish message
client.publish("home/temperature", "25.5");
// Subscribe to topic
client.subscribe("home/control");
}
// Message callback function
void messageReceived(char* topic, byte* payload, unsigned int length) {
// Handle received message
}
Practical Scenario: Smart Home Monitoring

Imagine a scenario where your Arduino monitors the temperature and humidity in your home via MQTT and can remotely control appliances!
Application Cases:
-
Remote air conditioning control -
Real-time environmental data reporting -
Device status monitoring
Development Tips

-
Choose a development board that supports WiFi -
Pay attention to the stability of the MQTT server -
Ensure communication security -
Control message size
Continue Your IoT Journey

This is just the tip of the iceberg of MQTT! We recommend you:
-
Deepen your understanding of network communication principles -
Try building more complex IoT projects -
Follow open-source MQTT platforms
Behind every smart device, there is a communication protocol working silently. MQTT makes your hardware no longer lonely!
Keywords: Arduino IoT, MQTT Communication, Smart Home, ESP8266 Development, Embedded Communication # Deep Dive into MQTT: From Basics to Advanced Practice
In the previous article, we briefly understood the basic principles and simple applications of MQTT. Now, let’s explore more details of MQTT and take your IoT skills to the next level!
The Secret Weapon of MQTT: QoS Service Quality

Imagine you are sending an important message to a friend, you certainly hope they receive it 100%. The Quality of Service (QoS) levels of MQTT are such a guarantee mechanism.
Three Levels of QoS: From Uncertain to 100% Reliable
- QoS 0
: At most once (fire and forget)
* Fast but no delivery guarantee
* Similar to regular messages in WeChat
- QoS 1
: At least once
* Ensures message is received
* May send duplicates
- QoS 2
: Exactly once
* The strictest transmission mode
* Suitable for critical data transmission
// QoS setting example
client.publish("topic", "message", QoS_LEVEL);
Secure Communication: Protect Your Data

IoT security is always a top priority! MQTT provides multiple security mechanisms:
Authentication Mechanisms
-
Username/Password verification -
SSL/TLS encryption -
Certificate authentication
// Secure connection example
client.setServer(MQTT_SERVER, 8883); // secure port
client.setCredentials("username", "password");
Advanced Topic Design: The Art of Architecture

Best Practices for Topic Naming
-
Use forward slashes to separate -
From general to specific -
Example: <span>home/livingroom/temperature</span>
Wildcard Usage
<span>+</span>
: Single-level wildcard <span>#</span>
: Multi-level wildcard
// Subscribe to all living room topics
client.subscribe("home/livingroom/#");
Advanced Practice: Multi-Device Collaboration

Bridging Example: Temperature and Humidity Monitoring System
-
Sensor nodes collect data -
Gateway summarizes and forwards -
Cloud storage and analysis
// Gateway forwarding example
void forwardData(float temperature, float humidity) {
String payload = "{\"temp\": " + String(temperature) +
", \"humi\": " + String(humidity) + "}";
client.publish("gateway/sensors", payload.c_str());
}
Performance Optimization: Making Communication Smarter

Heartbeat and Keep-Alive
-
Send heartbeat regularly -
Check offline status
unsigned long lastReconnectAttempt = 0;
void checkConnection() {
if (!client.connected()) {
long now = millis();
if (now - lastReconnectAttempt > 5000) {
reconnect();
lastReconnectAttempt = now;
}
}
}
Common Pitfalls and Solutions

-
Unstable network
* Increase reconnection mechanism
* Use caching
-
Memory constraints
* Control message size
* Release resources in time
Future Prospects: The Evolution of MQTT

The Internet of Things is developing rapidly, and MQTT 5.0 brings more features:
-
Shared subscriptions -
Stronger error reporting -
Enhanced authentication
Recommended Learning Path

-
Deeply research protocol specifications -
Practice open-source projects -
Follow community dynamics -
Try commercial solutions
In the world of IoT, there are no boundaries, only imagination!
Keywords: Advanced MQTT, IoT Security, Multi-Device Communication, Embedded Architecture, Sensor Networks
Encourage Yourself

Like Before Leaving