
Exploring FastAPI: A Powerful Python Framework for Backend Development
Hello everyone, I am Xiangqian! Today I want to introduce you to a super powerful Python web framework – FastAPI. It is not only fast and easy to use, but also comes with built-in API documentation, making it a magical tool for backend development! Let’s explore the charm of FastAPI together!
1.
What is FastAPI?
FastAPI is a modern, fast (high-performance) web framework for building APIs, based on Python 3.6+ standard type hints.
Its main features are speed, quick coding, few bugs, intuitive, easy to learn, concise, robust, and standards-based.
Sounds impressive, right?
That’s right, it is that awesome!
2.
Why Choose FastAPI?
1.Outstanding Performance: The speed of FastAPI can rival NodeJS and Go, making it one of the fastest Python web frameworks. 2.Simple and Easy to Use: Based on Python type hints, the code is concise and intuitive. 3.Automatically Generate API Documentation: Get beautiful interactive API documentation without extra work. 4.Standards-Based: Fully compatible with OpenAPI and JSON Schema standards.
3.
Getting Started with FastAPI
We need to install FastAPI and an ASGI server:
“`bash
pip install fastapi
pip install uvicorn
“`
Now, let’s create a simple FastAPI application:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/”)
async def root():
return {“message”: “Hello World”}
“`
Run Command: uvicorn main:app –reload
It’s that simple, our first FastAPI application is complete!
Run it using the command <span>uvicorn main:app --reload</span>
, then visit <span>http://127.0.0.1:8000</span>
in your browser, and you will see a JSON formatted “Hello World” message.
4.
Path Parameters and Query Parameters
FastAPI makes handling path parameters and query parameters super simple:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/items/{item_id}”)
async def read_item(item_id: int, q: str = None):
if q:
return {“item_id”: item_id, “q”: q}
return {“item_id”: item_id}
“`
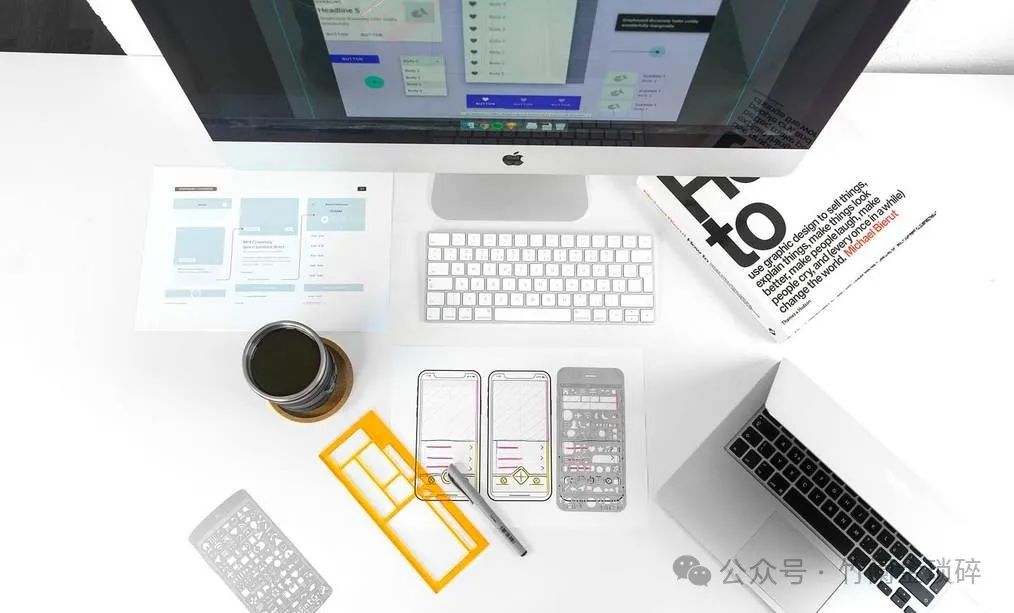
In this example, <span>item_id</span>
is a path parameter, and <span>q</span>
is an optional query parameter. FastAPI will automatically perform type checking and conversion!
5.
Request Body
Handling POST requests and request bodies is also a piece of cake:
“`python
from fastapi import FastAPI
from pydantic import BaseModel
class Item(BaseModel):
name: str
price: float
is_offer: bool = None
app = FastAPI()
@app.post(“/items”)
async def create_item(item: Item):
return {“item_name”: item.name, “item_price”: item.price}
“`

Using Pydantic models, we can easily define the structure of the request body, and FastAPI will automatically handle JSON serialization and deserialization.
6.
Automatically Generate API Documentation
This might be the coolest feature of FastAPI!
Visit the <span>/docs</span>
path, and you will see a beautiful Swagger UI interface containing all the information and interactive documentation of your API.
If you prefer the ReDoc style, you can visit the <span>/redoc</span>
path.
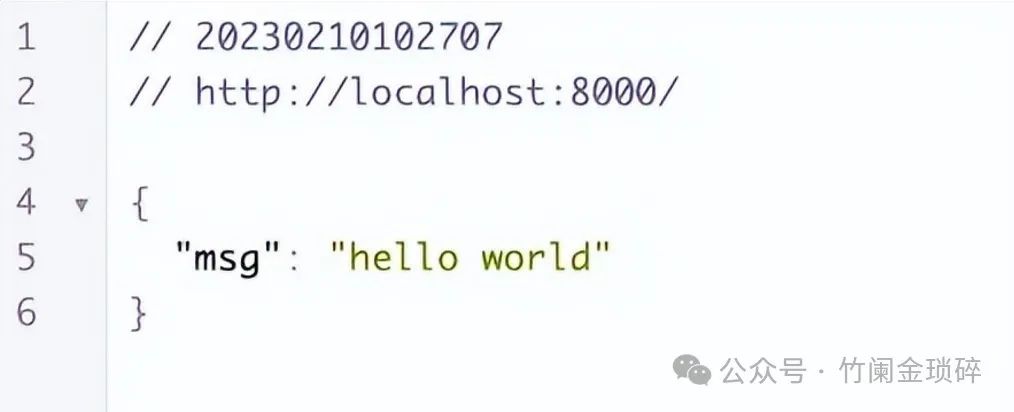
7.
Tips
1. FastAPI supports asynchronous programming, allowing full utilization of Python’s <span>async/await</span>
syntax. 2. Using type hints not only improves code readability but also helps IDEs provide better autocompletion. 3. FastAPI’s dependency injection system is very powerful and can be used to handle complex issues like security and database sessions.
Friends, today’s Python learning journey ends here!
FastAPI is truly a very powerful and friendly framework, and I believe you can’t wait to try it out!
Remember to get your hands on the code, and feel free to ask Xiangqian in the comments if you have any questions.
Wishing everyone a happy learning experience, may your Python skills improve!
Previous Reviews
1. Write a WeChat Bot with Python, Never Have a Dull Moment in Moments
2. A One-Click Python Reporting Tool!
3. Let Python Become Your Virtual Image Designer
