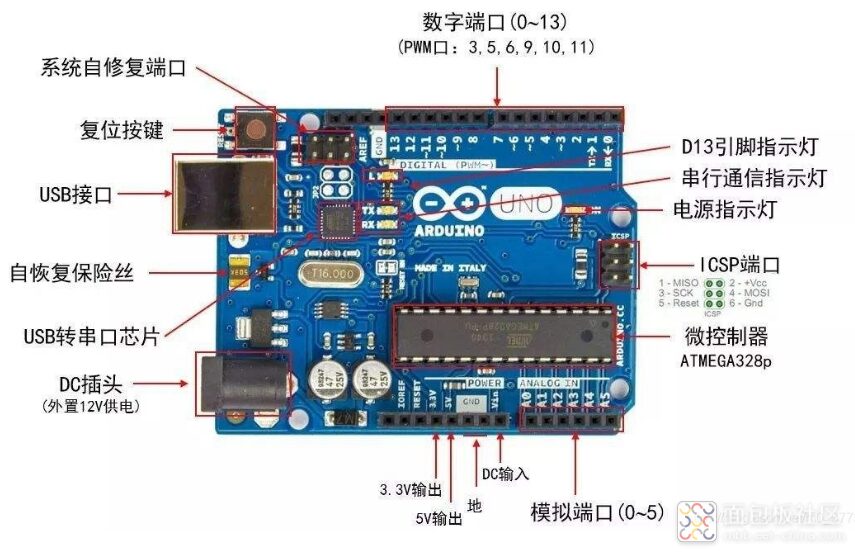
Performance Parameters① Main Control Chip: ATmega328P② Digital I/O Pins: 14③ PWM Pins: 6④ Storage (Code Space): 32KB⑤ RAM (Runtime Storage): 2KB; EEPROM (Power-Off Storage): 1KB⑥ Oscillator: 16MHzOnline Simulation: https://wokwi.com/
Arduino UNO Tutorial①: Install Arduino IDE
Arduino UNO Tutorial②: Development Board and LED TestingArduino UNO Tutorial③: Button Control of LED, with ExercisesArduino UNO Tutorial④: Analog Quantity, Using ADC to Measure VoltageArduino UNO Tutorial⑤: External Interrupt, with ExercisesArduino UNO Tutorial⑥: Serial Communication, Sending and ReceivingArduino UNO Tutorial⑦: 0.96 Inch OLED Display
(1) IntroductionThe 0.96 inch OLED display is a small display device commonly used in electronic projects. It uses the IIC (I2C) communication protocol for data transmission, featuring low power consumption and high contrast. The resolution of this display is 128×64, supporting various display modes such as text and graphics. Its driver chip is usually SSD1306, allowing flexible control of screen brightness, contrast, and other parameters.
Screen Parameters Operating Voltage: 3.3V Operating Current: 9MA Module Size: 27.3 x 27.8 MM Pixel Size: 128(H) x 64(V) RGB Driver Chip: SSD1306 Communication Protocol: IIC Pin Count: 4 Pin (2.54mm Pitch Pin Header)(2) Library FileU8G2 Library Project Address: https://github.com/olikraus/u8g2The U8g2 library is a monochrome graphics library for embedded devices, known as the “Universal 8bit Graphics Library version 2,” developed by Oliver Kraus, specifically designed for microcontrollers to drive various monochrome LCD and OLED displays. Below is a detailed introduction to the Arduino U8g2 library, including its main functions:1. U8g2 Library Features1. Cross-Platform: U8g2 can run on different microcontroller platforms, such as Arduino, STM32, ESP32/ESP8266, etc.2. Multiple Display Support: Supports various monochrome display devices, including LCD and OLED.3. Font Support: U8g2 includes various fonts, including different sizes and special fonts (including Chinese).4. Ease of Use: The API design of U8g2 is simple and easy to use, allowing developers to get started quickly.5. Low Memory Usage: U8g2 considers the resource limitations of embedded devices in its design, minimizing memory usage.6. Open Source: U8g2 is open source and can be freely used and modified.2. U8g2 Library FunctionsThe U8g2 library provides a rich API for controlling various displays and drawing text, graphics, and other elements on the screen. Here are some commonly used functions:1. Initialization Functionsu8g2_Setup(): Initializes the U8g2 object based on the specific display device and configuration. For example, u8g2_Setup_ssd1306_i2c_128x64_noname_f() is used to initialize the SSD1306 OLED display. u8g2.begin() or u8g2.beginSimple(): Constructs the U8G2 object and initializes the display controller.2. Display Control Functionsu8g2.initDisplay(): Initializes the display controller, writing key display parameters. u8g2.clearDisplay(): Clears the screen content. u8g2.setPowerSave(): Turns power-saving mode on or off. u8g2.clearBuffer(): Clears the buffer without clearing the screen. Usually paired with u8g2.sendBuffer() to refresh the display.3. Drawing Functionsu8g2.drawBox(): Draws a filled square. u8g2.drawCircle(): Draws an empty circle. u8g2.drawDisc(): Draws a filled circle. u8g2.drawEllipse(): Draws an empty ellipse. u8g2.drawFilledEllipse(): Draws a filled ellipse. u8g2.drawFrame(): Draws an empty square (rectangle frame). u8g2.drawGlyph(): Draws a symbol from the font set. u8g2.drawHLine(): Draws a horizontal line. u8g2.drawLine(): Draws a line between two points. u8g2.drawPixel(): Draws a pixel. u8g2.drawRBox(): Draws a rounded filled square. u8g2.drawRFrame(): Draws a rounded empty square. u8g2.drawStr(): Draws a string at a specified position. u8g2.drawTriangle(): Draws a filled triangle. u8g2.drawUTF8(): Draws UTF8 encoded characters (can display Chinese characters). u8g2.drawVLine(): Draws a vertical line. u8g2.drawXBM()/drawXBMP(): Draws an XBM format image.4. Display Configuration Functionsu8g2.setFont(): Sets the currently used font. u8g2.setDisplayRotation(): Sets the rotation angle of the display. u8g2.setAutoPageClear(): Sets whether to automatically clear the buffer. u8g2.setBitmapMode(): Sets bitmap mode. u8g2.setCursor(): Sets the drawing cursor position. u8g2.setDrawColor(): Sets the drawing color (for monochrome displays, usually only supports black and white).5. Other Functionsu8g2.disableUTF8Print(): Disables UTF8 printing function. u8g2.enableUTF8Print(): Enables UTF8 printing function. u8g2.home(): Resets the display cursor position to the origin (0,0). u8g2.firstPage()/u8g2.nextPage(): Control functions for paginated drawing of large images or graphics. u8g2.sendBuffer(): Sends the contents of the buffer to the screen to refresh the display. u8g2.getBufferPtr(): Gets the address of the buffer space. u8g2.getDisplayHeight()/u8g2.getDisplayWidth(): Gets the height and width of the display. u8g2.getMaxCharHeight()/u8g2.getMaxCharWidth(): Gets the maximum height and width of characters in the current font.(3) Program① First, include the standard Arduino library, as well as the U8g2 library; the Wire library is the IIC communication library used with U8g2.
#include <arduino.h>#include <u8g2lib.h>#include <wire.h></wire.h></u8g2lib.h></arduino.h>
② Define macros BOARD_I2C_SCL as A5 and BOARD_I2C_SDA as A4 because A5 is SCL and A4 is SDA in Arduino UNO. Declare the U8g2 library, NONAME_F_SW_I2C for software simulation, and can also use hardware simulation. U8G2_R0 for no rotation of the screen. Set the clock to SCL pin A5 and data to SDA pin A4. Reset function is U8X8_PIN_NONE, not used.
#define BOARD_I2C_SCL A5#define BOARD_I2C_SDA A4 U8G2_SSD1306_128X64_NONAME_F_SW_I2C u8g2(U8G2_R0, /* clock=*/ BOARD_I2C_SCL, /* data=*/ BOARD_I2C_SDA, /* reset=*/ U8X8_PIN_NONE);
③ The initialization function sets the baud rate to 115200 and outputs a test message. u8g2 starts initialization. u8g2 sets u8g2_font_ncenB08_tr font (U8g2 has many built-in fonts, check on GitHub).
void setup() { Serial.begin(115200); Serial.println("Init u8g2 ...."); u8g2.begin(); u8g2.setFont(u8g2_font_ncenB08_tr); // Set font delay(100); }
④ The loop function clears the screen’s buffer, outputs “good” at position (10, 10) on the screen, and uploads this data to the buffer for display.
void loop() { u8g2.clearBuffer(); // Clear buffer Serial.println("u8g2 showing chars ...."); u8g2.setCursor (10, 10); u8g2.println("good"); u8g2.sendBuffer(); delay(1000);}
⑤ Experimental Phenomenon
#include <arduino.h>#include <u8g2lib.h>#include <wire.h>#define BOARD_I2C_SCL A5#define BOARD_I2C_SDA A4 U8G2_SSD1306_128X64_NONAME_F_SW_I2C u8g2(U8G2_R0, /* clock=*/ BOARD_I2C_SCL, /* data=*/ BOARD_I2C_SDA, /* reset=*/ U8X8_PIN_NONE); void setup() { // Put your setup code here, to run once: Serial.begin(115200); Serial.println("Init u8g2 ...."); u8g2.begin(); u8g2.setFont(u8g2_font_ncenB08_tr); // Set font delay(100);} void loop() { u8g2.clearBuffer(); // Clear buffer Serial.println("u8g2 showing chars ...."); u8g2.setCursor (10, 10); u8g2.println("good"); u8g2.sendBuffer(); delay(1000);}</wire.h></u8g2lib.h></arduino.h>
Free Application Development Board

Submission/Promotion/Cooperation/Join Group Please scan the QR code to add WeChat
(Please indicate your intentions, for joining groups please indicate city-name-industry job information)