1.About This Book
Access method at the end of the article!!!
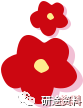
The content of this book is compiled from the frequently encountered written and interview questions during the early recruitment phase of the 2021 autumn recruitment by myself and my classmates, as well as from online blogs, interview experiences, WeChat public accounts, personal websites, etc.
During the writing of this book, if there are significant references, the sources will be noted alongside. The entire early recruitment, autumn recruitment process, and the compilation of this book owe much to the previous sharing of interview experiences and materials by my classmates.
1. This book aims to minimize length; some content is not elaborated upon but links are provided, and everything is organized quite clearly.
2. Some questions only provide answers; think more about the underlying principles to understand.
3. Trust me, if you patiently clarify all the knowledge points mentioned in the text, there will be no issues with C/C++ software development, embedded software development, or certain front-end and back-end positions.
This book organizes knowledge points and interview questions mainly involving C, C++, STL standard library and algorithms, operating systems, Linux, computer networks, data structures, commonly used algorithm problems, databases, design patterns, multithreading programming, and common intelligence questions. Since the author has a communication background, there is a bit of communication-related content and OFDM knowledge at the end of the book. This book is suitable for students who have a basic understanding of fundamental knowledge concepts but are not very solid, or for those who struggle to connect knowledge points. It is most suitable for students preparing for internships, early recruitment, and autumn recruitment interviews, making it very useful for last-minute interview preparation. This text includes at least 90% of the knowledge points for the aforementioned positions.
This is not a textbook; it is definitely not as comprehensive and systematic as professional-related books. It is merely a resource for interviews and consolidating what has been learned. If you are not yet at the point of imminent interviews, it is recommended to spend more time systematically learning foundational knowledge while expanding your knowledge base, as this is a long-term strategy. During this time, you need acceleration, not speed. You do not have to read from start to finish; you can read any chapter based on the knowledge points you wish to understand.
Due to the compilation of this book during the early recruitment and autumn recruitment period, time was tight, and errors or typos are inevitable. If you find any errors, suggestions, or additions during your reading, or have any other questions, you can contact me via the email below.
2.Table of Contents
Access method at the end of the article!!!!
Due to the lengthy table of contents, scroll to view the entire table!
1. About This Book
1. Introduction
2. Object-Oriented Programming
3. Reading Method
4. Corrections and Errata
2. C++
5. Difference between references and pointers?
6. Explain references from the assembly level.
7. Parameter passing with pointers and references in C++.
8. Difference between formal and actual parameters?
9. Usage and function of static?
10. When are static variables initialized?
11. const?
12. Understanding and application of const member functions?
13. Usage of pointers and const? How to differentiate const modified pointers?
14. How are constants defined in C++? Where are constants stored in memory?
15. mutable
16. Usage of extern?
17. Convert int to string and string to int? Internal implementation of strcat, strcpy, strncpy, memset, memcpy?
18. Deep copy vs shallow copy?
19. What is a C++ template and how is it implemented at the underlying level?
20. Introduction to static binding and dynamic binding.
21. Difference between C language struct and C++ struct.
22. Can virtual functions be declared as inline?
23. Introduction to all constructors in C++.
24. Under what circumstances is the copy constructor called?
25. Why must the copy constructor use reference passing and not value passing?
26. When is a synthesized constructor needed?
27. When is a synthesized copy constructor needed?
28. Location of the virtual function table and virtual functions in memory for C++ classes.
29. If a class is instantiated multiple times, do they share the virtual function table?
30. How should the compiler handle the virtual function table?
31. Initialization methods for class members? Execution order of constructors? Why is using the member initialization list faster?
32. Member list initialization?
33. When is a member initialization list needed? What is the process?
34. Why can’t constructors be virtual functions? Why should destructors be virtual functions?
35. Role of destructors, how do they work?
36. Can constructors and destructors call virtual functions, and why?
37. Can constructors and destructors call virtual functions?
38. Execution order of constructors? Execution order of destructors? What happens inside the constructor? What happens in the copy constructor?
39. Role of virtual destructors, should the parent class destructor be set as virtual?
40. Can constructors and destructors throw exceptions?
41. How to implement a class that can only be statically or dynamically allocated?
42. If a class is to be used as a base class, why must it be defined and not just declared?
43. Under what circumstances is a default constructor automatically generated?
44. Expansion process of constructors?
45. Process of expansion for user-defined destructors?
46. Execution algorithm of constructors?
47. Which functions cannot be virtual functions?
48. Mechanism of virtual function calls.
49. What is class inheritance?
50. What is multiple inheritance?
51. What is composition?
52. Why can’t abstract base classes create objects?
53. Definition of pure virtual functions.
54. The difference between virtual functions and pure virtual functions.
55. When does a class get destructed?
56. Why must friend functions be declared inside the class?
57. The three major characteristics of C++ object-oriented programming are encapsulation, inheritance, and polymorphism.
58. Brief introduction to polymorphism in C++?
59. Differences between overloading and overriding in C++.
60. Cost of virtual functions?
61. Implementing C++ inheritance using C language.
62. How do objects convert between each other in the inheritance mechanism? How do pointers and references convert?
63. Four types of type conversion in C++.
64. Why use static_cast for conversion instead of C language conversions?
65. Advantages and disadvantages of composition vs inheritance?
66. Lvalue and rvalue.
67. Summary of concepts of lvalue and rvalue.
68. Move constructor.
69. Compilation and linking process of C language?
70. Differences and applications of vector and list? How to find the second-to-last element of a vector or list?
71. Implementation of STL vector, deletion of elements, how does the iterator change? Why is it double capacity expansion? Releasing space?
72. Deleting an element inside a container.
73. How STL iterators are implemented.
74. Differences between set and hash_set.
75. Differences between hash_map and map.
76. How map and set are implemented, how does a red-black tree manage to implement both types of containers? Why use a red-black tree?
77. How to use STL standard library in shared memory?
78. How many ways to insert into a map?
79. Differences between unordered_map (hash_map) and map in STL, how hash_map resolves conflicts and expands.
80. Out-of-bounds access for vector indices, out-of-bounds access for map indices? Does vector release space when deleting elements?
81. Differences between map[] and find?
82. Differences between list and queue in STL.
83. Allocator and deallocator in STL.
84. What happens during STL hash_map expansion?
85. How to create a map?
86. How are additions and deletions performed in vector? Why is it 1.5 times?
87. Underlying implementation of C++ sort sorting algorithm.
88. Function pointers?
89. Relationship and differences between C and C++.
90. Differences between NULL and nullptr in C++.
91. Memory allocation in C/C++.
92. Memory allocation model of c/c++, explain stack, heap, static storage area in detail?
93. What is a wild pointer? How to detect memory leaks?
94. Differences between dangling pointers and wild pointers?
95. Memory leaks.
96. Locating memory overflow.
97. What is memory alignment?
98. Why memory alignment?
99. Memory alignment rules.
100. What is the function of byte alignment?
101. Usage of #pragma in C language.
102. Differences between new and malloc?
103. Differences between malloc/calloc/realloc?
104. delete p; vs delete[] p, allocator.
105. Implementation principles of new and delete, how does delete know the size of the memory to be released?
106. Can memory allocated by malloc be released by delete?
107. Order of function parameters being pushed onto the stack.
108. Differences between heap and stack.
109. Advantages and disadvantages of heap vs stack.
110. Virtual memory management in kernel space.
111. Implementation principles of malloc and free?
112. Differences between malloc, realloc, and calloc.
113. Differences between __stdcall and __cdecl?
114. Handwritten string functions strcat, strcpy, strncpy, memset, memcpy implementation.
115. Use smart pointers to manage memory resources, RAII.
116. Handwritten implementation of smart pointer class.
117. Comparison of structure variable equality.
118. Bitwise operations.
119. Changes in the stack during function calls, which comes first: return value or parameter variable?
120. How to determine if two floating point numbers are equal?
121. Macro definition to get the larger of two numbers.
122. Usage of define, const, typedef, inline?
123. Implementation principles of printf?
124. Order of #include and the difference between angle brackets and double quotes.
125. Lambda functions.
126. Differences between template classes and template functions?
127. Why are template classes generally placed in a single .h file?
128. Access permissions and inheritance permissions issues of class members in C++.
129. Differences between cout and printf?
130. Overloading operators?
131. Function matching principles for overloaded functions.
132. Differences between definition and declaration.
133. Differences between global variables and static variables.
134. Differences between static functions and ordinary functions?
135. Differences between static members and ordinary members.
136. Understanding ifdef endif.
137. Implicit conversion, how to eliminate implicit conversion?
138. Memory structure of virtual functions, what about the memory structure of virtual functions in diamond inheritance?
139. Advantages and disadvantages of multiple inheritance, how should developers view multiple inheritance?
140. Which is better, ++it or it++? Why?
141. How does C++ handle multiple exceptions?
142. Can templates and implementations be written in different files? Why?
143. What happens when delete this is called in a member function? Can the object still be used?
144. Three smart pointers.
145. How to use smart pointers? How to solve circular references in smart pointers?
146. Role of smart pointers.
147. Role of auto_ptr.
148. Differences between class, union, and struct.
149. Dynamic binding vs static binding.
150. Dynamic compilation vs static compilation.
151. Differences between dynamic linking and static linking.
152. How to swap two numbers without using extra space?
153. Differences between strcpy and memcpy.
154. Briefly describe the differences between strcpy, sprintf, and memcpy.
155. Differences between strcpy and strncpy? Which function is safer?
156. Memory structure when executing int main(int argc, char *argv[]).
157. Role of the volatile keyword?
158. Discuss big-endian and little-endian, how to detect (three methods).
159. Methods to view memory.
160. Empty class.
161. What default items are added to an empty class? How to write it?
162. What is the standard library?
163. Relationship between const char* and string, parameter passing issues?
164. char * vs char[]
165. Differences between sizeof and strlen.
166. For char* and char[], calculate sizeof().
167. new, delete, operator new, operator delete, placement new, placement delete.
168. What is the size of an empty class? Why?
169. Calculate the sizes of the following classes.
170. Size of class objects.
171. Several ways to pass parameters in functions.
172. What are the characteristics of using “references” as function parameters?
173. When do you use pointers as parameters and when do you use references, and why?
174. Which to choose for large memory allocation? Where are C++ variables stored? Where is the size of variables stored? Where is the symbol table stored?
175. Why are big-endian and little-endian necessary, what is the role of functions like htol?
176. Can static functions be defined as virtual functions? Const functions?
177. What changes occur in the stack when the this pointer calls member variables?
178. Design a class to count the number of subclasses.
179. How to quickly locate where an error occurs.
180. Code implementation to clear a specific bit of a variable or set it to 1.
181. Write comparison statements for variables a of types BOOL, int, float, and pointer with “zero”.
182. Issues with local variables and global variables?
183. Differences between arrays and pointers?
184. How to prevent a class from being instantiated in C++? When to declare a constructor as private?
185. How to prohibit the automatic generation of a copy constructor?
186. assert and NDEBUGE.
187. Differences between Debug and Release.
188. Does the main function have a return value?
189. Write a template function to compare sizes.
190. How to implement a function that runs before the main function in C++?
191. What happens when memset(this,0,sizeof(*this)) is called in a member function?
192. Principles of method calls (stack, assembly).
193. How MFC message processing is encapsulated?
194. Function pointers.
195. Role of callback functions.
196. Generation of random numbers.
197. Differences between for(;;) and while(true).
198. Common tree structures in data structures.
199. What is a balanced binary tree?
200. Properties of red-black trees.
201. Insertion and rotation of red-black trees.
202. Differences and connections between binary balanced trees, red-black trees, B-trees, and B+ trees.
203. The entire process from hello world program start to printing on the screen?
3. STL Standard Library and Algorithms
204. Introduction to C++ STL.
205. Implementation of sequence containers in STL:
206. Points to note and performance analysis of vector usage.
207. Differences between map and set, how are they implemented?
208. Deleting elements from STL iterators.
209. Role of iterators in STL, why iterators when there are pointers?
210. Answer the difference between resize and reserve in STL.
211. How does vector store data of varying sizes?
4. Operating Systems
212. Characteristics of operating systems.
213. Differences between concurrency and parallelism.
214. What is a process? What is a thread?
215. Differences between processes and threads.
216. What resources do threads share?
217. Resources of processes and threads.
218. Application scenarios for multithreading and processes.
219. Why use multithreading?
220. When to use multiple processes? When to use multiple threads?
221. What advantages do threads have over processes?
222. What is a coroutine?
223. Differences between programs, processes, and jobs?
224. Process creation process? What functions and data structures are needed?
225. Detailed explanation of creating child processes and fork.
226. Parent and child processes.
227. How do child processes communicate with parent processes?
228. Global variables in parent and child processes.
229. How many inter-process communication methods are there, and what are their differences?
230. What is thread safety? How to achieve it?
231. Ways to synchronize threads?
232. Critical sections, mutexes, semaphores, event objects.
233. Differences between mutexes and read-write locks.
234. Differences between synchronous and asynchronous communication.
235. Process state transition diagram.
236. What is process scheduling?
237. Concepts of process scheduling, situations of process scheduling.
238. Rules of process scheduling.
239. Thread states.
240. Seven types of process scheduling algorithms.
241. Process scheduling algorithms.
242. What is context switching?
243. What is deadlock? Necessary conditions? How to resolve it?
244. Deadlock prevention and avoidance.
245. Ostrich strategy.
246. Banker’s algorithm.
247. Differences between orphan processes and zombie processes? How to avoid these two types of processes? Daemon processes?
248. What is a daemon process? How to implement it?
249. Recursive locks?
250. Kernel mode and user mode.
251. Principles of conversion from user mode to kernel mode?
252. Implementation and role of interrupts, process of interrupt implementation?
253. What is a system interrupt, and the difference between user mode and kernel mode?
254. CPU interrupts.
255. Process that occurs when executing a system call, the more detailed the better.
256. Differences between function calls and system calls?
257. Classic synchronization problems solutions: producer-consumer problem, dining philosophers problem, readers-writers problem.
258. Why were segmentation and paging technologies developed?
259. Segmented storage.
260. Paged storage.
261. Differences between paged storage and segmented storage.
262. Segmented paged storage.
263. Differences between pages and segments?
264. Virtual memory? Advantages of using virtual memory? What is virtual address space?
265. Relationship between virtual memory and physical memory in operating systems.
266. MMU address translation process.
267. Page faults.
268. Common I/O models, five? Asynchronous I/O application scenarios? What are the drawbacks?
269. Three mechanisms of I/O multiplexing: Select, Poll, Epoll.
270. Principles of I/O multiplexing? Zero-copy? Three functions? Understanding the LT and ET modes of epoll.
271. Summary of differences between select, poll, and epoll:
272. Connection limits of select, poll, and epoll three commonly used I/O multiplexing methods.
273. Understanding the LT and ET modes of epoll:
274. How does Linux avoid memory fragmentation?
275. What is the principle of recursion? How to solve stack overflow encountered in recursion?
276. Is ++i an atomic operation?
277. Page fault, page table addressing.
278. Implementation of LRU.
279. Memory partitioning.
280. Dynamic partition allocation algorithms in operating systems.
281. Buddy system related.
282. I/O control methods.
283. Spooling technology.
284. Channel technology.
285. Implementation of shared memory.
286. Design a thread pool, memory pool.
287. Basic functions of operating systems.
288. What happens if epoll reads halfway and new events arrive?
289. Differences between blocking sockets and non-blocking sockets?
290. Blocking and non-blocking in ET and LT modes?
5. Linux
291. Organizational form of Linux file systems.
292. Linux file systems.
293. Virtual File System (VFS) in Linux.
294. Inode nodes.
295. Relationship between bootloader, kernel, and root file.
296. What is a hard disk?
297. Linux soft links, hard links, if the source file of the soft link is deleted, can the soft link still be used?
298. Two phases of bootloader startup.
299. How is the memory space of Linux system applications allocated, how large is the user space, and how large is the kernel space?
300. How is shared memory implemented in Linux?
301. Detailed principles and usage of Linux library function mmap().
302. Valgrind tool in Linux.
303. Three commands for file processing: grep, awk, sed that must be known.
304. Commands to query process CPU usage.
305. Complete process of a program from start to finish.
306. Generally, what is the size of stack space on Linux/Windows platforms?
307. Linux redirection.
308. Why use command output redirection?
309. Differences between three I/O multiplexing models.
310. Common Linux commands.
311. Linux command manual.
312. Usage of chmod.
6. Computer Networks
313. TCP flow completed by socket.
314. Physical layer.
315. Data link layer.
316. Network layer.
317. What is the function of a router?
318. How is an IP packet delivered from bottom to top?
319. What is the use of an IP address, and why do we need both IP addresses and MAC addresses?
320. Function of ARP protocol.
321. What is ARP protocol? Why do we need both IP and MAC addresses?
322. Principle of NAT, how to distinguish between different IP packets during communication between external networks and internal networks or between internal networks?
323. RIP routing protocol.
324. Why use IP addresses for communication?
325. What is the use of subnet masks?
326. Methods of subnetting.
327. Reasons for layered TCP/IP protocol stack.
328. Why does congestion control occur?
7. Transport Layer
329. Functions of the transport layer.
330. Detailed explanation of TCP and UDP.
331. UDP and TCP packet formats.
332. Analysis of UDP and TCP data packet formats and lengths.
333. TCP flag bits.
334. How many timers does TCP protocol have?
335. Content of TCP protocol’s three-way handshake and four-way handshake? 13 states.
336. Content of the three-way handshake.
337. Why randomly generate sequence numbers instead of starting from 0?
338. Why must a three-way handshake be performed?
339. What is the essence of TCP being connection-oriented? Is it really a connection?
340. Differences between TIME_WAIT and CLOSE_WAIT.
341. Under what circumstances does a connection enter the CLOSE_WAIT state?
342. What is the duration of the time_wait state? Why is there a time_wait state?
343. Why is the duration of time_wait 2MSL?
344. Why does the fourth wave of TCP wait for 2MSL?
345. What happens if a connection is established but the client suddenly fails?
346. Differences and application scenarios between TCP and UDP.
347. Why is UDP sometimes more advantageous than TCP? (TCP packet loss transmission speed is slow).
348. What is the maximum size of a packet in UDP?
349. TCP packet sticking.
350. Guarantee of TCP reliability.
351. Congestion control.
352. TCP flow control.
353. Differences between flow control and congestion control?
354. Explain the process of ping, what protocols are used?
355. Socket programming.
356. Why does the client not need to bind?
357. Disadvantages of send and recv.
358. Why does TCP need one more wave than handshake?
359. Application layer.
360. Common network protocols?
361. Differences between IPv4 and IPv6 protocols.
362. Network devices at each layer of network protocols?
363. Describe the entire process after entering an address in a browser, and the corresponding processes at each level.
364. What is HTTP?
365. Working methods of http and https.
366. Differences between http and https.
367. HTTP status codes.
368. Differences between HTTP1.0 and HTTP1.1?
369. Process of https.
370. Differences between GET and POST in HTTP.
371. Compare GET and POST.
372. HTTP request message format.
373. HTTP response message format.
374. Names and functions of each layer in the OSI 7-layer network model?
375. Names and functions of the TCP/IP 4-layer network model?
376. Common protocols and their functions at each layer in the OSI 7-layer network?
377. Differences between OSI and TCP models?
378. What is DNS for?
379. Describe what happens after the network card receives data.
380. Under what circumstances does the send function block?
381. Common port numbers.
8. Data Structures
382. Common search algorithms? Specific implementations.
383. Common sorting algorithms? Specific implementations, which are stable, time complexity, space complexity, how to implement quick sort non-recursively? Advantages of quick sort?
384. Common algorithms for graphs?
385. Huffman coding?
386. Differences between AVL trees, B+ trees, red-black trees, and B trees? Where is B+ tree applied?
387. B+ trees and B- trees.
388. What is the use of the bidirectional ordered linked list in B+ trees?
389. Why use red-black trees, and when to use AVL trees? What are the advantages of red-black trees over AVL trees?
390. Why is the database index engine B+ tree, and what is the difference from B tree? Why not use red-black trees/AVL trees?
391. Database index is B+ tree.
392. How to determine if a single linked list has a loop?
393. How to determine if a graph is connected?
394. Where is hash used, several methods to resolve hash collisions? Load factor?
395. Number of different constructions of a binary tree with n nodes.
396. Relationship between node degrees in a binary tree.
397. Lowest common ancestor of binary trees, lowest common ancestor of binary search trees.
398. Maximum distance between nodes.
399. Convert a binary tree in place into a doubly linked list.
400. All paths in a binary tree.
401. Find the maximum value in each level of a binary tree?
402. Maximum depth, minimum depth, whether it is a balanced tree.
403. Number of leaf nodes in a binary tree.
404. Swap left and right children, mirror of binary tree.
405. Are two binary trees equal?
406. Is it a complete binary tree?
407. Is it a symmetric binary tree?
408. Determine if B is a subtree of A.
409. Construct Huffman tree.
410. Handwrite single linked list reversal? Delete a specified node of a single linked list.
411. Implement a circular queue.
412. Top K problem.
413. Find the maximum distance of a tree.
414. KMP algorithm.
415. Differences between arrays and linked lists?
416. Thought process for reverse pairs.
417. Merge 100 sorted arrays.
418. Use recursion and non-recursion to find the depth of a binary tree.
419. Advantages and disadvantages of indexes and linked lists?
420. Find all points contained in a circle centered at a point.
421. Understanding of trie.
422. Optimization of quick sort.
423. Principles of bitmap usage for massive data.
424. Properties and rotation rules of red-black trees.
425. Properties of balanced binary trees (AVL).
426. Differences between red-black trees and balanced binary trees:
9. Algorithms
427. Pre-order, in-order, post-order traversal of binary trees.
428. Reconstruct a binary tree based on pre-order and in-order traversal.
429. Dynamic programming, longest common subsequence.
430. Divide and conquer vs recursion.
431. Greedy algorithm, knapsack problem.
432. BFS, DFS, Dijkstra’s algorithm, Floyd’s algorithm.
433. Dynamic programming for palindrome strings?
434. Sorting algorithms? Time complexity? Stability of algorithms?
435. Quick methods for problems like stack in and out order.
436. String matching?
437. Find the square root of a number (binary search).
10. Databases
438. What is a transaction?
439. Distributed transactions.
440. First, second, and third normal forms.
441. Types of database indexes, role of database indexes.
442. Differences between clustered and non-clustered indexes.
443. Differences between unique indexes and primary key indexes.
444. Database engines, characteristics and differences between InnoDB and MyISAM.
445. Differences between relational and non-relational databases.
446. Isolation levels of databases.
447. Role of database connection pools.
448. Types of data locks, methods of locking.
449. Differences between union and join in databases.
450. Differences between inner join, left outer join, and right outer join.
11. Design Patterns
451. Singleton pattern.
452. Handwritten thread-safe singleton pattern?
453. Factory pattern.
454. Decorator pattern.
455. Publish/Subscribe pattern.
456. Observer pattern.
457. MVC pattern.
12. Multithreading Programming
13. Common Intelligence Questions in Interviews
458. Binary problems.
459. Gold bar problem.
460. Mouse and poison problem.
461. Bucket problem.
462. Water pouring problem 1.
463. Water pouring problem 2.
464. Wine scooping problem.
465. Money problem.
466. Earning money problem.
467. Fake money problem.
468. Coin problem.
469. Hotel problem.
470. Blue eyes problem.
471. Blue-eye problem.
472. Crazy dog problem (same as blue eyes).
473. Slap problem (same as blue eyes).
474. Time problem.
475. Candle burning problem.
476. Weight problem.
477. Ping pong ball weight.
478. Salt weight problem.
479. Pill problem.
480. Pill problem 2.
481. Math problem.
482. Probability problem 1.
483. Probability problem 2.
484. Poker problem.
485. Egg throwing problem.
486. Filling numbers.
487. Patterns.
488. Guess number problem.
489. Other problems.
490. Fruit label problem.
491. Penny label problem (same as fruit label).
492. Medicine problem.
493. Coin problem.
494. Light tube problem.
495. Blind person problem.
496. Maximum diamond problem.
14. HR Questions
15. Communication Related
497. OFDM and 802.11a protocol.
498. OFDM.
499. Fading.
500. ICI and ISI:
501. Communication synchronization.
502. PAPR.
503. Why does OFDM have a relatively large out-of-band power?