In the field of self-driving technology, DonkeyCar, as an open-source platform based on Python, allows enthusiasts to build their own self-driving cars at a low cost. This project not only helps beginners understand the basic principles of autonomous driving but can also serve as a practical platform for artificial intelligence and machine learning. This article will guide you through the DonkeyCar project and explore how to use Python to build a simple self-driving system.
## 1. Introduction to DonkeyCar
DonkeyCar is a modular self-driving platform that uses Python as the primary development language, combined with hardware like Raspberry Pi and Arduino, allowing ordinary remote-controlled cars to transform into self-driving vehicles. The main features of this platform include:
- Completely open-source, with an active community
- Based on Python, easy to learn and expand
- Low hardware costs, low entry barrier
- Supports deep learning frameworks (TensorFlow/Keras)
- Modular design for easy customization
## 2. System Components
### 2.1 Hardware Components
- RC car chassis
- Raspberry Pi (or Jetson Nano)
- Camera
- Motor driver board
- Battery pack
- Other accessories (screws, brackets, etc.)
### 2.2 Software Components
```python
# Example of the core code structure of DonkeyCar
from donkeycar import Vehicle
from donkeycar.parts.camera import PiCamera
from donkeycar.parts.controller import get_js_controller
from donkeycar.parts.actuator import PCA9685
def init_car():
car = Vehicle()
# Add camera component
cam = PiCamera()
car.add(cam, outputs=['camera'])
# Add controller
ctr = get_js_controller()
car.add(ctr,
inputs=['camera'],
outputs=['steering', 'throttle'])
# Add actuators
steering_controller = PCA9685(channel=0)
throttle_controller = PCA9685(channel=1)
car.add(steering_controller,
inputs=['steering'])
car.add(throttle_controller,
inputs=['throttle'])
return car
3. Basic Workflow
-
Data Collection: Manually drive the car to collect training data. -
Data Processing: Clean and preprocess the collected data. -
Model Training: Use deep learning to train the driving model. -
Autonomous Driving: Deploy the model to achieve autonomous driving.
3.1 Data Collection Example
# Example of data collection code
from donkeycar.parts.datastore import TubWriter
def collect_training_data(car):
# Initialize data recorder
inputs = ['camera', 'steering', 'throttle']
types = ['image_array', 'float', 'float']
recorder = TubWriter(path='./data',
inputs=inputs,
types=types)
car.add(recorder, inputs=inputs)
# Start data collection
car.start()
3.2 Model Training Example
# Example of model training code
from donkeycar.parts.keras import KerasLinear
def train_model():
model = KerasLinear(input_shape=(120, 160, 3))
# Compile model
model.compile(optimizer='adam',
loss='mse',
metrics=['accuracy'])
# Train model
model.fit(train_data, train_labels,
epochs=10,
validation_split=0.2)
return model
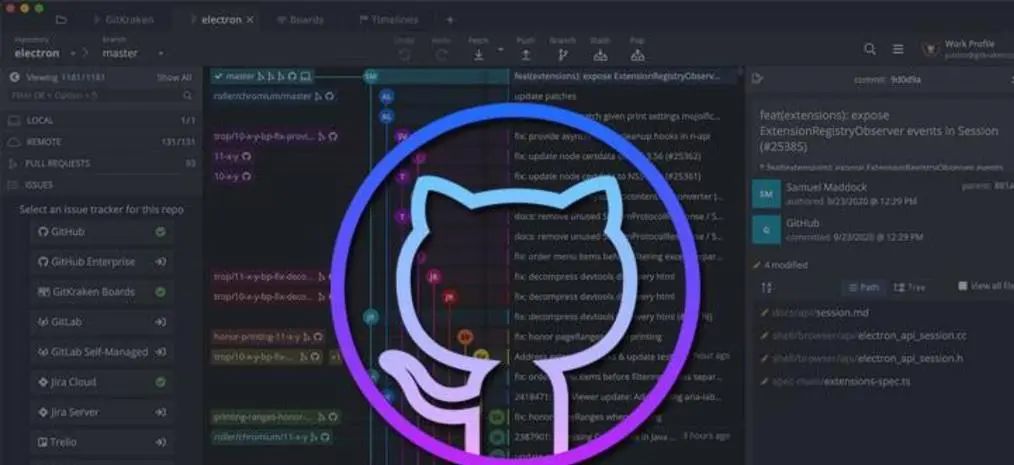
4. Practical Tips
-
Data Quality is Important
-
Ensure training data covers various scenarios. -
Data should be sufficiently diverse. -
Avoid collecting duplicate data.
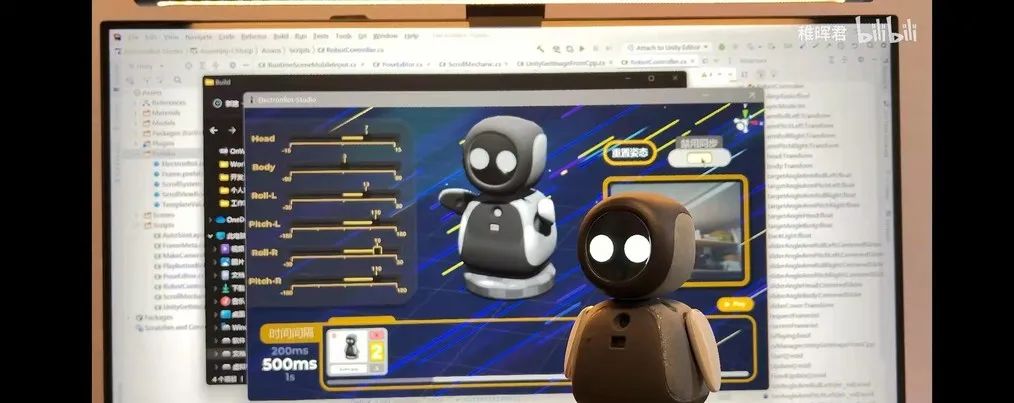
-
Use the tub
tool to view collected data. -
Commonly used donkey calibrate
command to calibrate the servo. -
You can view the status of the car in real-time through the web interface.
5. Common Problem Solutions
-
Inaccurate Steering
# Adjust steering parameters
STEERING_LEFT_PWM = 460 # Adjust these values appropriately
STEERING_RIGHT_PWM = 290
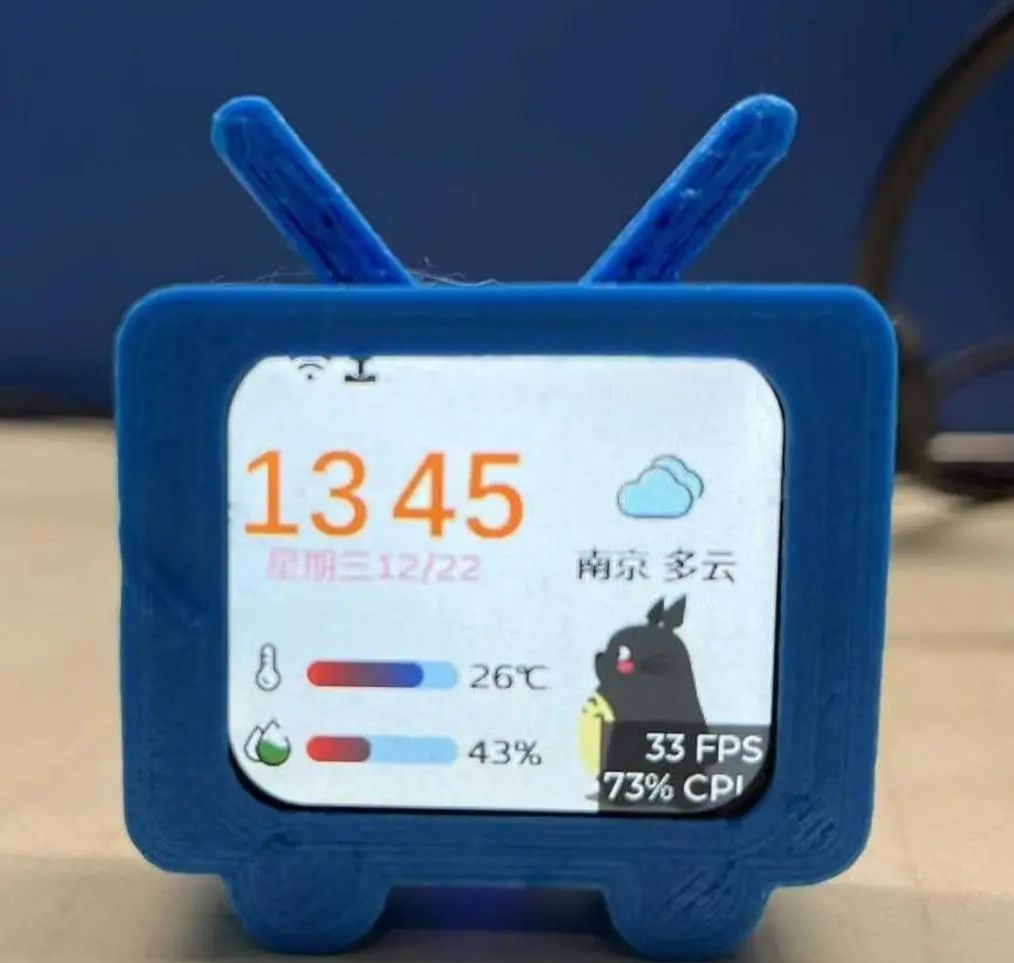
-
Speed Control
# Limit maximum speed
MAX_THROTTLE = 0.3 # It is recommended for beginners to start with small values
MIN_THROTTLE = 0.1
Friends, that’s all for today’s introduction to DonkeyCar! This project is perfect for Python enthusiasts who want to get started with autonomous driving. Remember to pay attention to safety during practice and find an open space for testing. Feel free to discuss any questions in the comments section. Have fun and happy learning!
Additional links:
-
Official Documentation: docs.donkeycar.com -
GitHub Repository: github.com/autorope/donkeycar
Would you like me to explain or break down the code samples?