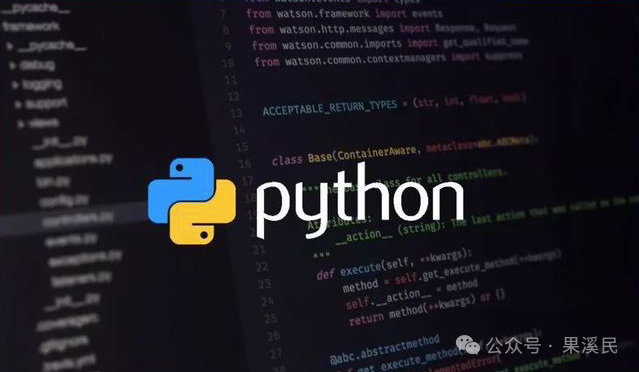
Game Introduction
The Knife Challenge is a classic arcade game where players control a knife, aiming and throwing it at a rotating target (usually a wooden board) to hit the bullseye. The core of the game is simple yet challenging: the target keeps rotating, and players need to throw the knife at the right moment.
This tutorial will guide you through creating a simple Knife Challenge game using Python, helping you learn how to use the Pygame library for developing mini-games. Pygame is a widely used Python game development library suitable for creating 2D games.
Requirements
-
Python 3.x -
Pygame library (install command: <span>pip install pygame</span>
)
Game Rules
-
Players control the number of knives; each throw reduces the number of knives by one. -
There is a rotating wooden target in the game scene, and the goal is to hit the bullseye. -
Each time a knife hits the bullseye, the player scores, and the target’s speed increases. -
If the knife misses the target, the game ends.
Code Implementation
import pygame
import random
import math
# Initialize pygame
pygame.init()
# Game window size
screen_width = 600
screen_height = 400
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption('Knife Challenge')
# Colors
WHITE = (255, 255, 255)
RED = (255, 0, 0)
BLACK = (0, 0, 0)
# Game parameters
clock = pygame.time.Clock()
font = pygame.font.SysFont('Arial', 24)
game_over = False
score = 0
knife_count = 5
# Knife class
class Knife(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = pygame.Surface((10, 30))
self.image.fill(RED)
self.rect = self.image.get_rect()
self.rect.center = (x, y)
self.angle = 0
self.speed = 5
def update(self):
self.rect.centerx += self.speed * math.cos(math.radians(self.angle))
self.rect.centery += self.speed * math.sin(math.radians(self.angle))
if self.rect.centerx < 0 or self.rect.centerx > screen_width or self.rect.centery < 0 or self.rect.centery > screen_height:
self.kill()
# Target class
class Target(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.Surface((50, 50))
pygame.draw.circle(self.image, WHITE, (25, 25), 25, 5)
pygame.draw.circle(self.image, RED, (25, 25), 15)
self.rect = self.image.get_rect()
self.rect.center = (screen_width // 2, screen_height // 2)
self.angle = 0
def update(self):
self.angle += 3
self.image = pygame.Surface((50, 50))
pygame.draw.circle(self.image, WHITE, (25, 25), 25, 5)
pygame.draw.circle(self.image, RED, (25, 25), 15)
self.image = pygame.transform.rotate(self.image, self.angle)
self.rect = self.image.get_rect()
self.rect.center = (screen_width // 2, screen_height // 2)
# Main game loop
def game_loop():
global game_over, score, knife_count
knives = pygame.sprite.Group()
targets = pygame.sprite.Group()
# Create target
target = Target()
targets.add(target)
# Main game loop
while not game_over:
screen.fill(BLACK)
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.MOUSEBUTTONDOWN and knife_count > 0:
mouse_x, mouse_y = pygame.mouse.get_pos()
# Calculate knife angle
angle = math.degrees(math.atan2(mouse_y - screen_height // 2, mouse_x - screen_width // 2))
knife = Knife(screen_width // 2, screen_height // 2)
knife.angle = angle
knives.add(knife)
knife_count -= 1
# Update game objects
knives.update()
targets.update()
# Check for knife and target collisions
for knife in knives:
if knife.rect.colliderect(target.rect):
score += 1
knife.kill()
knife_count += 1 # Restore one knife each time the bullseye is hit
if score % 5 == 0: # Speed up the target every 5 hits
target.angle += 2
# Draw target and knives
targets.draw(screen)
knives.draw(screen)
# Display score and remaining knives
score_text = font.render(f'Score: {score}', True, WHITE)
knife_count_text = font.render(f'Remaining Knives: {knife_count}', True, WHITE)
screen.blit(score_text, (10, 10))
screen.blit(knife_count_text, (screen_width - 200, 10))
if knife_count == 0:
game_over_text = font.render('Game Over!', True, RED)
screen.blit(game_over_text, (screen_width // 2 - 100, screen_height // 2))
pygame.display.flip()
# Control frame rate
clock.tick(60)
# Start the game
game_loop()
# Exit the game
pygame.quit()
Code Analysis
-
Initialization and Setup
-
Use <span>pygame.init()</span>
to initialize Pygame. -
Create a window and set the title.
Knife Class
-
<span>Knife</span>
class represents the behavior of the knife, including its position, speed, and direction of movement. The knife moves along a specified angle during each update until it goes off-screen.
Target Class
-
<span>Target</span>
class represents the rotating target. The target rotates 3 degrees during each update.
Main Game Loop
-
The game loop handles events (such as mouse clicks), creates knives, and calculates the angle of knife throws. -
Update the positions of knives and the target, and check if the knife hits the bullseye. -
Display the score and the number of remaining knives. If a knife hits the bullseye, increase the score and restore one knife.
Game Over
-
If the remaining number of knives is 0, the game ends and displays “Game Over!”.
Conclusion
This simple Knife Challenge game is implemented using Python and Pygame, covering basic game development skills such as graphic rendering, event handling, and collision detection. By extending this framework, you can add more features like different levels, more types of targets, player ability upgrades, and more.