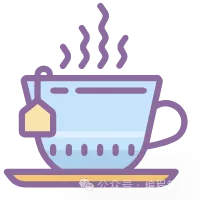
Project Name: How to Easily Adjust Video Resolution to 720p Using Python
Development Environment:PyCharm 2023.3.4 + python3.7
Libraries Used:PIL, os
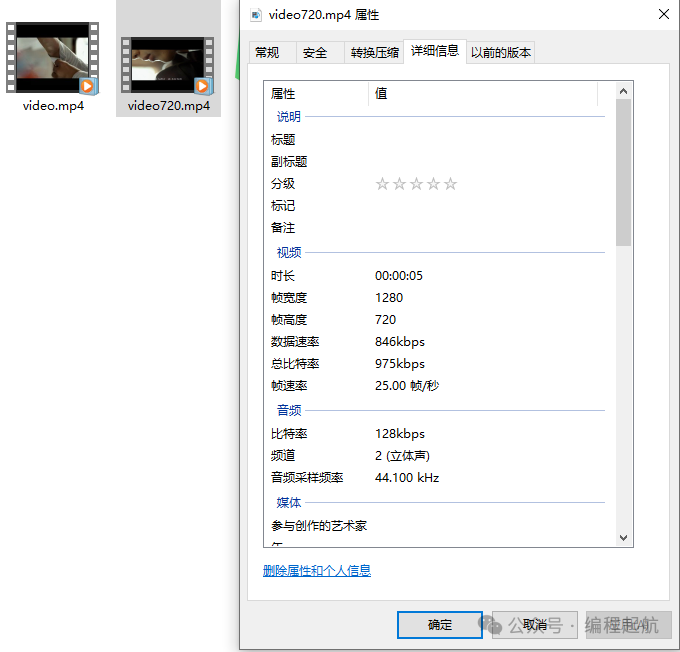
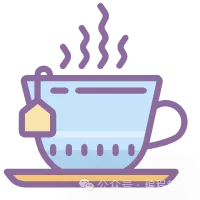
Implementation Process
from moviepy.editor import VideoFileClip
import os
2. Define<tspan><span>convert_video_resolution</span></tspan>
function to convert the resolution of the video file to the specified new resolution
def convert_video_resolution(input_path, output_path, new_resolution=(1280, 720)):
"""
Convert the resolution of the video file to the specified new resolution.
:param input_path: The path of the input video file.
:param output_path: The path where the output video file will be saved.
:param new_resolution: A tuple containing the new width and height to set the new resolution of the video, default is 720p (1280x720).
"""
# Check if the input file exists
if not os.path.isfile(input_path):
raise FileNotFoundError(f"Input file {input_path} not found.")
try:
# Load the video clip using a context manager to automatically release resources
with VideoFileClip(input_path) as clip:
# Resize the video to the new resolution
resized_clip = clip.resize(new_resolution)
# Set parameters for writing the video file:
# codec='libx264' - use H.264 encoding, a widely supported video compression format
# audio_codec='aac' - use AAC audio encoding, providing good audio quality and compatibility
# preset='medium' - balance between encoding speed and compression ratio
# threads=4 - number of parallel processing threads, can be adjusted according to CPU core count
# bitrate - optional parameter, defines the output video bitrate (e.g., '5000k'), adjust as needed to control file size and quality
write_params = {
"codec": "libx264",
"audio_codec": "aac",
"preset": "medium",
"threads": 4,
# "bitrate": "5000k", # Uncomment and adjust bitrate as needed
}
# Write the new video file
resized_clip.write_videofile(output_path, **write_params)
except Exception as e:
# Capture any errors that occur and print the error message
print(f"An error occurred while processing the video: {e}")
raise # Raise the exception so the caller knows an error occurred
3. Call the Function
# Video source file path
video_path = './video.mp4'
# Video target file path
output_path = './video720.mp4'
# Call the function to convert video resolution
convert_video_resolution(video_path, output_path)
4. Conclusion
The above is all the content of this sharing. If you have any questions or want to share your automation office experience, our comment section is always open for you. Your every like and share is our greatest support and encouragement!
Want to learn more and get the complete code from this article? It’s simple, follow our official account and reply with the article title to get it immediately.Thank you again for reading, and I look forward to seeing you in the next sharing!
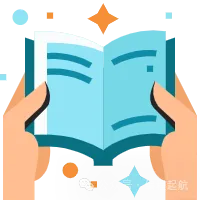
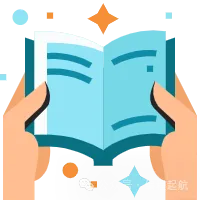
Python Automation Series: Convert PDF Pages to Images
Python Automation Series: Rearrange the Order of PDF Pages
Python Automation Series: Crop Specific Areas of Specific Pages in a PDF
Python Automation Series: Automatically Fill Student Information from Excel into a Word Document
Python Automation Series: Easily Merge Specific Pages of PDF Files