Introduction
Currently, there is a task requirement for the shopping cart, which involves image recognition and motion control. Therefore, it is necessary to initialize two processes to complete the respective actions. Since motion control requires the results of image recognition, we now need to implement the collaboration between the two processes using Python syntax. This blog combines an actual Python program to achieve process interaction through queues.
Program Analysis
First, let’s introduce the libraries we need:
import time
from multiprocessing import Process, Queue
from multiprocessing import Process, Queue is the main library, which provides queue and process operation functions. In this test, we use the following process and queue operation functions:
q1.get() # Get content from q1 queue
q1.put("q1 put things1") # Add content to q1 queue
p1 = Process(target=test1, args=(q1, q2)) # Initialize p1 process
p1.start() # Start p1 process
p1.join() # Join p1 for system scheduling
q1.qsize() # Check the size of q1 queue
The correspondence between threads and processes in the test:
q1 queue -> q1 process
q2 queue -> q2 process
With the above basic functions, we can look at our program implementation. It can be seen that the program first initializes the processes, starts them, and joins them for system scheduling. After running these statements, we can consider that the p1 and p2 processes are initialized.
p1 = Process(target=test1, args=(q1, q2))
p2 = Process(target=test2, args=(q1, q2))
p1.start()
p2.start()
p1.join()
p2.join()
After initializing the processes, we can look at the main test function, starting with test1.
def test1(q1, q2):
q1.put("first data")
while(1):
s = q2.get()
print('q2 left + ' + str(q2.qsize()))
print('q1 get + ' + s)
q1.put("q1 put things1")
q1.put("q1 put things2\n")
time.sleep(1)
In the previous initialization function, we first initialized the p1 process, which means that test1 will run first. Therefore, to ensure that there is content in the queue at the beginning, I added the content “first data” to the q1 queue at the very start of the function. To ensure continuous testing, I set the test1 process to an infinite loop. In test1, it first retrieves the content from the q2 queue and prints the size of the q2 queue, then puts new content into the q1 queue. Note that two data nodes are added here, namely “q1 put things1” and “q1 put things2\n”.
I raise a question: when the p1 process retrieves content from the q2 queue, does it retrieve all the content from the q2 queue or just the first piece of data?
Next, let’s explore this question. Now let’s see what the test2 function does:
def test2(q1, q2):
while(1):
s = q1.get()
print('q1 left + ' + str(q1.qsize()))
print('q2 get + ' + s)
q2.put("q2 put data1")
time.sleep(1)
The test2 function does relatively less, which is to first retrieve data from the q1 queue and print the remaining size of the q1 queue, then add “q2 put data1” to the q2 queue.
Result Analysis
Let’s look at the calls in the main function:
if __name__ == '__main__':
p1 = Process(target=test1, args=(q1, q2))
p2 = Process(target=test2, args=(q1, q2))
p1.start()
p2.start()
p1.join()
p2.join()
The main function only performs thread startup operations. Now let’s look at the output results:
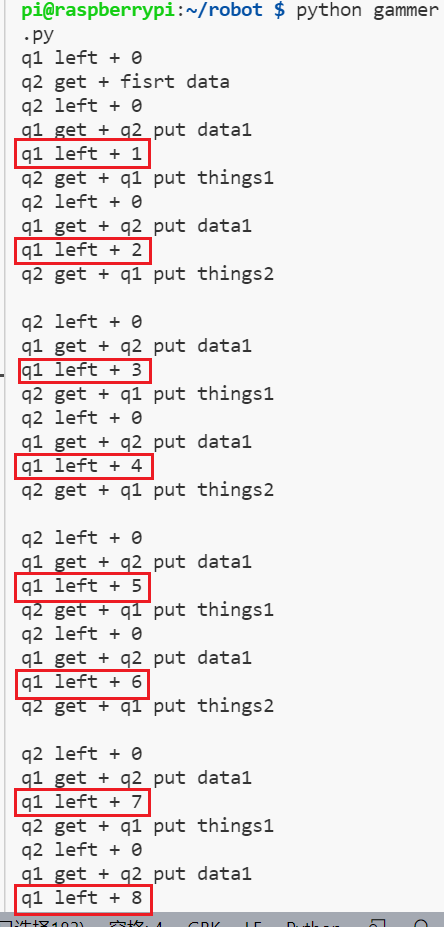
From the results, we can clearly see that the q1 thread adds two nodes of data each time, and the p2 process performs the q1.get() operation from the q1 thread. With the increasing number of loops, we can find that the length of the q1 queue keeps increasing. Thus, we can conclude that when the p1 process retrieves content from the q2 queue, it retrieves only the first piece of data from the q2 queue.
Moreover, from the results, we can also analyze the waiting issue in process scheduling. Logically, since the p1 process where test1 is located is initialized first, test1 should be scheduled first. Therefore, the print(‘q2 left + ‘ + str(q2.qsize())) should be the first queue operation statement to be called, but in reality, it is not. Why?
This is because before the statement print(‘q2 left + ‘ + str(q2.qsize())) in the p1 process’s test1 function, the statement s = q2.get() is executed first. However, at the beginning, the q2 queue is empty, so the program enters a waiting state until the p2 process puts data into the q2 queue, allowing the p1 process to obtain the data from the q2 queue and continue executing the test1 program.

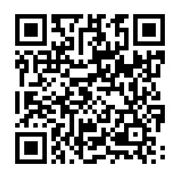
(Scan the QR code for course details)
Click “Read Original” to view course details