Porting Introduction
For embedded devices, due to the large differences in chip models and peripherals, and limited resources, IoT operating systems cannot adapt and integrate all drivers like Windows/Linux. Therefore, typically, only part of the chips/development boards are adapted first. To run the operating system on other chips/development boards, porting is necessary.
Hardware Information
Porting Preparation
This porting used the Raspberry Pi 3 Model B development board, USB to TTL module, SD card and card reader.
This porting requires setting up the Linux development environment (make, arm-none-eabi toolchain) according to the LiteOS tutorial on Gitee. The environment setup tutorial can be found at: https://gitee.com/LiteOS/LiteOS/blob/master/doc/LiteOS_Build_and_IDE.md.
This porting requires downloading the official Raspberry Pi imaging tool (Raspberry Pi Imager),
Download link: https://www.raspberrypi.org/software/.
Porting Steps
Directory/File | Description |
Inc | Header files for chip peripheral configuration |
include | System-related configuration header files |
os_adapt | Adaptation interface files |
Src | Source files for chip peripheral configuration |
liteos.ld | Link file for the current development board project |
Makefile | Makefile for the current development board project |
los_startup_gcc.S | Chip startup file |
In los_startup_gcc.S, set the 13th bit of the System Control Register to 0, indicating that the base address of the exception vector table starts from 0. The code is as follows:
/* set vector base 0x00000000 */ mrc p15, 0, r0, c1, c0, 0 bic r0, #(1 << 13) mcr p15, 0, r0, c1, c0, 0
[13] | V |
V Vectors bit. This bit selects the base address of the exception vectors: 0:Normal exception vectors, base address 0x00000000. Software can remap this base address using the VBAR. 1:High exception vectors, base address 0xFFFF0000. This base address is never remapped. The primary input VINITHI defines the reset value of the V bit. |
-
Set L1 Page Table Entries
Add the following code to main.c to set L1 page table entries (this MMU only involves L1 page table), mapping 4G virtual memory linearly to physical addresses.
VOID MmuSectionInit(VOID){ UINT32 ttbBase = (UINTPTR)g_firstPageTable; /* First clear all TT entries - ie Set them to Faulting */ (VOID)memset_s((VOID *)(UINTPTR)ttbBase, MMU_16K, 0, MMU_16K); /* Set all mem 4G as uncacheable & rw first */ X_MMU_SECTION(0, 0, (MMU_4G >> SHIFT_1M), UNCACHEABLE, UNBUFFERABLE, ACCESS_RW, NON_EXECUTABLE, D_CLIENT); X_MMU_SECTION(0, 0, ((AUX_BASE - 1) >> SHIFT_1M), CACHEABLE, BUFFERABLE, ACCESS_RW, EXECUTABLE, D_CLIENT);}
(Swipe left to see more)
-
Add the following code in los_startup_gcc.S to enable MMU
1. Invalidate all TLB entries
mcr p15, #0, r0, c8, c7, #0
2. Set access permissions for DOMAIN0 and DOMAIN01
mov r0, #(DOMAIN0 | (DOMAIN1 << 2)) mcr p15, 0, r0, c3, c0
Note:Register C3 in CP15 defines the access permissions for the 16 domains of the ARM processor. (For details, please refer to the relevant documents at the end)
3. Set MMU L1 page table base address
ldr r0, =g_firstPageTable mcr p15, #0, r0, c2, c0, #0
4. Enable MMU and cache
/* enable mmu */ mrc p15, #0, r0, c1, c0, #0 orr r0, r0, #1 mcr p15, #0, r0, c1, c0, #0 /* enable icache */ mrc p15, #0, r0, c1, c0, #0 orr r0, r0, #(1 << ICACHE_BIT) mcr p15, #0, r0, c1, c0, #0 mrc p15, #0, r0, c1, c0, #0 orr r0, r0, #(1 << DCACHE_BIT) mcr p15, #0, r0, c1, c0, #0
-
Add the following code in los_startup_gcc.S:
mrc p15, 0, r11, c0, c0, 5 and r11, r11, #MPIDR_CPUID_MASK
[1:0] | CPU ID |
Indicates the processor number in the Cortex-A7 MPCore processor. For: • One processor, the CPU ID is 0x0. • Two processors, the CPU IDs are 0x0 and 0x1. • Three processors, the CPU IDs are 0x0, 0x1, and 0x2. • Four processors, the CPU IDs are 0x0, 0x1, 0x2, and 0x3. |
cmp r11, #0 bne excstatck_loop_done
cpu_start: #ifdef LOSCFG_APC_ENABLE bl setup_mmu #ifdef LOSCFG_KERNEL_SMP bl secondary_cpu_start #endif #endif b .
-
Add the following code in main.c:
for (coreId = 1; coreId < LOSCFG_KERNEL_CORE_NUM; coreId++) { /* per cpu 4 mailbox */ mailbox->writeSet[coreId * 4 + MAILBOX3_IRQ - MAILBOX0_IRQ] = (UINT32)reset_vector; asm volatile ("sev");}while (LOS_AtomicRead(&g_cpuNum) < LOSCFG_KERNEL_CORE_NUM) { asm volatile("wfe");}
(Swipe left to see more)
Note:The main core wakes up other cores through the mailbox mechanism and waits for other cores to start.
-
Add IPI interrupt code in drivers/interrupt/arm_control.c. Refer to the mailbox mechanism in the official Raspberry Pi chip manual to implement the HalIrqSendIpi function and HalSmpIrqInit function.
-
Add the following code in targets/kconfig.raspberry:
config LOSCFG_PLATFORM_RASPBERRY32_PI3B bool "Raspberry32_Pi3B" select LOSCFG_ARCH_CORTEX_A53_AARCH32 select LOSCFG_USING_BOARD_LD select LOSCFG_PLATFORM_ARM_CONTROL select LOSCFG_RASPBERRY_PI_SYSTICK
-
Modify the following Makefile:
driver/timer/Makefiledriver/interrupt/Makefiletargets/Raspberry32_Pi3B/Makefile
Use the Raspberry Pi Imager tool to create the Raspberry Pi system.
-
Replace the kernel7.img file generated by the compilation with the kernel7.img file on the SD card.
-
Insert the SD card with the written image file into the Raspberry Pi 3B and power it on, the Raspberry Pi 3B can run the LiteOS system.
The running result is as follows:
********Hello Huawei LiteOS********LiteOS Kernel Version : 5.1.0Processor : Cortex-A53 * 4Run Mode : SMPbuild time : Aug 21 2021 10:15:24**********************************OsAppInitcpu 0 entering schedulercpu 1 entering schedulercpu 2 entering schedulercpu 3 entering schedulerHuawei LiteOS #
Related Document Links
MMU Access Control:https://blog.csdn.net/llf021421/article/details/6330102
MMU Access Control:https://blog.csdn.net/llf021421/article/details/6330102
MMU Cache and Write Buffer:https://blog.csdn.net/fcglx/article/details/87924578
MMU Page Table:https://blog.csdn.net/czg13548930186/article/details/74979222
Official Raspberry Pi Chip Manual:
https://datasheets.raspberrypi.org/bcm2835/bcm2835-peripherals.pdf
Conclusion
https://gitee.com/LiteOS/LiteOS/issues
To make it easier to find the “LiteOS” code repository, it is recommended to visithttps://gitee.com/LiteOS/LiteOS, follow “ Watch”, like “Star”, and “Fork” to your account, as shown in the figure below.
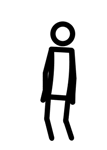
– End –
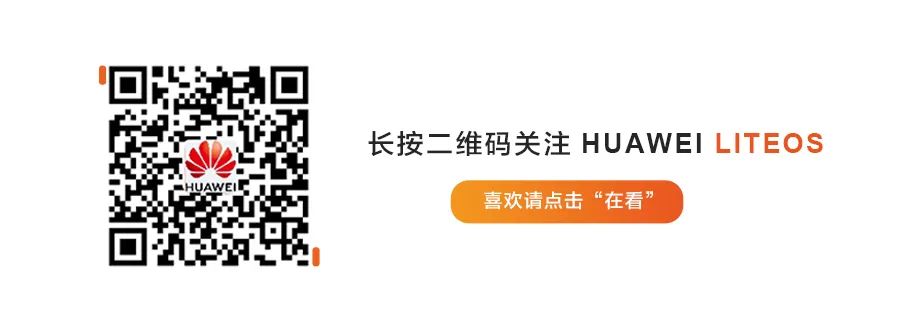