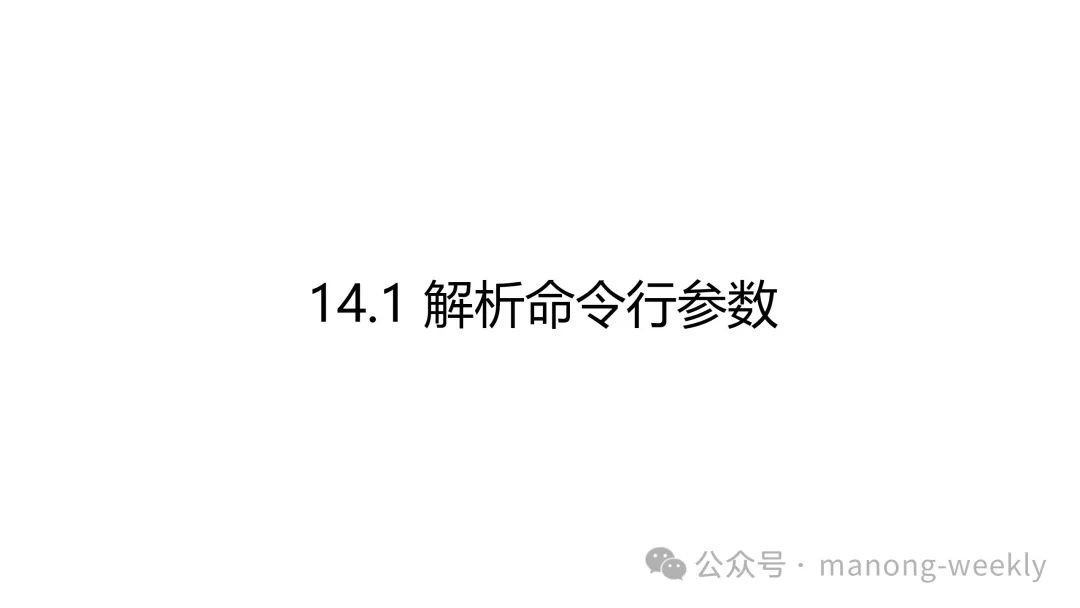
14.1 Parsing Command Line Arguments
Building command line tools (CLI) is a common task in Rust practical projects. Rust provides various ways to parse command line arguments, with the most common approach being the use of third-party libraries like <span>clap</span>
or <span>structopt</span>
. These libraries help us easily define and parse command line arguments, handle user input, and generate corresponding help information.
In this section, we will explore in detail how to use the <span>clap</span>
library to parse command line arguments and implement a basic command line tool.
14.1.1 Using <span>clap</span>
to Parse Command Line Arguments
`clap`[1] is one of the most popular command line argument parsing libraries in the Rust ecosystem. It provides powerful features that allow developers to define command line arguments, subcommands, flags, options, etc., and automatically generate help documentation.
First, include the <span>clap</span>
library in your project. Open <span>Cargo.toml</span>
and add <span>clap</span>
in the <span>[dependencies]</span>
section:
[dependencies]
clap = "4.0"
14.1.2 Creating a Simple Command Line Tool
Let’s start with a simple example by creating a command line tool that accepts a <span>--name</span>
argument and outputs a greeting. We will use <span>clap</span>
to parse the command line arguments and provide help information.
Step 1: Create a Project
cargo new cli_hello
cd cli_hello
Step 2: Modify <span>Cargo.toml</span>
Add the <span>clap</span>
dependency:
[dependencies]
clap = "4.0"
Step 3: Write Code
Open <span>src/main.rs</span>
and add the following content:
use clap::{Arg, Command};
fn main() {
// Use clap to define command line arguments
let matches = Command::new("cli_hello")
.version("1.0")
.author("Rust Developer <[email protected]>")
.about("A simple CLI tool that greets the user")
.arg(
Arg::new("name")
.short('n')
.long("name")
.takes_value(true)
.help("Sets your name")
.required(true),
)
.get_matches();
// Get command line arguments
if let Some(name) = matches.value_of("name") {
println!("Hello, {}!", name);
}
}
In the code above, we used <span>clap::Command</span>
to define the basic information and parameters of the command line tool. Here are explanations of the key parts:
-
<span>Command::new("cli_hello")</span>
: Creates a new command line tool named<span>cli_hello</span>
. -
<span>.version("1.0")</span>
: Sets the version information of the tool. -
<span>.author("Rust Developer <[email protected]>")</span>
: Sets the author information. -
<span>.about("A simple CLI tool that greets the user")</span>
: Sets the description information of the tool. -
<span>Arg::new("name")</span>
: Defines a parameter named<span>name</span>
, where<span>short('n')</span>
indicates it can be accessed via<span>-n</span>
or<span>--name</span>
. -
<span>.takes_value(true)</span>
: Indicates that this parameter requires a value (i.e., the name input by the user). -
<span>.required(true)</span>
: Makes this parameter required; the user must provide it. -
<span>.get_matches()</span>
: Parses the command line arguments.
Step 4: Compile and Run
Run the following command in the terminal to build and run the program:
cargo run -- --name Alice
Output:
Hello, Alice!
If you do not provide the <span>--name</span>
argument, the program will automatically print help information and inform the user that this parameter is required:
Usage: cli_hello [OPTIONS]
A simple CLI tool that greets the user
Options:
-n, --name <NAME> Sets your name
-h, --help Print help information
14.1.3 Parsing Multiple Arguments and Options
You can parse multiple command line arguments and options using <span>clap</span>
. For example, we can add an <span>--age</span>
option to the command line tool and output different greetings based on the user’s provided age.
Modify the Code
In <span>src/main.rs</span>
, modify the code to accept multiple arguments:
use clap::{Arg, Command};
fn main() {
// Define command line tool to support multiple arguments
let matches = Command::new("cli_hello")
.version("1.0")
.author("Rust Developer <[email protected]>")
.about("A simple CLI tool that greets the user")
.arg(
Arg::new("name")
.short('n')
.long("name")
.takes_value(true)
.help("Sets your name")
.required(true),
)
.arg(
Arg::new("age")
.short('a')
.long("age")
.takes_value(true)
.help("Sets your age")
.required(false),
)
.get_matches();
// Get parameters and output corresponding greeting
let name = matches.value_of("name").unwrap();
let age = matches.value_of("age").unwrap_or("unknown");
println!("Hello, {}! You are {} years old.", name, age);
}
In the code above, we added an <span>age</span>
parameter, which is optional (<span>required(false)</span>
), and used the <span>unwrap_or</span>
method to provide a default value of <span>"unknown"</span>
if the user does not input an age.
Step 5: Compile and Run
When running the command, you can choose to provide an <span>age</span>
parameter:
cargo run -- --name Alice --age 30
Output:
Hello, Alice! You are 30 years old.
If the <span>age</span>
parameter is not provided, the default value will be used:
cargo run -- --name Alice
Output:
Hello, Alice! You are unknown years old.
14.1.4 Handling Subcommands
<span>clap</span>
also supports handling subcommands, which is particularly useful for building complex command line tools. For example, you can add subcommands like <span>greet</span>
and <span>bye</span>
to the command line tool, each with different behaviors.
Modify Code to Support Subcommands
use clap::{Arg, Command};
fn main() {
let matches = Command::new("cli_hello")
.version("1.0")
.author("Rust Developer <[email protected]>")
.about("A simple CLI tool that greets or says goodbye")
.subcommand(
Command::new("greet")
.about("Greets the user")
.arg(
Arg::new("name")
.short('n')
.long("name")
.takes_value(true)
.required(true),
),
)
.subcommand(
Command::new("bye")
.about("Says goodbye to the user")
.arg(
Arg::new("name")
.short('n')
.long("name")
.takes_value(true)
.required(true),
),
)
.get_matches();
match matches.subcommand() {
Some(("greet", sub_m)) => {
let name = sub_m.value_of("name").unwrap();
println!("Hello, {}!", name);
}
Some(("bye", sub_m)) => {
let name = sub_m.value_of("name").unwrap();
println!("Goodbye, {}!", name);
}
_ => println!("Please specify a subcommand"),
}
}
Step 6: Compile and Run
You can now choose the <span>greet</span>
or <span>bye</span>
subcommand:
cargo run -- greet --name Alice
Output:
Hello, Alice!
Or:
cargo run -- bye --name Alice
Output:
Goodbye, Alice!
14.1.5 Summary
Through this section, you have learned how to use the <span>clap</span>
library to parse command line arguments and build a feature-rich command line tool. You can add more parameters, options, and subcommands as needed to implement more complex functionalities. Additionally, the automatically generated help information and error handling features of <span>clap</span>
greatly simplify the development process of command line tools.
In actual development, Rust’s strong type system and the features provided by <span>clap</span>
make it easier and more efficient to build effective and reliable command line tools.
References
<span>clap</span>
: https://docs.rs/clap/