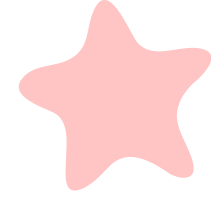
About Conversion Specifiers and Modifiers
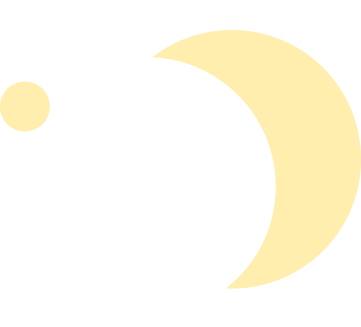
In C/C++ (and many languages that support C-style formatted strings, such as Java and Python in certain contexts), it is often necessary to use formatted output or input functions (like printf, scanf, etc.) to handle different types of data. The “format string” for these functions typically consists of two parts:
Modifiers
Conversion Specifiers
Together, they determine how to convert variable values to strings (for output functions like printf), or how to parse variable values from strings (for input functions like scanf).
Let’s take a look at the role and usage of modifiers and conversion specifiers in format strings.
Conversion Specifiers
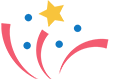
Conversion specifiers indicate the type of data to be output or read. For example:
%d indicates a signed decimal integer (int);
%u indicates an unsigned decimal integer (unsigned int);
%f indicates a floating-point number (float / double, specifics depend on modifiers);
%c indicates a single character (char);
%s indicates a string (char* / const char*);
%x or %X indicates an unsigned hexadecimal integer;
%o indicates an unsigned octal integer;
%p indicates a pointer address (void*);
%e, %E, %g, %G, etc., indicate output of floating-point numbers in scientific notation or more compact forms.
When you see a statement like printf(“%d”, x);, %d is the conversion specifier that tells printf to output the variable x as a decimal integer.
In scanf, if you see scanf(“%d”, &x);, scanf will read an integer from the input stream and assign it to x.
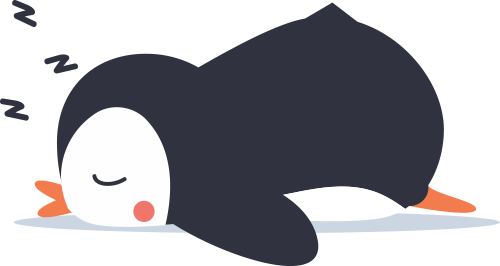
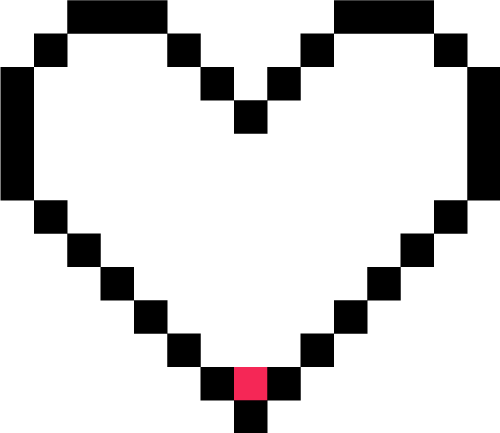
Modifiers
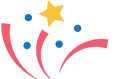
In format strings, modifiers (sometimes called “length modifiers” or “flags/width/precision modifiers”) can further specify:
Length Modifiers;
Flags;
Width and Precision.
They typically follow the % sign and precede the conversion specifier, used to adjust or supplement the behavior of the conversion specifier, specifying how to handle different lengths, precisions, alignments, etc.
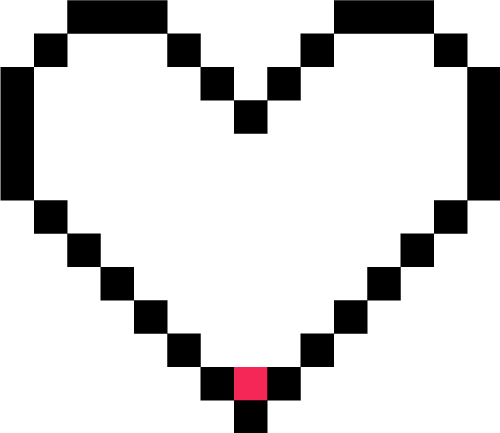
1. Length Modifiers
Common length modifiers include:
①h / hh
h: Treats int in integer conversion specifiers (like %d, %u, %x, etc.) as short int;
hh: Treats int in integer conversion specifiers as signed char / unsigned char.
②l / ll
l: Treats int in integer conversion specifiers as long int; or treats %f conversion specifier as double (using %lf in certain contexts or specific compilers);
ll: Treats int in integer conversion specifiers as long long int.
③L
Generally used with %f, %e, %g, etc., indicating long double type.
Here are a few examples:
printf(“%hd”, x);
Indicates that variable x is treated as short int for output.
printf(“%lld”, y);
Indicates that variable y is treated as long long int for output.
scanf(“%lf”, &fval);
Indicates that input data is read as double into fval (if only %f is written, then under strict C standards it reads as float, but the actual C library usually treats printf(“%f”) as double, while scanf(“%f”) reads as float).
2. Flags
Flags control the format of the output and generally include:
①- (Left Align): Default is right align; for example, %10d places the number on the right side of a width of 10. %-10d will left-align the number.
②+ (Show Sign): For signed numbers (like int, float), always shows the positive or negative sign; for example, printf(“%+d”, 10); outputs +10.
③0 (Zero Fill): For example, %05d ensures the number has a total length of at least 5, filling with 0 if insufficient; for example, outputting 123 will display 00123.
④ (Space): Reserves a space before positive numbers, while negative numbers will show the negative sign.
⑤# (Base or Decimal Point Flag): Adds prefixes 0 or 0x for octal and hexadecimal numbers; for floating-point numbers, it will force the display of the decimal point if there is no fractional part.
Example:
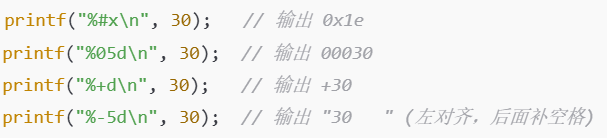
3. Width and Precision
①Width (minimum field width) specifies how many character widths to occupy for output. If the number of characters output is less than the width, it will be filled with spaces or 0 (if there is a 0 flag) in the default direction (right or left alignment).
②Precision has different meanings for different types of conversion specifiers:
Example:
printf(“%8.2f\n”, 3.14159); // Outputs ” 3.14″ (total width of 8 characters, 2 decimal places)
printf(“%.3s\n”, “abcdefg”); // Outputs “abc”
printf(“%5.3d\n”, 12); // Indicates at least 3 digits and total width at least 5, first fills 0 to ensure 3 digits -> “012”, then fills spaces outputting ” 012″
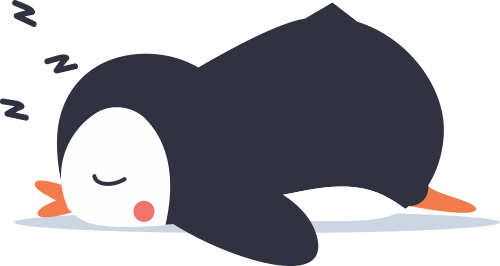
Comprehensive Example
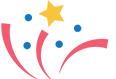
Let’s look at a relatively complex example:
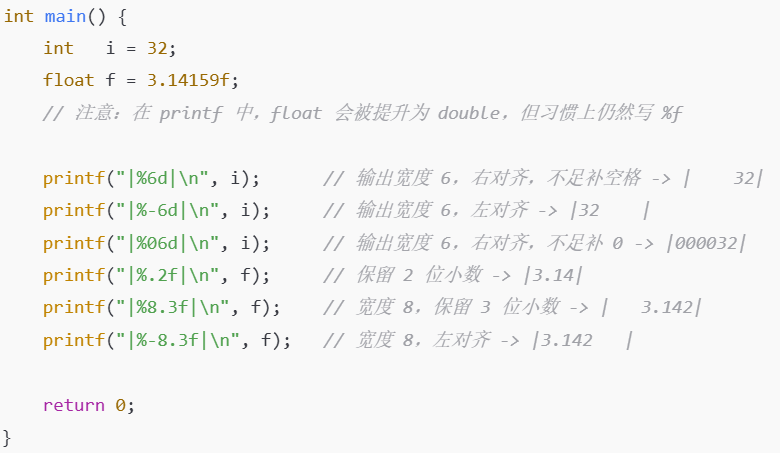
Summary
Conversion Specifiers are key to specifying data types, such as %d, %f, %s, etc.
Modifiers are used to further specify output/input lengths (h, l, ll, etc.), alignment, fill methods, as well as width and precision. Common flags include -, +, 0, (space), #, and can be used in conjunction with width and precision.
Mastering their usage allows for flexible control over the format of program input and output, enhancing code readability and maintainability.
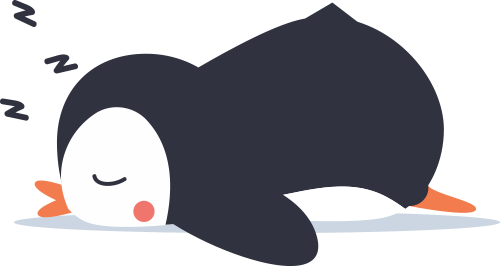
END