In embedded systems, what is the internal mechanism when a function is called, and what steps are executed? As shown in Figure 1, first, let’s look at the memory distribution of a program when it is running.
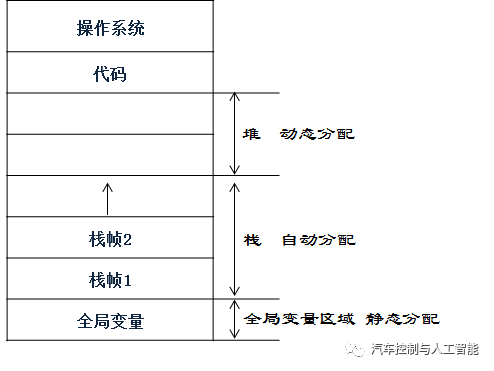
Figure 1 Memory Distribution in the System
When a program runs, its code is loaded into memory and stored in the code section, including the main function and other functions. There are mainly three memory areas used to store data:
The first area is the global variable area, which stores all global variables in the program. Since the number and size of global variables are known, the memory size occupied by this area is determined at the beginning, and they are called static allocation. Variables in this area exist throughout the entire runtime of the program, and this memory area is only released when the entire program finishes running.
The second area is the stack area, which contains all stack frames. A stack frame is a memory area automatically allocated by the system for a function when it is called, used to save its execution context, formal parameters, local variables, and other information. Such a memory area is called a stack frame. Stack frames are allocated when a function is called and are released when the function call ends. Therefore, for a function’s local variables, the system only allocates storage space for the function’s formal parameters and local variables when the function call occurs; after the function call ends, these local variables are released. Additionally, the stack area is automatically allocated by the system, so users do not need to worry about it, which is why it is also called automatic allocation.
The third area is the heap area, which is mainly used for dynamically allocated memory.
Let’s look at an example for clarity.
Figure 2 Example of Memory Distribution
As shown in Figure 2, when the program starts running, the function demudashu() is loaded into memory. Its code is stored in the memory code area. Since a global variable z is defined in this program, a storage unit is allocated for it in the global variable area.
Next, when the system calls the function to run, it allocates a block of memory in the stack, i.e., a stack frame, to store the local variables defined in the function, namely x and y.
Then, the program counter (PC) jumps to the first statement of the function and starts executing.
When the function execution ends, the stack frame it occupies must first be released. For any function call, the corresponding stack frame must be released after the function call ends, so the storage space occupied by the local variables x and y is released.
When a function call occurs, its execution process can be summarized in the following five steps:
-
Allocate a stack frame in the memory stack space to store the function’s formal parameters and local variables.
-
Copy the values of the actual parameters into the corresponding formal parameters.
-
Transfer control flow to the starting position of the function.
-
The function begins execution.
-
After the function finishes executing, the control flow and return value return to the function call point.
Next, let’s summarize the storage and scope of variables with an example.
/* Global variable, fixed address, visible in other source files */ int demu_global_static; /* Static global variable, fixed address, but only visible in this file */ static int demu_static; /* Function parameter: located in stack frame, dynamically created, dynamically released */ int foo(int auto_parameter) { /* Static local variable, fixed address, only visible in this function */ static int func_static; /* Ordinary local variable, located in stack frame, only visible in this function */ int auto_i, auto_a[10]; /* Dynamically allocated memory space, located in heap */ double *auto_d = malloc(sizeof(double) * 2020); return auto_i; }
With the understanding of memory allocation above, let’s take a look at function calls.
① The program first executes the statements before the function call;
② Control flow transfers to the entry point of the called function while passing parameters;
③ Execute the statements in the body of the called function;
④ Control returns to the next instruction of the calling function, bringing back the return value;
Figure 3 Function Call Process
This requires that before transferring to the called function, the address of the instruction being executed at that time must be noted, and the “context must be preserved” (note the relevant information at that time) to facilitate continuing execution after the function call. After the function call, the flow returns to the previously noted address, and based on the noted information, the “context is restored”, and then execution continues. These processes take a certain amount of time. If some functions need to be used frequently, the time spent can be quite long, thereby reducing the execution efficiency of the program. Some utility programs have efficiency requirements, requiring the system’s response time to be short, which necessitates minimizing the time spent in the calling process.
2.1 Built-in Functions
The C language provides a method to improve function call efficiency, which is to embed the called code directly into the calling function at compile time instead of transferring the flow out. These functions embedded in the calling function are called inline functions. Some people refer to them as inline functions.
Usage: Add the keyword inline to the left end of the first line of the function.
Let’s look at an example for clarity.
int main() { int i = 3, j = 5, k = 8, m; m = max(i, j, k); cout << "max=" << m = endl; return 0; }
inline int max(int a, int b, int c); // Define max as an inline function { if (b > a) a = b; if (c > a) a = c; return a; }
Since the function is defined as an inline function, when the compiler encounters the function call “max(i,j,k)”, it replaces it with the code of the max function body, while substituting the actual parameters for the formal parameters. The inline declaration can be written both in the function declaration and definition, or only in one of them, and the effect is the same, allowing it to be treated as an inline function.
Using inline functions can save runtime, but it increases the length of the target program. For instance, if the max function is called 10 times, the compiler will copy and insert the max code into the main function 10 times at compile time, which increases the length of the target file of the main function. Therefore, generally only small-scale functions that are used frequently (such as functions for timed data collection) are declared as inline functions. In cases where the function is very small, the time spent calling the function may be comparable to or even exceed the time taken to execute the function itself, defining it as an inline function can greatly reduce the program’s runtime.
Complex control statements, such as loops and switch statements, cannot be included in inline functions.
Declaring a function as inline is merely a suggestion made by the programmer to the compiler system; it is advisory rather than directive. It does not mean that the compiler must treat it as inline. The decision is made based on specific circumstances. For example, functions that contain loops or switch statements or recursive functions cannot be replaced with inline code, and a function that has thousands of lines is unlikely to be expanded at the call point. In such cases, the compiler will ignore the inline declaration and treat it as a normal function.
Therefore, only small-scale and frequently called simple functions are suitable for being declared as inline functions.
2.2 Function Call Process
As described earlier, as shown in Figure 3, when executing a particular function, the system jumps to execute that function, and after completion, it continues to execute the next instruction. During the process of calling the function, the system also needs to perform some tasks based on the function, and these operations are completed by forming a stack frame. The stack frame is a data structure used by the compiler to implement the function calling process. In C language, each stack frame corresponds to an unfinished function.
Let’s look at the execution process of the Add() function through debugging.
int Add(int a, int b) { int z = 0; z = a + b; return z; } int main() { int a = 10; int b = 20; int ret; ret = Add(a, b); printf("%d", ret); system("pause"); return 0; }
In the following debugging process, everyone can qualitatively observe the calling process, which slightly differs from the embedded system.
Before calling the main function, in the VC6.0 editor, we can see that the main function is called in the _tmainCRTStartup function, and the _tmainCRTStartup function is called by mainCRTStartup. This process needs to allocate stack space for the function, which we call the function stack frame.
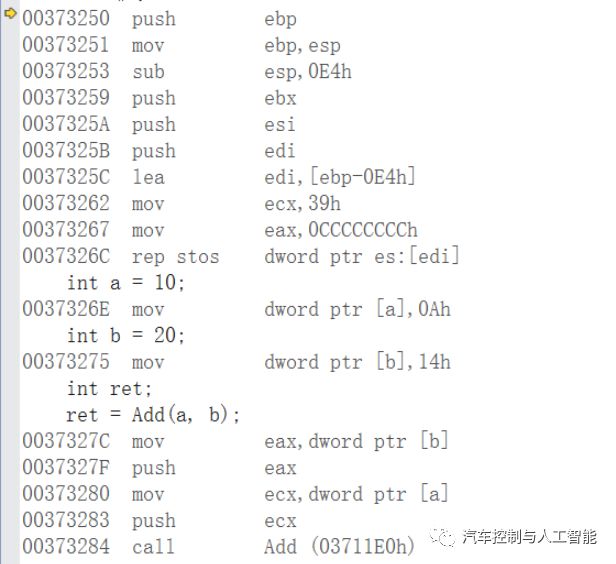
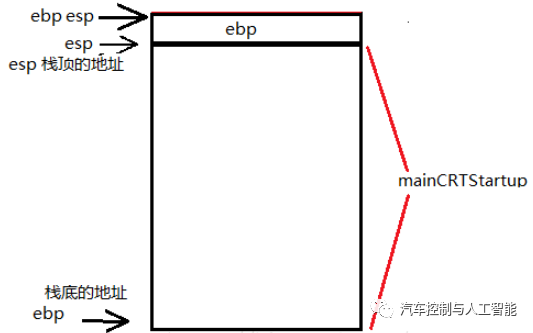
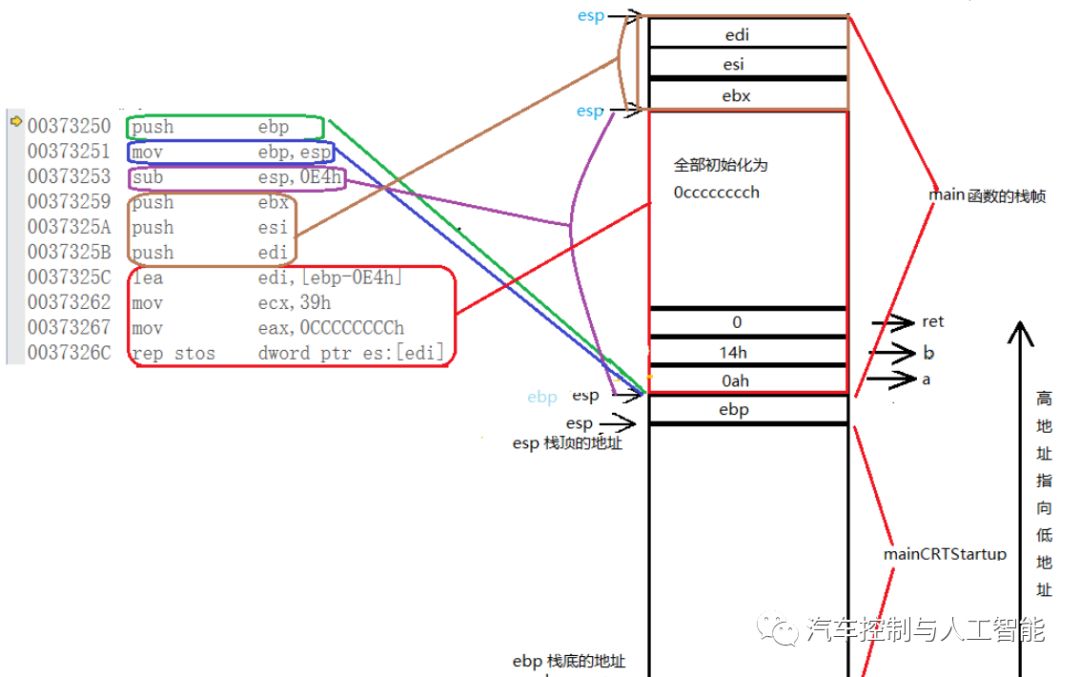
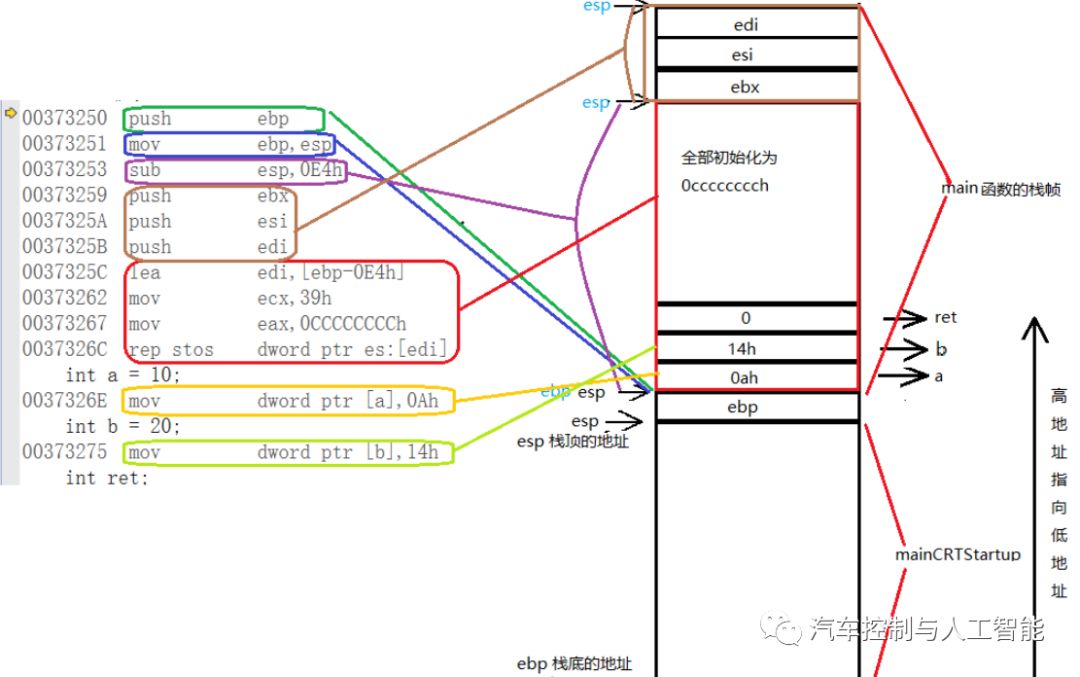
Calling the Add Function
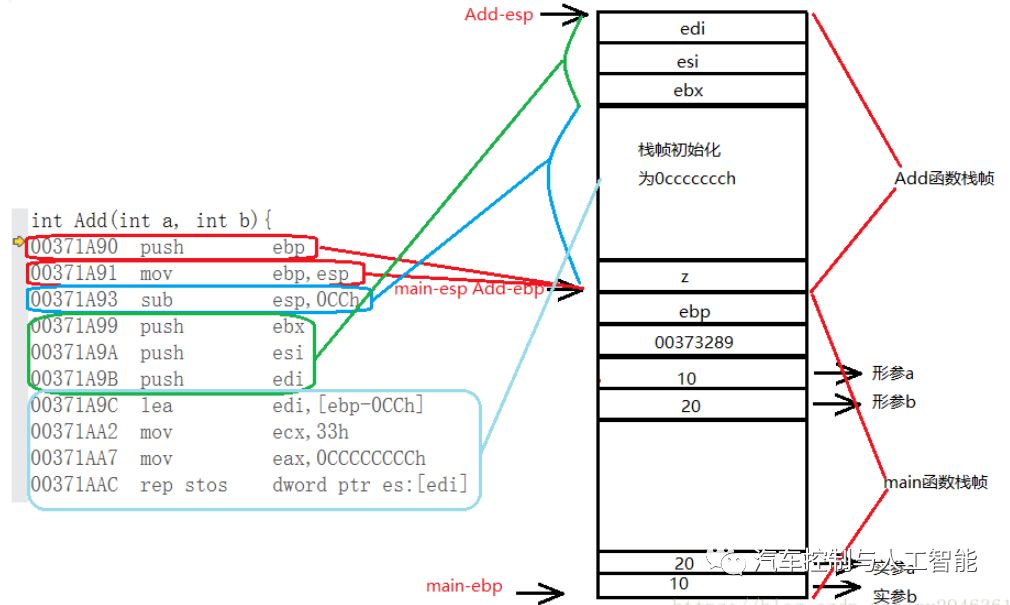
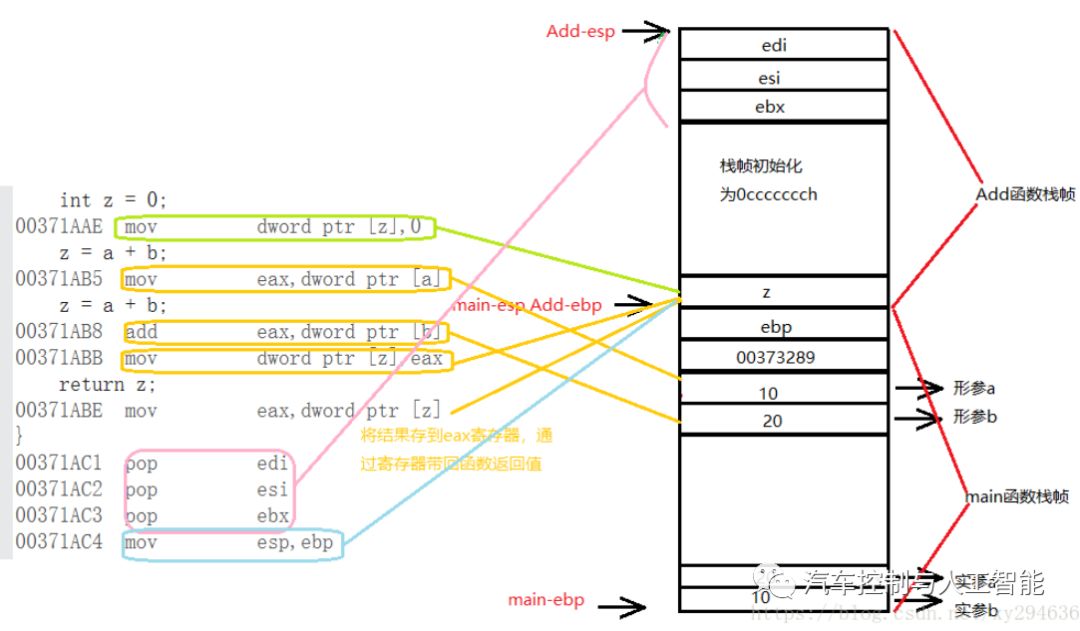
N. Upon executing ret, the previously pushed address will be popped out, thus returning to the main function, which is why we previously pushed this address, so that the call instruction can complete, and then continue executing from that call instruction;
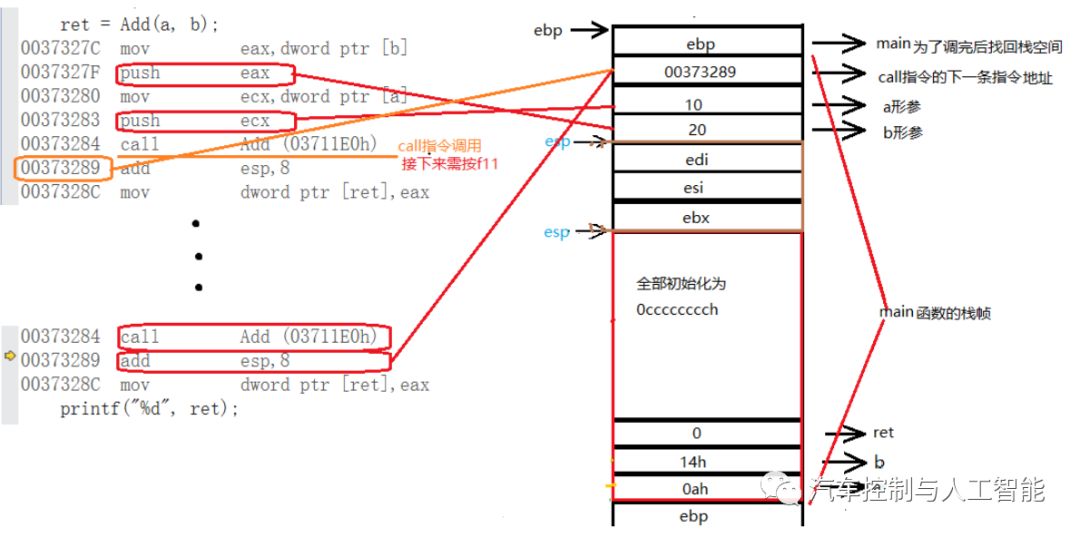
Summary of Stack Frames:
-
Function call framework;
-
Pass parameters;
-
Save return address;
-
Provide local variable space;
Stack-related registers
-
esp, stack pointer
-
ebp, base pointer
-
Push: reduce the top address by 4 bytes (32 bits)
-
Pop: increase the top address by 4 bytes
-
ebp is used in C language to record the base address of the current function call
Here, the function is the Send interface, and the data sent is managed by RTE. However, since this SWCn.c file does not include the .h files in BSW, this method isolates AppL from BSW. Therefore, if the necessary .h files are present, it is also possible to call functions in BSW, but it is not recommended to do so; this process should be completed by RTE for triggering and scheduling.
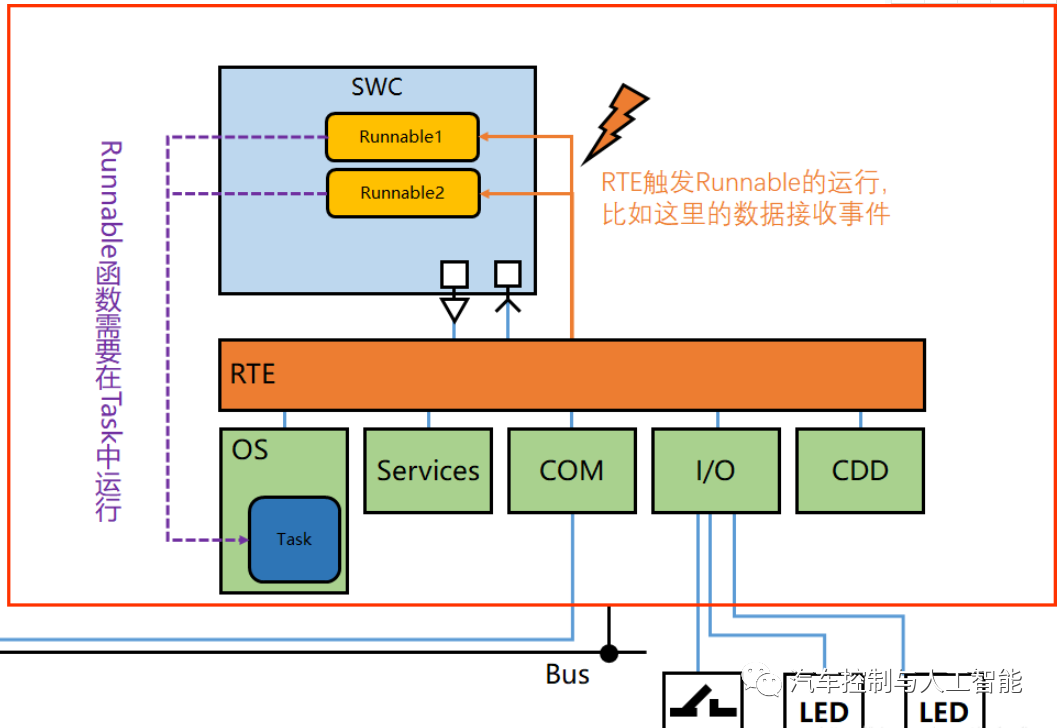
-
Initialization event: automatically triggered upon initialization
-
Timer event: triggers when a periodic timer reaches its time
-
Receiving data event (S/R): triggers when the Receiver Port receives data
-
Receiving data error event (S/R)
-
Data sending completion event (S/R): triggers upon completion of sending by Send Port
-
Operation call event (C/S): triggers when the function is called
-
Asynchronous service return event (C/S): C/S can run asynchronously, meaning when an asynchronous call to a Server function occurs, the called function runs as a thread parallel to the current running program, and when the called function finishes running and returns, the asynchronous service return event is triggered.
-
Mode switch event
-
Mode switch response event
🌏 WeChat: @FighterInAmmonia
✍🏻 Zhihu: @NoBrushTeethAfterEating
👬 Public Account: @AutomobileECUDesign
Collection of Historical Excellent Articles:
[Automobile ECU Design] Collection of Articles on Automotive Buses
[Automobile ECU Design] Collection of Articles on Autosar
[Automobile ECU Design] Collection of Thoughts on Work
[Automobile ECU Design] Directory of Issues 101-150
[Automobile ECU Design] Directory of Issues 51-100
Currently, the public account platform has changed its push mechanism. Those who click “Like”, click “Read”, or have added “Starred” will receive my article pushes first, so everyone remember to click “Read” and “Like” after finishing the article.