However, in RTOS, there is another less common program called Coroutine. Today, let’s briefly discuss coroutines and tasks in RTOS, as well as their differences.
What is a Coroutine?
A coroutine is short for a cooperative routine, and its English name is Coroutine.
A coroutine is a more lightweight concurrent programming model and program component than threads, allowing multiple coroutines to run within a single thread, where these coroutines can be suspended and resumed during execution, thus achieving concurrent execution.
Here is a diagram to understand the relationship between processes, threads, and coroutines:
Coroutines mainly have three states: Running, Ready, and Blocked.
-
Running: When a coroutine is actively executing, it is said to be in the running state, and the current coroutine is using the processor.
-
Ready: A ready coroutine is one that can execute (not blocked) but is not currently executing.
-
Blocked: If a coroutine is currently waiting for a time event or an external event, it is said to be in a blocked state.
Coroutine Function Structure:
void vACoRoutineFunction( CoRoutineHandle_t xHandle, UBaseType_t uxIndex ){ crSTART( xHandle );
for( ;; ) { //-- Co-routine application code here. -- }
crEND();}
Starting with the call to crSTART() and ending with crEND(), the coroutine function should not return any value.
It is actually somewhat similar to tasks in RTOS, but there are many differences as well (differences will be discussed later).
Coroutine Examples
The above description might be a bit abstract for many beginners and also somewhat difficult to understand, so here we will describe coroutines with code examples.
1. Create a Simple LED Blinking Coroutine
void vFlashCoRoutine( CoRoutineHandle_t xHandle, UBaseType_t uxIndex ){ crSTART( xHandle );
for( ;; ) { // Delay for a while crDELAY( xHandle, 10 );
// Blink (toggle LED) vParTestToggleLED( 0 ); }
crEND();}
2. Schedule the Coroutine
Coroutines can be scheduled by calling vCoRoutineSchedule(). This can be done during task idle time:
void vApplicationIdleHook( void ){ vCoRoutineSchedule( void );}
Or by continuously calling it when no other functions are being executed:
void vApplicationIdleHook( void ){ for( ;; ) { vCoRoutineSchedule( void ); }}
3. Create the Coroutine and Start the Task Scheduler
For example, create and start the scheduler in the main() function.
#include "task.h"
#include "croutine.h"
#define PRIORITY_0 0
void main( void ){ // Create coroutine xCoRoutineCreate( vFlashCoRoutine, PRIORITY_0, 0 );
// Start the scheduler. vTaskStartScheduler();}
#include "task.h"
#include "croutine.h"
#define PRIORITY_0 0
#define NUM_COROUTINES 8
void main( void ){ int i;
for( i = 0; i < NUM_COROUTINES; i++ ) { // This time i is passed in as the index. xCoRoutineCreate( vFlashCoRoutine, PRIORITY_0, i ); }
// NOTE: Tasks can also be created here!
// Start the RTOS scheduler. vTaskStartScheduler();}
const int iFlashRates[ NUM_COROUTINES ] = { 10, 20, 30, 40, 50, 60, 70, 80 };
const int iLEDToFlash[ NUM_COROUTINES ] = { 0, 1, 2, 3, 4, 5, 6, 7 }
void vFlashCoRoutine( CoRoutineHandle_t xHandle, UBaseType_t uxIndex ){ crSTART( xHandle );
for( ;; ) { // Set delay based on index crDELAY( xHandle, iFlashRate[ uxIndex ] );
// LED blinking vParTestToggleLED( iLEDToFlash[ uxIndex ] ); } crEND();}
Differences Between Coroutines and Tasks in RTOS
The content of coroutines introduced above through examples should be clear to friends who have used RTOS (tasks) programming. In fact, tasks and coroutines have many similarities, but there are also many differences:
1. Scheduling and Management
Tasks are scheduled and managed by the operating system, while coroutines do not require a system scheduler for management, but are managed by the user.
2. Resource Usage
Tasks consume more system resources due to system calls, while coroutines execute within a single thread and do not consume as much system resources as multithreading.
3. RAM Usage
Coroutines do not occupy system resources, making them more suitable for processors with smaller RAM, such as early 8-bit and 16-bit MCUs.
4. Limitations
The advantage of coroutines is that they use less RAM, but the trade-off is that coroutines have more limitations. Compared to tasks, coroutines are more restrictive and more complex to use.
5. Execution Efficiency
Since coroutine switching is controlled by the program itself and does not incur the overhead of thread switching, coroutines typically have higher execution efficiency.
6. …
In the early days, both MCU resources and performance were relatively low, and some RTOS (like FreeRTOS) had coroutine capabilities. However, as MCU resources and performance have improved, coroutines have been replaced by threads (tasks).
———— END ————
Due to recent changes in the WeChat public platform push rules, many readers have reported not seeing the updated articles in time. According to the latest rules, it is recommended to frequently click on “Recommended Reading, Share, Favorite,” etc., to become a regular reader.
Recommended Reading:
-
System Bug? Xiaomi SU7 Collides Collectively!
-
Original Author Responds! Well-Known Blogger “He Tongxue” Accused of Plagiarizing Open Source Projects
-
Unexpected! Huawei Mate 70 Arrived, Over a Million Units in 80 Minutes
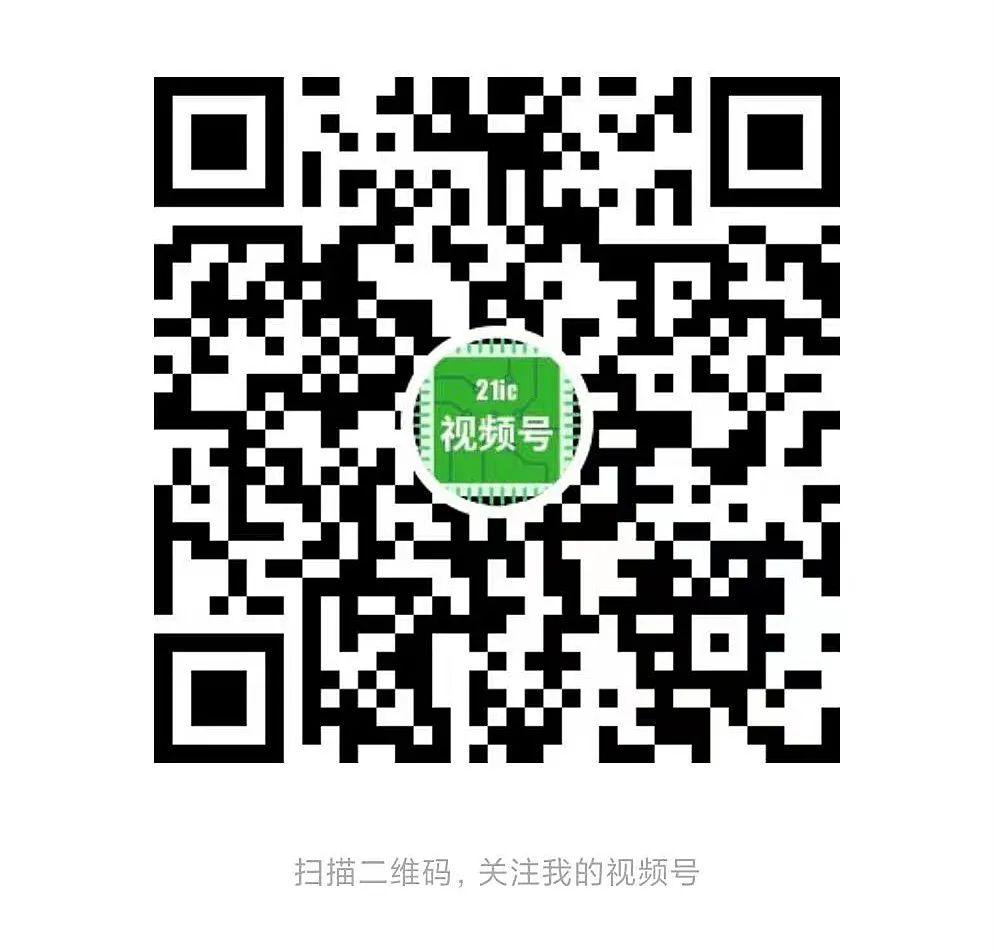
Please click 【Looking】 to give the editor a thumbs up
