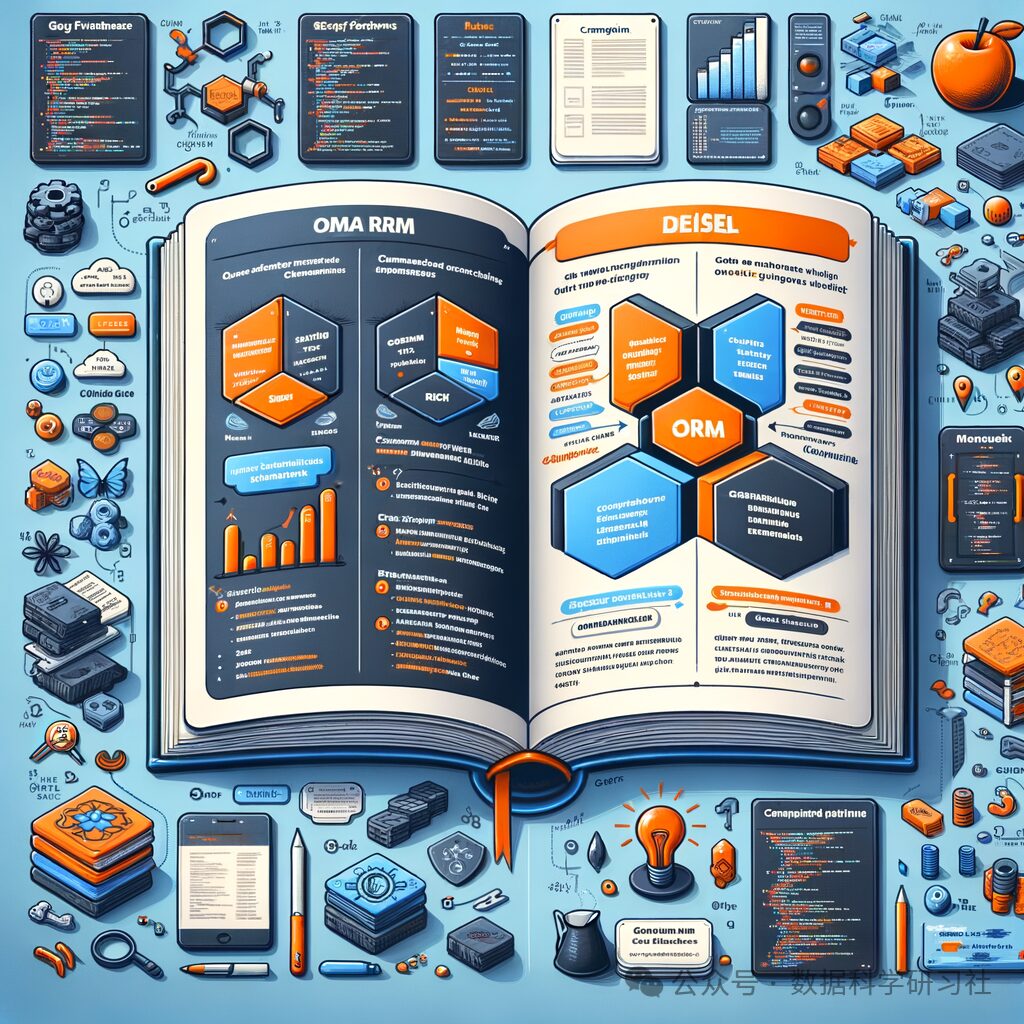
Introduction
Introduction
In the Rust ecosystem, there are many frameworks for handling databases. How do you choose an ORM framework that suits your project? This article will compare mainstream frameworks like Diesel, SQLx, and SeaORM based on dimensions such as stability, safety, flexibility, scalability, usability, and performance to help you make the best choice.
Comparison of Mainstream Frameworks
1. Stability Comparison
Currently, only Diesel and SeaORM have version numbers greater than 1.0:
-
Diesel: Released 1.0 in 2018, 2.0 in 2022 -
SeaORM: Expected to release 1.0 in summer 2024 -
Other frameworks are still in 0.x status
2. Safety Guarantees
Mainstream frameworks provide three levels of safety guarantees:
-
Pure database interface (e.g., tokio-postgres)
-
Only accepts SQL strings -
Errors can only be discovered at runtime
Unchecked query builders (e.g., SeaORM)
-
Provides Rust DSL to build queries -
Ensures syntax correctness -
Cannot check type constraints
Compile-time checks (e.g., Diesel, SQLx)
-
Validates queries at compile time -
Diesel uses the type system for implementation -
SQLx uses procedural macros for implementation
3. Usage Example
Here is a simple example using Diesel:
// Define data model
#[derive(Queryable)]
struct User {
id: i32,
name: String,
email: String,
}
// Query all users
fn get_all_users(conn: &mut PgConnection) -> QueryResult<Vec<User>> {
// Use Diesel's DSL to build the query
use schema::users::dsl::*;
users.load<User>(conn)
}
4. Performance Comparison
According to benchmark results:
-
Sync vs Async
-
SQLite: Sync performance is significantly better than async -
PostgreSQL: Frameworks that support query pipelines (e.g., diesel-async) perform better
-
Data Serialization Efficiency
-
Diesel performs excellently in large data scenarios -
In small data scenarios, differences among frameworks are minor
Framework Selection Recommendations
-
If you value:
-
Type safety → Choose Diesel -
Ease of use → Choose SQLx -
Active community → Choose SeaORM
-
Specific scenario recommendations:
-
SQLite projects → Use sync frameworks -
High concurrency projects → Consider frameworks that support query pipelines -
Enterprise projects → Choose stable versions (>1.0) of frameworks
Conclusion
Diesel, as the most mature ORM framework in the Rust ecosystem, offers powerful compile-time checks and excellent performance. However, when choosing a framework, it is also essential to consider specific project needs, team tech stack, and other factors. There is no absolute good or bad in frameworks; choosing the one that best fits your needs is the most important.
References
-
Compare Diesel: https://diesel.rs/compare_diesel.html
Recommended Books
For all Rust enthusiasts, today I would like to introduce a book titled Programming Rust: Fast, Safe Systems Development (Second Edition) co-authored by Jim Blandy, Jason Orendorff, and Leonora Tindall. This guide delves into the application of the Rust language in system programming, focusing on how to leverage Rust’s unique features to balance performance and safety. The book covers core topics such as Rust’s basic data types, ownership and borrowing concepts, traits and generics, concurrent programming, closures, iterators, and asynchronous programming. This updated edition is based on Rust 2021 and provides comprehensive and practical guidance for system programmers.
-
Rust: The Performance King Sweeping C/C++/Go?
-
A Developer’s Perspective on C++: Revealing Pros and Cons
-
Rust vs Zig: The Battle of Emerging System Programming Languages