Follow+Star Public Account Number, don’t miss out on exciting content
Author | strongerHuang
WeChat Public Account | strongerHuang
Generally speaking, programming on microcontrollers is done in assembly or C language, while using C++ for development is rare.
So, can C++ be used for microcontroller development? The answer is definitely yes.
Below, based on Keil and STM32, I will provide some ideas for beginners on how to use C++ to light up an LED.
Why C++ is Rarely Used for Microcontroller Development
I wonder how much everyone understands about procedural-oriented and object-oriented programming?
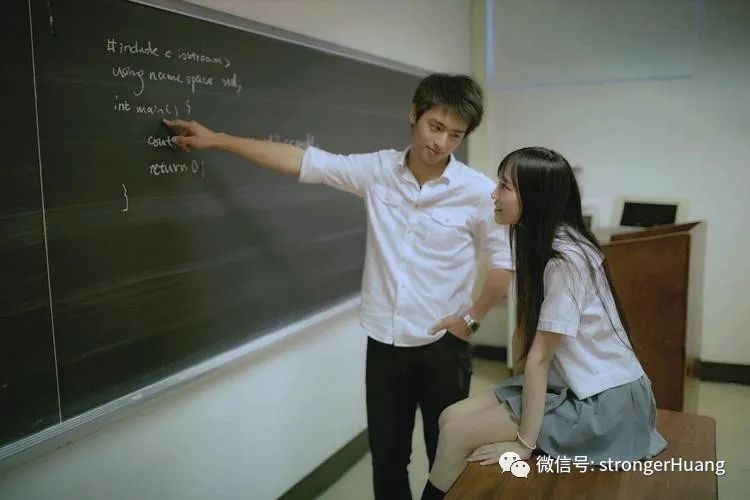
Preparation Work
This is a basic tutorial for Keil. If there are students who do not understand, you can reply with the keyword “Keil Series Tutorial” in the backend of my public account to read more about how to use Keil.
Then, you need to understand some basic syntax of C++. The content discussed in this article is quite basic, using fundamental knowledge of C++. For example: classes, objects, and other foundational concepts.
If you haven’t learned C++, that’s okay; as long as you understand C language, you can easily learn the basics of C++ online.
Usage Instructions
Add C++ source code in the project (for example: main.cpp).
There are some differences in the project configuration options between V5 and V6:
The ST development libraries have already provided support, and you will see a piece of code like this:
#ifdef __cplusplus extern "C" {#endif
//C source code here
#ifdef __cplusplus}#endif
What does this mean?
This is a piece of preprocessing code, and from the preprocessing code, we can see that it roughly means: support for mixed programming in C and C++.
Defining the LED Class
This article discusses a very basic example “LED Lighting”, there are many methods to implement it using C++, and here we will present one of the basic methods.
First, create a main.cpp source code file and define an LED class:
class LED_Class{}
Then define private members (of course, public can also be used):
class LED_Class{private: GPIO_TypeDef *GPIOx; uint16_t GPIO_Pin; uint32_t RCC_APB2Periph;}
Next, we will use functions: initializing GPIO, turning on, and turning off the LED, etc.
class LED_Class{private: GPIO_TypeDef *GPIOx; uint16_t GPIO_Pin; uint32_t RCC_APB2Periph;
public: LED_Class(GPIO_TypeDef *GPIOx,uint16_t GPIO_Pin, uint32_t RCC_APB2Periph){ LED_Class::GPIOx = GPIOx; LED_Class::GPIO_Pin = GPIO_Pin; LED_Class::RCC_APB2Periph = RCC_APB2Periph; }
void Init(void){ GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph, ENABLE); GPIO_InitStruct.GPIO_Pin = GPIO_Pin; GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_Init(GPIOx, &GPIO_InitStruct); }
void Open(void){ GPIO_SetBits(GPIOx, GPIO_Pin); }
void Close(void){ GPIO_ResetBits(GPIOx, GPIO_Pin); }};
Implementing the Flowing LED (main function)
int main(void){ LED_Class LED1(GPIOF, GPIO_Pin_7, RCC_APB2Periph_GPIOF); LED_Class LED2(GPIOF, GPIO_Pin_8, RCC_APB2Periph_GPIOF);
LED1.Init(); LED2.Init();
while(1) { LED1.Open(); LED2.Open(); Delay(10);
LED1.Close(); LED2.Close(); Delay(10); }}
There is also another way to initialize:
int main(void){ LED_Class *LED1 = new LED_Class(GPIOF, GPIO_Pin_7, RCC_APB2Periph_GPIOF); LED_Class *LED2 = new LED_Class(GPIOF, GPIO_Pin_8, RCC_APB2Periph_GPIOF);
LED1->Init(); LED2->Init();
while(1) { LED1->Open(); LED2->Open(); Delay(50);
LED1->Close(); LED2->Close(); Delay(50); }}
The compiled code size is relatively larger:
Then, if you have a development board, just download it to run the LED blinking phenomenon.
Note:
Using C++ to write microcontroller LED light programs can be implemented in many ways, such as using advanced features like inheritance, polymorphism, etc., which can also be introduced. The above simply provides one idea, guiding beginners to learn C++ programming.
Isn’t it simple? Have you learned it by now?
Click “Read the Original” to see more shares.