1. C Language Programming Exercise Description
We have 10 integers, and we need to shift each integer to the right by one position, with the last integer moving to the first position.
For example, the integer sequence: 1,2,3,4,5,6,7,8,9,10
After shifting one position to the right, it becomes: 10,1,2,3,4,5,6,7,8,9
Please implement this program in C language.
2. Analysis of Array Element Shifting
The complete code will be at the end; let’s analyze it step by step.
The first step is to use the scanf function to input 10 integers, which is quite simple:
for(index = 0; index < COUNT; index++) { scanf("%d", &array[index]);}
After inputting, we need to think about how to shift these 10 array elements to the right by one position. I thought about encapsulating this shifting functionality into a dedicated function so that it can be called directly in the main function. I will define this function as follows:
void move(int array[], int len);
The function is named move, where the first parameter array contains the 10 elements, and the second parameter len is 10.
Now comes the key part: to shift the 10 elements to the right, the first 9 elements can be shifted normally, but how do we move the last element to the first position? We can use a variable to store the last element!
num = array[len - 1];
The variable num stores the last element.
How do we shift the first 9 elements to the right? We can start from the 9th element and assign the previous element’s value to the current position!
array[index] = array[index - 1];
Thus, the main logic should be like this.
Let me run my code to see if it works!
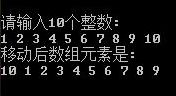
Hey, it works great!
Now, here’s the complete code:
#include <stdio.h>
#define COUNT 10
void move(int array[], int len) { int num = 0, index = 0; num = array[len - 1]; for(index = len - 1; index > 0; index--) { array[index] = array[index - 1]; } array[0] = num;}
int main() { int array[COUNT], index = 0; printf("\n"); printf("Please enter 10 integers:\n"); for(index = 0; index < COUNT; index++) { scanf("%d", &array[index]); } move(array, COUNT); printf("The array elements after shifting are:\n"); for(index = 0; index < COUNT; index++) { printf("%d ", array[index]); } printf("\n\n\n\n"); system("pause"); return 0;}
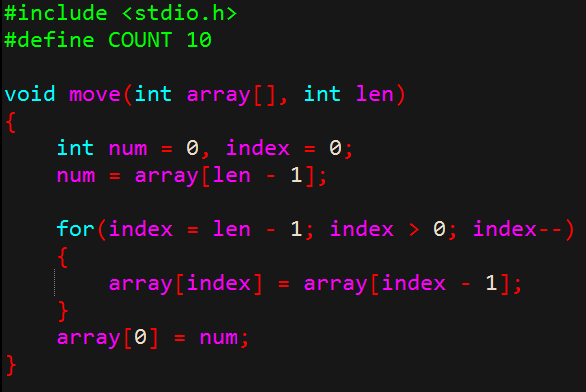
3. Extension of Array Element Shifting
This example of shifting the array is relatively simple; let’s expand it: how to shift the array back by N positions? How to shift the array forward by N positions? N is a variable. Leave it for practice and thought!
If you have questions about what I explained, please reply with the number: 1 to discuss with me!