Hello everyone, today I want to share with you one of my favorite Python libraries – Requests. As the preferred library for sending HTTP requests in Python, its design is very elegant and particularly easy to use. Whether it’s web scraping, calling APIs, or network programming, it is your reliable assistant. Let’s learn about this powerful HTTP request library together today!
1. Quick Start: Installation and Basic Usage
First, we need to install the Requests library. Open the terminal and enter the following command:
pip install requests
Once installed, we can start using it. Let’s look at the simplest example of a GET request:
import requests
# Send a GET request
response = requests.get('https://api.github.com')
print(response.status_code) # Output status code
print(response.text) # Output response content
Isn’t it simple? You can send a request and get a response with just one line of code.
2. Common HTTP Methods
The Requests library supports all common HTTP methods, including GET, POST, PUT, DELETE, etc. Let me give you a few examples:
# GET request with parameters
params = {'key1': 'value1', 'key2': 'value2'}
response = requests.get('https://httpbin.org/get', params=params)
# POST request sending data
data = {'username': 'python', 'password': '123456'}
response = requests.post('https://httpbin.org/post', data=data)
# Sending JSON data
json_data = {'name': 'python', 'age': 30}
response = requests.post('https://httpbin.org/post', json=json_data)
Tip: When using the params parameter, Requests automatically encodes the parameters and adds them to the URL, which is much safer than manually concatenating URLs!
3. Handling Response Content
The Requests library provides various ways to handle server responses:
response = requests.get('https://api.github.com')
# Get response status code
print(response.status_code) # 200
# Check if the request was successful
print(response.ok) # True
# Get response headers
print(response.headers)
# Get JSON response
json_response = response.json()
# Get raw response content
raw_content = response.content
Note: When using the .json() method, ensure that the server returns data in JSON format, otherwise an exception will be thrown!
4. Custom Request Headers and Cookies
Sometimes we need to customize request headers or use cookies, and Requests provides us with simple methods:
# Custom request headers
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36',
'Accept': 'application/json'
}
response = requests.get('https://api.github.com', headers=headers)
# Using cookies
cookies = {'session_id': 'abc123'}
response = requests.get('https://httpbin.org/cookies', cookies=cookies)
5. Handling Timeouts and Exceptions
In network requests, handling timeouts and exceptions is very important. Let’s see how to elegantly handle these situations:
try:
# Set timeout to 3 seconds
response = requests.get('https://api.github.com', timeout=3)
response.raise_for_status() # Raise exception for non-200 status codes
except requests.exceptions.Timeout:
print("Request timed out!")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
Tip: Develop the habit of setting timeouts to avoid the program waiting indefinitely!
Practical Exercise
Let’s do a simple exercise! Write a function to get all public repositories of a specific GitHub user:
def get_github_repos(username):
try:
url = f'https://api.github.com/users/{username}/repos'
response = requests.get(url)
response.raise_for_status()
repos = response.json()
print(f"{username}'s public repositories:")
for repo in repos:
print(f"- {repo['name']}: {repo['description']}")
except requests.exceptions.RequestException as e:
print(f"Failed to get repository information: {e}")
# Call the function
get_github_repos('kennethreitz') # kennethreitz is the author of the Requests library
Conclusion
Today we learned the basic usage of the Requests library, including:
-
Sending various HTTP requests -
Handling request parameters and response content -
Customizing request headers and cookies -
Exception handling
The design philosophy of Requests is “Python for Humans”, making HTTP requests so simple and elegant. I encourage everyone to practice more, try calling some open APIs, or write a simple web scraper. Remember, the most important thing in learning programming is practice!
Next time, I will introduce some advanced uses of Requests, such as Sessions, authentication, proxy settings, etc. Thank you all for reading, and see you next time!
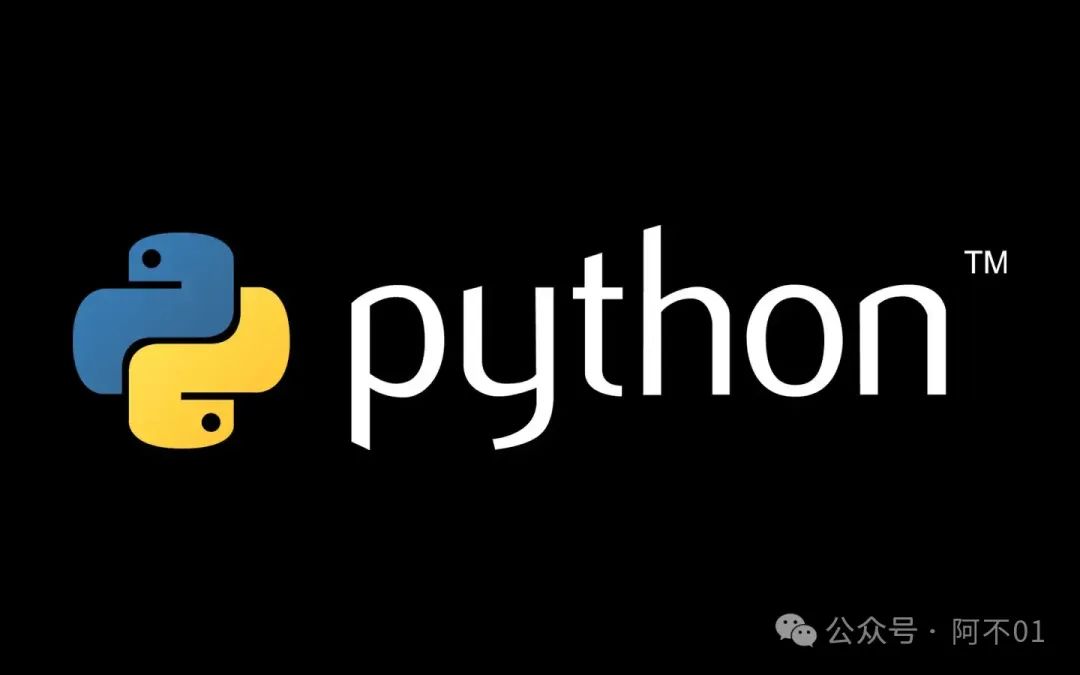