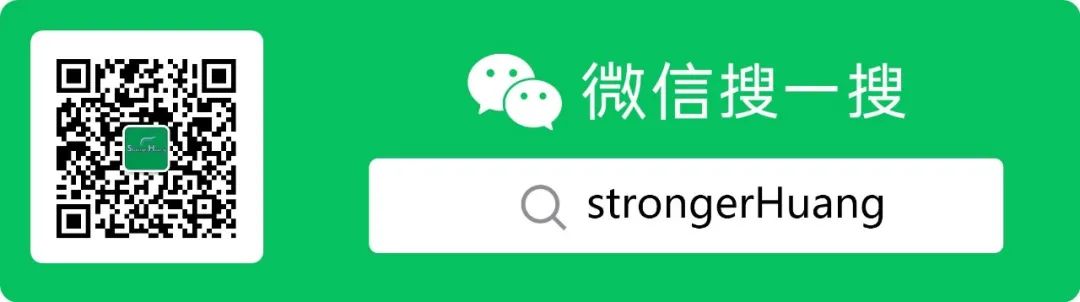
There are many methods for driving LCDs with microcontrollers, and many supporting examples online. However, with thousands of examples online, which one is your “no.1”?
Today, I will share a programming idea for driving LCDs with microcontrollers in an object-oriented way.
Overview of LCD Types
Before discussing how to write an LCD driver, let’s first understand the commonly used embedded LCDs. I will provide an overview of some concepts related to driver architecture design, without going into deep discussions about principles and details, as there will be dedicated articles on this topic or you can refer to online documents.
TFT LCD
TFT LCD, commonly referred to as color screens, typically have high pixel counts, such as the commonly seen 2.8-inch, 320X240 pixels, or 4.0-inch with 800X400 pixels. These screens usually use parallel interfaces, specifically the 8080 or 6800 interfaces (STM32’s FSMC interface); or RGB interfaces, supported by chips such as STM32F429. Others, like those used in smartphones, use MIPI interfaces.
In summary, there are many types of interfaces. There are also some that support SPI interfaces. Unless it is a relatively small screen, it is not recommended to use SPI interfaces due to slow speeds and screen flickering. Common TFT LCD driver ICs used with STM32 include ILI9341/ILI9325, etc.
tft lcd:
IPS:
COG LCD
Many people may not know what COG LCD is. I believe it relates to the current trend of development boards focusing on larger screens and flashy interfaces, without delving into deeper technologies such as software architecture design. COG LCDs actually account for a significant portion of products that use microcontrollers. COG stands for Chip On Glass, meaning the driver chip is directly bonded to the glass, making it transparent. The physical object is as shown below:
This type of LCD usually has lower pixel counts, commonly 128X64 or 128X32. Generally, they only support monochrome displays, although grayscale screens are also available.
The interfaces are usually SPI or I2C. There are also claims of supporting 8-bit parallel interfaces, but they are rarely used; 3 IOs can solve the problem without needing 8. Common driver ICs include STR7565.
OLED LCD
If you’ve bought a development board, you’ve likely used OLEDs. This new technology is perceived as high-end and is commonly used in products like wristbands. OLED screens are currently relatively small, and larger ones are quite expensive. The control mechanism is similar to COG LCDs, but the display methods differ. From a programming perspective, the biggest difference is that OLED LCDs do not require backlight control… The physical object is shown below:
The common interfaces are SPI and I2C. Common driver ICs include SSD1615.
Hardware Scenario
The following discussions will be based on the following hardware information:
1. A TFT screen connected to the hardware’s FSMC interface; model unknown.
2. A COG LCD connected to several ordinary IO ports, with driver IC STR7565, 128X32 pixels.
3. A COG LCD connected to hardware SPI3 and several IO ports, with driver IC STR7565, 128X64 pixels.
4. An OLED LCD connected to SPI3, using CS2 to control the chip select, with driver IC SSD1315.
Prerequisites
Before we delve into the discussion, let’s briefly talk about a few concepts. If you want to learn more about these concepts, please GOOGLE.
Object-Oriented Programming
Object-oriented programming is a concept in the programming world. What does object-oriented mean? Programming has two elements: programs (methods), data (attributes). For example: an LED can be turned on or off, which is called a method. What is the state of the LED? On or off? That is the attribute. We usually program like this:
u8 ledsta = 0;
void ledset(u8 sta)
{
}
This type of programming has a problem. If we have 10 such LEDs, how do we write it? At this point, we can introduce object-oriented programming by encapsulating each LED as an object. We can do it like this:
/*
Define a struct to encapsulate the properties and methods of the LED object.
This struct is an object.
However, this is not a real existence but an abstraction of an object.
*/
typedef struct{
u8 sta;
void (*setsta)(u8 sta);
}LedObj;
/* Declare an LED object named LED1 and implement its method drv_led1_setsta */
void drv_led1_setsta(u8 sta)
{
}
LedObj LED1={
.sta = 0,
.setsta = drv_led1_setsta,
};
/* Declare an LED object named LED2 and implement its method drv_led2_setsta */
void drv_led2_setsta(u8 sta)
{
}
LedObj LED2={
.sta = 0,
.setsta = drv_led2_setsta,
};
/* Function to operate the LED, specifying which LED as a parameter */
void ledset(LedObj *led, u8 sta)
{
led->setsta(sta);
}
Yes, in C language, the means to achieve object-oriented programming is through the use of structs. The above code is very user-friendly for APIs. To operate all LEDs, we use the same interface, just tell the interface which LED to operate. Think about the previously mentioned LCD hardware scenario. For 4 LCDs, if we don’t use object-oriented programming, “wouldn’t the interface for displaying Chinese characters need to be implemented 4 times?” Each screen one?
Separation of Driver and Device
If you want to understand the separation of driver and device in depth, please refer to LINUX driver books.
What is a device? In my opinion, a device is “attributes”, “parameters”, and “data and hardware interface information used by the driver program”. The driver is “the code process that controls these data and interfaces”.
Generally speaking, if the LCD driver ICs are the same, the same driver can be used. Some different ICs can also use the same driver, for example, SSD1315 and STR7565, except for initialization, all others can use the same driver. For example, a COG LCD:
❝
The driver IC is STR7565 128 * 64 pixels using SPI3, backlight using PF5, command line using PF4, reset pin using PF3
❞
All the information above combines to form a device. The driver is the driver code for STR7565.
Why separate the driver and device? To solve the following problem:
❝
There is a new product, a cash register device. The system has two LCDs, both OLED, with the same driver IC, but one is 128×64 and the other is 128×32 pixels, one is called the main display for the cashier; the other is called the customer display for the customer to see the amount.
❞
The best solution to this problem is “to control both devices with the same program”. The means of separating driver and device:
❝
Add device parameters to the driver program interface function parameters, and pass all resources used by the driver through the device parameters.
❞
How do drivers bind to devices? Through the model of the device’s driver IC.
Modularity
I believe modularity means encapsulating a piece of code and providing a stable interface for different drivers to use. Non-modular means implementing this piece of code in different drivers. For example, font library processing, when displaying Chinese characters, we need to find the dot matrix, and when printing Chinese characters on a printer, we also need to find the dot matrix. How do you think the code should be written? Making dot matrix processing a module is modularization. A typical feature of non-modularization is “a line connects to the end without any sense of hierarchy”.
What is LCD?
Earlier we talked about object-oriented programming, and now we need to abstract the LCD to derive an object, which requires knowing what LCD really is. Ask yourself the following questions:
-
What can LCD do? -
What do you want LCD to do? -
Who wants LCD to do what?
Friends who are new to embedded systems may not understand this and may find it hard to comprehend. Let’s simulate the data flow of LCD functionality operation. The APP wants to display a Chinese character on the LCD.
1. First, we need an interface to display Chinese characters; the APP calls this interface to display the character, assuming the interface is called lcd_display_hz.
2. Where does the Chinese character come from? From the dot matrix font library, so within the lcd_display_hz function, we need to call a function called find_font to retrieve the dot matrix.
3. After obtaining the dot matrix, we need to display it on the LCD, so we call an interface called ILL9341_dis to refresh the dot matrix to the LCD with the driver IC model ILI9341.
4. How does ILI9341_dis display the dot matrix? By calling an interface called 8080_WRITE.
Good, this is the general process, and from this process, we can abstract the LCD functionality interface. Do Chinese characters relate to the LCD object? No relation. In the eyes of the LCD, whether it is a Chinese character or an image, they are all dots. So the answers to the previous questions are:
-
LCD can display content dot by dot. -
To make the LCD display Chinese characters or images — it is to display a bunch of dots. -
APP wants the LCD to display images or text.
The conclusion is: the functionality of all LCD objects is to display dots. “Therefore, the driver only needs to provide an interface to display dots, whether to display a single dot or a group of dots.” The abstract interface is as follows:
/*
LCD driver definition
*/
typedef struct
{
u16 id;
s32 (*init)(DevLcd *lcd);
s32 (*draw_point)(DevLcd *lcd, u16 x, u16 y, u16 color);
s32 (*color_fill)(DevLcd *lcd, u16 sx,u16 ex,u16 sy,u16 ey, u16 color);
s32 (*fill)(DevLcd *lcd, u16 sx,u16 ex,u16 sy,u16 ey, u16 *color);
s32 (*onoff)(DevLcd *lcd, u8 sta);
s32 (*prepare_display)(DevLcd *lcd, u16 sx, u16 ex, u16 sy, u16 ey);
void (*set_dir)(DevLcd *lcd, u8 scan_dir);
void (*backlight)(DevLcd *lcd, u8 sta);
}_lcd_drv;
The above interface corresponds to the driver and includes a driver ID number.
-
ID, driver model -
Initialization -
Draw point -
Display a region of points in a certain color -
Display a region of points in certain colors -
Display on/off -
Prepare to refresh area (mainly for color screens using direct DMA refresh) -
Set scanning direction -
Backlight control
Displaying characters, drawing lines, etc., do not belong to the LCD driver. They should be classified at the GUI layer.
LCD Driver Framework
We designed the following driver framework:
Design ideas:
1. The intermediate display driver IC driver program provides a unified interface, structured as the _lcd_drv struct mentioned earlier.
2. Each display IC driver calls different interface drivers based on device parameters. For example, TFT uses 8080 drivers, while others use SPI drivers. There is only one SPI driver, and we also simulate SPI for those controlled by IO ports.
3. The LCD driver layer manages LCDs, including recognizing TFT LCDs and encapsulating all LCD interfaces into a single set of interfaces.
4. The simple GUI layer encapsulates some display functions, such as drawing lines and displaying characters.
5. The font dot matrix module provides interfaces for obtaining and processing dot matrices.
Since it is not as complex in reality, we have combined the GUI and LCD driver layers in the example. The two drivers for TFT LCDs are also placed in one file, but the logic is separated. The OLED, except for initialization, has similar interfaces to COG LCDs, so these two drivers are also placed in one file.
Code Analysis
The code is divided into three layers:
1. GUI and LCD driver layer: dev_lcd.c dev_lcd.h
2. Display driver IC layer: dev_str7565.c & dev_str7565.h, dev_ILI9341.c & dev_ILI9341.h
3. Interface layer: mcu_spi.c & mcu_spi.h, stm324xg_eval_fsmc_sram.c & stm324xg_eval_fsmc_sram.h
GUI and LCD Layer
This layer primarily has three functions:
“1. Device Management”
First, we define a series of LCD parameter structs, which include ID and pixel dimensions. These structs are then combined into a list array.
/* Various LCD specifications */
_lcd_pra LCD_IIL9341 ={
.id = 0x9341,
.width = 240, //LCD width
.height = 320, //LCD height
};
...
/* Various LCD list */
_lcd_pra *LcdPraList[5]=
{
&LCD_IIL9341,
&LCD_IIL9325,
&LCD_R61408,
&LCD_Cog12864,
&LCD_Oled12864,
};
Then we define all driver list arrays, where the contents are the drivers implemented in the corresponding driver files.
/* All driver lists
Driver list */
_lcd_drv *LcdDrvList[] = {
&TftLcdILI9341Drv,
&TftLcdILI9325Drv,
&CogLcdST7565Drv,
&OledLcdSSD1615rv,
We define a device tree, which specifies how many LCDs the system has, which interfaces they are connected to, and what driver ICs they use. In a complete system, this could be made into a device tree similar to LINUX.
/*Device tree definition*/
#define DEV_LCD_C 3//System has 3 LCD devices
LcdObj LcdObjList[DEV_LCD_C]=
{
{"oledlcd", LCD_BUS_VSPI, 0X1315},
{"coglcd", LCD_BUS_SPI, 0X7565},
{"tftlcd", LCD_BUS_8080, NULL},
};
“2. Interface Encapsulation”
void dev_lcd_setdir(DevLcd *obj, u8 dir, u8 scan_dir)
s32 dev_lcd_init(void)
DevLcd *dev_lcd_open(char *name)
s32 dev_lcd_close(DevLcd *dev)
s32 dev_lcd_drawpoint(DevLcd *lcd, u16 x, u16 y, u16 color)
s32 dev_lcd_prepare_display(DevLcd *lcd, u16 sx, u16 ex, u16 sy, u16 ey)
s32 dev_lcd_display_onoff(DevLcd *lcd, u8 sta)
s32 dev_lcd_fill(DevLcd *lcd, u16 sx,u16 ex,u16 sy,u16 ey,u16 *color)
s32 dev_lcd_color_fill(DevLcd *lcd, u16 sx,u16 ex,u16 sy,u16 ey,u16 color)
s32 dev_lcd_backlight(DevLcd *lcd, u8 sta)
Most of the interfaces are secondary encapsulations of the driver IC interfaces. The difference lies in the initialization and opening interfaces. Initialization involves finding the corresponding driver based on the previously defined device tree, locating the corresponding device parameters, and completing device initialization. The opening function searches for the device based on the passed device name, and upon finding it, returns the device handle, which is needed for all subsequent operations.
“3. Simple GUI Layer”
Currently, the most important function is the character display function.
s32 dev_lcd_put_string(DevLcd *lcd, FontType font, int x, int y, char *s, unsigned colidx)
Other functions for drawing lines and circles are currently just tests and will be improved later.
Driver IC Layer
The driver IC layer is divided into two parts:
“1. Encapsulating LCD Interfaces”
LCDs use either the 8080 bus or the SPI bus, and these bus functions are implemented in separate files. However, in addition to these communication signals, LCDs also have reset signals, command data line signals, backlight signals, etc. We encapsulate these signals along with the communication interfaces into a “LCD communication bus”, also known as buslcd. BUS_8080 is encapsulated in the dev_ILI9341.c file. BUS_LCD1 and BUS_lcd2 are encapsulated in dev_str7565.c.
“2. Driver Implementation”
Implement the _lcd_drv driver structure. Each driver implements one, and some drivers can share functions.
_lcd_drv CogLcdST7565Drv = {
.id = 0X7565,
.init = drv_ST7565_init,
.draw_point = drv_ST7565_drawpoint,
.color_fill = drv_ST7565_color_fill,
.fill = drv_ST7565_fill,
.onoff = drv_ST7565_display_onoff,
.prepare_display = drv_ST7565_prepare_display,
.set_dir = drv_ST7565_scan_dir,
.backlight = drv_ST7565_lcd_bl
};
Interface Layer
The 8080 layer is relatively simple, using official interfaces. The SPI interface provides the following operation functions, which can operate both SPI and VSPI.
extern s32 mcu_spi_init(void);
ext s32 mcu_spi_open(SPI_DEV dev, SPI_MODE mode, u16 pre);
ext s32 mcu_spi_close(SPI_DEV dev);
ext s32 mcu_spi_transfer(SPI_DEV dev, u8 *snd, u8 *rsv, s32 len);
ext s32 mcu_spi_cs(SPI_DEV dev, u8 sta);
As for why SPI is written this way, there will be a separate file explaining it.
Overall Process
How do the previously mentioned modules connect? Please see the following structure:
/* During initialization, the number of devices will be defined,
and the driver and parameters will be matched, initializing variables.
When opening, it only retrieves a pointer */
typedef struct _strDevLcd
{
s32 gd;//Handle, controls whether it can be opened
LcdObj *dev;
/* LCD parameters, fixed, unchangeable*/
_lcd_pra *pra;
/* LCD driver */
_lcd_drv *drv;
/* Variables needed by the driver */
u8 dir; //Portrait or landscape control: 0, portrait; 1, landscape.
u8 scandir;//Scanning direction
u16 width; //LCD width
u16 height; //LCD height
void *pri;//Private data, black and white screens and OLED screens will allocate display memory during initialization
};
Each device will have such a structure, which is initialized when the LCD is initialized.
-
The member dev points to the device tree, from which we can know the device name, which LCD bus it is connected to, and the device ID.
typedef struct
{
char *name;//Device name
LcdBusType bus;//Connected to which LCD bus
u16 id;
}LcdObj;
-
The member pra points to the LCD parameters, allowing us to know the specifications of the LCD.
typedef struct
{
u16 id;
u16 width; //LCD width Portrait
u16 height; //LCD height Portrait
}_lcd_pra;
-
The member drv points to the driver, with all operations implemented through drv.
typedef struct
{
u16 id;
s32 (*init)(DevLcd *lcd);
s32 (*draw_point)(DevLcd *lcd, u16 x, u16 y, u16 color);
s32 (*color_fill)(DevLcd *lcd, u16 sx,u16 ex,u16 sy,u16 ey, u16 color);
s32 (*fill)(DevLcd *lcd, u16 sx,u16 ex,u16 sy,u16 ey, u16 *color);
s32 (*prepare_display)(DevLcd *lcd, u16 sx, u16 ex, u16 sy, u16 ey);
s32 (*onoff)(DevLcd *lcd, u8 sta);
void (*set_dir)(DevLcd *lcd, u8 scan_dir);
void (*backlight)(DevLcd *lcd, u8 sta);
}_lcd_drv;
-
The members dir, scandir, width, and height are common variables used by the driver. Since each LCD has its own structure, a single driver program can control multiple devices without interference. -
The member pri is a private pointer; some drivers may need special variables, so this pointer records them, typically pointing to a structure defined by the driver, and memory is allocated for it during device initialization. Currently, it is mainly used for caches for COG LCD and OLED LCD displays.
The entire LCD driver is combined through this structure.
1. During initialization, based on the device tree, the driver and parameters are found, and the structure mentioned above is initialized.
2. Before using the LCD, call the dev_lcd_open function. If successful, it returns a pointer to the structure mentioned above.
3. To display characters, after finding the dot matrix through the interface, we call the corresponding driver program using the drv of the structure.
4. The driver program decides which LCD bus to operate based on this structure and uses the variables of this structure.
Usage and Benefits
-
Benefit 1
Please see the test program
void dev_lcd_test(void)
{
DevLcd *LcdCog;
DevLcd *LcdOled;
DevLcd *LcdTft;
/* Open three devices */
LcdCog = dev_lcd_open("coglcd");
if(LcdCog==NULL)
uart_printf("open cog lcd err\r\n");
LcdOled = dev_lcd_open("oledlcd");
if(LcdOled==NULL)
uart_printf("open oled lcd err\r\n");
LcdTft = dev_lcd_open("tftlcd");
if(LcdTft==NULL)
uart_printf("open tft lcd err\r\n");
/*Turn on the backlight*/
dev_lcd_backlight(LcdCog, 1);
dev_lcd_backlight(LcdOled, 1);
dev_lcd_backlight(LcdTft, 1);
dev_lcd_put_string(LcdOled, FONT_SONGTI_1212, 10,1, "ABC-abc,", BLACK);
dev_lcd_put_string(LcdOled, FONT_SIYUAN_1616, 1, 13, "This is oled lcd", BLACK);
dev_lcd_put_string(LcdOled, FONT_SONGTI_1212, 10,30, "www.wujique.com", BLACK);
dev_lcd_put_string(LcdOled, FONT_SIYUAN_1616, 1, 47, "Roof Sparrow Studio", BLACK);
dev_lcd_put_string(LcdCog, FONT_SONGTI_1212, 10,1, "ABC-abc,", BLACK);
dev_lcd_put_string(LcdCog, FONT_SIYUAN_1616, 1, 13, "This is cog lcd", BLACK);
dev_lcd_put_string(LcdCog, FONT_SONGTI_1212, 10,30, "www.wujique.com", BLACK);
dev_lcd_put_string(LcdCog, FONT_SIYUAN_1616, 1, 47, "Roof Sparrow Studio", BLACK);
dev_lcd_put_string(LcdTft, FONT_SONGTI_1212, 20,30, "ABC-abc,", RED);
dev_lcd_put_string(LcdTft, FONT_SIYUAN_1616, 20,60, "This is tft lcd", RED);
dev_lcd_put_string(LcdTft, FONT_SONGTI_1212, 20,100, "www.wujique.com", RED);
dev_lcd_put_string(LcdTft, FONT_SIYUAN_1616, 20,150, "Roof Sparrow Studio", RED);
while(1);
}
Using a single function dev_lcd_open, we can open three LCDs and obtain LCD devices. Then, by calling dev_lcd_put_string, we can display on different LCDs. All other GUI operation interfaces are unified. This design is very user-friendly for the APP layer. Display effect:
-
Benefit 2
The current device tree is defined as follows
LcdObj LcdObjList[DEV_LCD_C]=
{
{"oledlcd", LCD_BUS_VSPI, 0X1315},
{"coglcd", LCD_BUS_SPI, 0X7565},
{"tftlcd", LCD_BUS_8080, NULL},
};
One day, if the oled lcd needs to be connected to SPI, we just need to change the parameters in the device tree array, and of course, you can’t connect two devices to one interface.
LcdObj LcdObjList[DEV_LCD_C]=
{
{"oledlcd", LCD_BUS_SPI, 0X1315},
{"tftlcd", LCD_BUS_8080, NULL},
};
Font Library
Currently not elaborated, the font library in the example is stored on the SD card, and you can modify it based on your needs during porting. Please refer to font.c for details.
Disclaimer
This method is for learning programming ideas and techniques and may not be suitable for all projects.
———— END ————
● Column “Embedded Tools”
● Column “Embedded Development”
● Column “Keil Tutorial”
● Selected Tutorials for Embedded Columns
Follow the public account reply “Join group” to join the technical exchange group according to the rules, reply “1024” for more content.
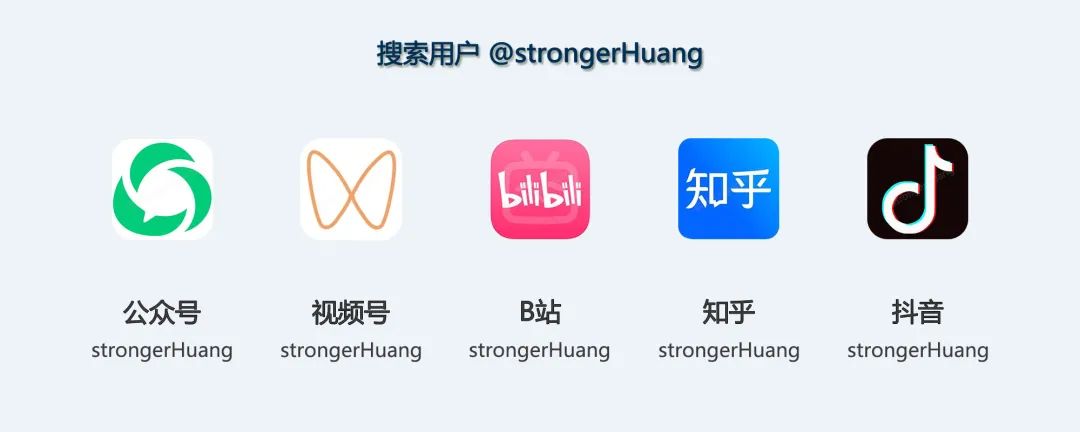
Click “Read the original text” for more shares.