Predicting Stock Trends with Python: A Beginner’s Guide
Hello everyone, I am your learning assistant Xiaolizi! Today I want to share how to use Python to predict stock trends.
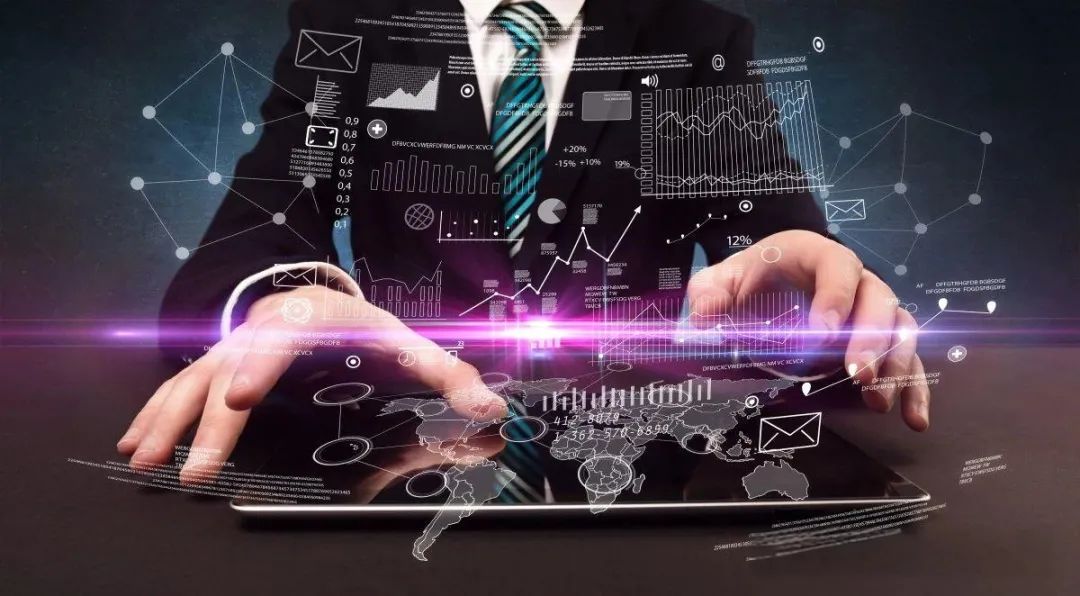
As a data analysis enthusiast, I have found that many friends are especially interested in stock predictions. But don’t worry, even if you are a Python beginner, you can easily get started with stock data analysis by following my pace!
Preparation: Install Necessary Libraries
First, we need to install some commonly used Python libraries. Open the command line and enter the following commands:
pip install pandas
pip install numpy
pip install yfinance
pip install matplotlib
These libraries are used for data processing, numerical calculations, fetching stock data, and plotting charts.
Fetching Stock Data
Let’s start by fetching stock data. I usually use Yahoo Finance’s API to get data, which is very convenient:
import yfinance as yf
import pandas as pd
import matplotlib.pyplot as plt
# Get Apple's stock data for the past year
stock = yf.Ticker("AAPL")
df = stock.history(period="1y")
print(df.head())
Tip: The <span>yfinance</span>
library supports data from major global stock markets. If you want to get data from the A-shares market, you can use the <span>tushare</span>
library!
Calculating Moving Averages
In stock analysis, moving averages are the most basic technical indicators. Let’s calculate the 20-day and 50-day moving averages:
# Calculate moving averages
df['MA20'] = df['Close'].rolling(window=20).mean()
df['MA50'] = df['Close'].rolling(window=50).mean()
# Plot stock price and moving averages
plt.figure(figsize=(12,6))
plt.plot(df.index, df['Close'], label='Stock Price')
plt.plot(df.index, df['MA20'], label='20-Day MA')
plt.plot(df.index, df['MA50'], label='50-Day MA')
plt.title('Stock Price Trend Chart')
plt.legend()
plt.show()
Calculating Stock Returns
To assess the risk and return of stocks, we need to calculate daily returns:
# Calculate daily returns
df['Returns'] = df['Close'].pct_change()
# Calculate cumulative returns
df['Cumulative_Returns'] = (1 + df['Returns']).cumprod()
print(f"Annualized Return: {df['Returns'].mean() * 252:.2%}")
print(f"Annualized Volatility: {df['Returns'].std() * np.sqrt(252):.2%}")
Note: The 252 here is the number of trading days in a year, used for annualization calculations.
Simple Trend Prediction
We can use simple linear regression to predict future trends:
from sklearn.linear_model import LinearRegression
import numpy as np
# Create features and target variable
X = np.arange(len(df)).reshape(-1, 1)
y = df['Close'].values
# Train linear regression model
model = LinearRegression()
model.fit(X, y)
# Predict future 30 days
future_dates = np.arange(len(df), len(df) + 30).reshape(-1, 1)
future_prices = model.predict(future_dates)
print("Predicted price trend for the next 30 days:")
print(future_prices[-1])
Tip: This is the most basic prediction method, and in actual trading, more factors need to be considered!
Practical Exercises
-
Try fetching data for other stocks and calculate their returns. -
Modify the moving average periods and observe the effects of different periods. -
Try adding more technical indicators like RSI or MACD.
Advanced Tips
-
Stock prediction needs to consider multiple factors; a single indicator may be misleading. -
In actual trading, consider transaction costs and slippage. -
It is recommended to backtest with historical data to verify the effectiveness of the strategy.
Friends, today’s Python learning journey ends here! Remember to code along, and feel free to ask me any questions in the comments!
Stock prediction is a profound topic, and what we learned today is just the tip of the iceberg, but it’s enough to get started! Wishing everyone a happy learning experience and continuous improvement in Python!