Congratulations! The new board has finally arrived, without further ado, let’s get started.
The main function of the ESP32 is WIFI. With WIFI, it can directly connect to the home WIFI router and access the internet. At this point, it acts like a small computer or smartphone, making it very convenient to connect to the internet.
The ESP32 WIFI has three main modes:
-
1. AP mode (WIFI router mode), where it becomes a router itself, providing WIFI services to other devices.
-
2. STA mode (the mode of a computer or smartphone), where it connects to the home WIFI.
-
3. Mixed mode.
However, for the NanoFramework’s WIFI, STA mode has not been debugged yet, but AP mode is working fine, so let’s start with AP mode.
We will implement a function to scan all available WIFI networks, connect to a WIFI network, and retrieve web page information using http request.
This is targeted functionality to avoid getting lost.
WifiScan
First, remember to install the NuGet package:
Install-Package nanoFramework.System.Device.Wifi -Version 1.4.0.22
The code is quite simple, let’s look at the code directly:
public class Program
{
public static void Main()
{
try
{
// Get the WIFI adapter
WifiAdapter wifi = WifiAdapter.FindAllAdapters()[0];
// Set network change event
wifi.AvailableNetworksChanged += Wifi_AvailableNetworksChanged;
// Loop to scan WIFI list
while (true)
{
Debug.WriteLine("Starting WIFI scan");
wifi.ScanAsync();
Thread.Sleep(30000);
}
}
catch (Exception ex)
{
Debug.WriteLine("message:" + ex.Message);
Debug.WriteLine("stack:" + ex.StackTrace);
}
Thread.Sleep(Timeout.Infinite);
}
/// <summary>
/// Scan completion traversal
/// </summary>
private static void Wifi_AvailableNetworksChanged(WifiAdapter sender, object e)
{
Debug.WriteLine("Obtaining valid WIFI information");
WifiNetworkReport report = sender.NetworkReport;
foreach (WifiAvailableNetwork net in report.AvailableNetworks)
{
Debug.WriteLine($"WIFI Name (SSID):{net.Ssid}, MAC Address (BSSID): {net.Bsid}, Signal Strength (rssi): {net.NetworkRssiInDecibelMilliwatts.ToString()}, Signal Strength (signal): {net.SignalBars.ToString()}");
}
}
}
Viewing the Output Results
We can see that we can directly obtain the information we want.
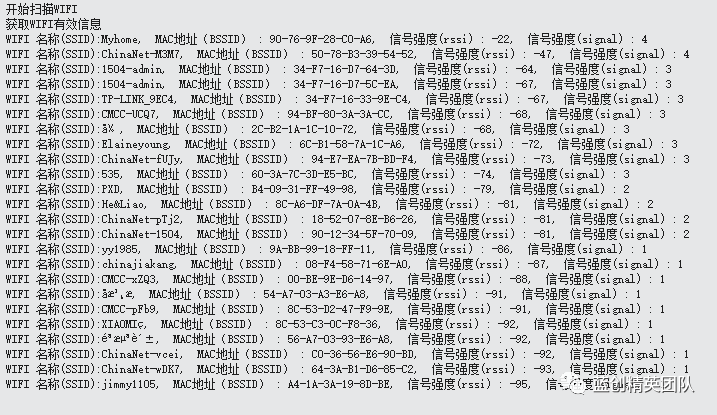
WIFI Connection Functionality
Let’s look at the code directly:
public class Program
{
const string MYSSID = "Myhome";
const string MYPASSWORD = "1213141516";
public static void Main()
{
try
{
var success = WifiNetworkHelper.ConnectDhcp(MYSSID, MYPASSWORD, requiresDateTime: true, token: new CancellationTokenSource(60000).Token);
if (success)
{
Debug.WriteLine($"Connection Status: {success}");
Debug.WriteLine($"We obtained the latest time: {DateTime.UtcNow.AddHours(8)}");
}
else
{
Debug.WriteLine("An exception occurred");
}
}
catch (Exception e)
{
Debug.WriteLine($"{e.Message}");
}
Thread.Sleep(Timeout.Infinite);
}
}
It has connected successfully and obtained the latest time, but this time actually has a timezone, we need to add 8 hours to it.
The effect is as follows:
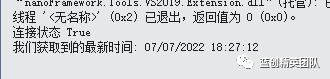
Wifi HttpRequest
public class Program
{
const string MYSSID = "Myhome";
const string MYPASSWORD = "1213141516";
public static void Main()
{
try
{
var success = WifiNetworkHelper.ConnectDhcp(MYSSID, MYPASSWORD, requiresDateTime: true, token: new CancellationTokenSource(60000).Token);
if (success)
{
Debug.WriteLine($"Connection Status: {success}");
Debug.WriteLine($"We obtained the latest time: {DateTime.UtcNow.AddHours(8)}");
}
else
{
Debug.WriteLine("An exception occurred");
}
X509Certificate rootCACert = new X509Certificate(Resource.GetBytes(Resource.BinaryResources.digicertglobalrootca));
// Request address and obtain information
var httpWebRequest = (HttpWebRequest)WebRequest.Create("https://kesshei.github.io/esp32.html");
httpWebRequest.Method = "GET";
httpWebRequest.SslProtocols = System.Net.Security.SslProtocols.Tls12;
httpWebRequest.HttpsAuthentCert = rootCACert;
using (var httpWebResponse = (HttpWebResponse)httpWebRequest.GetResponse())
{
StreamReader sr = new StreamReader(httpWebResponse.GetResponseStream());
var data = sr.ReadToEnd();
Debug.WriteLine(">>>>>>>>>>>>>");
Debug.WriteLine("Request completed");
Debug.WriteLine($"Obtained: {data} Data Length: {data.Length}");
}
}
catch (Exception e)
{
Debug.WriteLine($"{e.Message}");
}
Thread.Sleep(Timeout.Infinite);
}
}
One thing to note here is the difference between http and https.
If the following two lines are not needed:
httpWebRequest.SslProtocols = System.Net.Security.SslProtocols.Tls12;
httpWebRequest.HttpsAuthentCert = rootCACert;
Then it can only use http. In my case, it is set up this way.
To use https, SSL support must be added along with a certificate.
Running Results
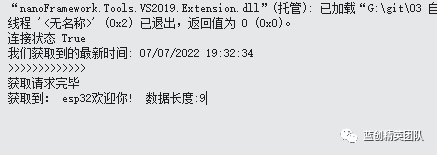
Conclusion
Finally, we have tackled the most important aspect of ESP32 WIFI. Without this, we wouldn’t be able to play around with it. Now it should be considered as a beginner’s entry.
To all the experts, enjoy!
Code Repository
https://github.com/kesshei/NanoFreamworkWifiDemo.git
gitee (500 error, will be resubmitted later)
End
Don’t forget to support with a one-click three-link! Your support is my motivation!