Introduction to FreeRTOS Interrupts

/* Cortex-M specific definitions. */
#ifdef __NVIC_PRIO_BITS
/* __BVIC_PRIO_BITS will be specified when CMSIS is being used. */
#define configPRIO_BITS __NVIC_PRIO_BITS
#else
#define configPRIO_BITS 4 /* 15 priority levels */
#endif
/* The lowest interrupt priority that can be used in a call to a "set priority"
function. */
#define configLIBRARY_LOWEST_INTERRUPT_PRIORITY 0xf
/* The highest interrupt priority that can be used by any interrupt service
routine that makes calls to interrupt safe FreeRTOS API functions. DO NOT CALL
INTERRUPT SAFE FREERTOS API FUNCTIONS FROM ANY INTERRUPT THAT HAS A HIGHER
PRIORITY THAN THIS! (higher priorities are lower numeric values. */
#define configLIBRARY_MAX_SYSCALL_INTERRUPT_PRIORITY 5
/* Interrupt priorities used by the kernel port layer itself. These are generic
to all Cortex-M ports, and do not rely on any particular library functions. */
#define configKERNEL_INTERRUPT_PRIORITY ( configLIBRARY_LOWEST_INTERRUPT_PRIORITY << (8 - configPRIO_BITS) )
/* !!!! configMAX_SYSCALL_INTERRUPT_PRIORITY must not be set to zero !!!!
See http://www.FreeRTOS.org/RTOS-Cortex-M3-M4.html. */
#define configMAX_SYSCALL_INTERRUPT_PRIORITY ( configLIBRARY_MAX_SYSCALL_INTERRUPT_PRIORITY << (8 - configPRIO_BITS) )
Interrupts with a priority higher than configMAX_SYSCALL_INTERRUPT_PRIORITY cannot be disabled.
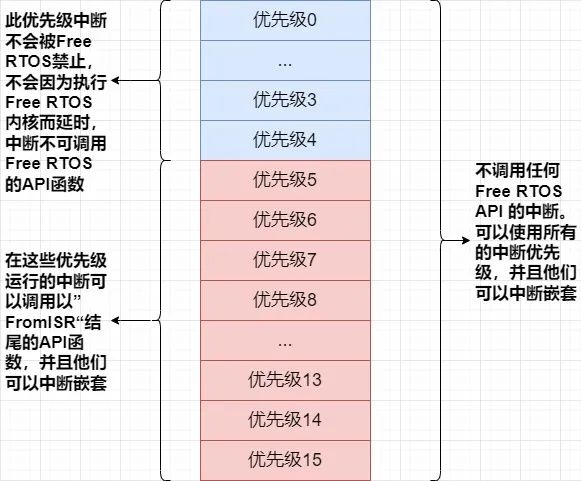
Testing Process

1、Create an interrupttest task
xTaskCreate((TaskFunction_t )interrupt_task, //Task function
(const char* )"interrupt_task", //Task name
(uint16_t )INTERRUPT_STK_SIZE, //Task stack size
(void* )NULL, //Parameters passed to the task function
(UBaseType_t )INTERRUPT_TASK_PRIO, //Task priority
(TaskHandle_t* )&INTERRUPTTask_Handler); //Task handle
2、Interrupt Test Task Function
void interrupt_task(void *pvParameters)
{
static u32 count_num=0;
while(1)
{
count_num++;
if(count_num==5)
{
printf("Disabling interrupts.............\r\n");
portDISABLE_INTERRUPTS(); //Disable interrupts
delay_xms(5000); //Delay 5s
printf("Enabling interrupts.............\r\n");
portENABLE_INTERRUPTS(); //Enable interrupts
}
vTaskDelay(1000);//Delay for 1000 clock ticks, which is 1s configTICK_RATE_HZ
}
}
3、Timer Interrupt Service Functions
void TIM6_IRQHandler(void)
{
if(TIM_GetITStatus(TIM6, TIM_IT_Update)) //Check if interrupt occurred
{
printf("TIM6 interrupt\r\n");
TIM_ClearITPendingBit(TIM6,TIM_IT_Update);//Clear interrupt flag
}
}
void TIM7_IRQHandler(void)
{
if(TIM_GetITStatus(TIM7, TIM_IT_Update)) //Check if interrupt occurred
{
printf("TIM7 interrupt\r\n");
TIM_ClearITPendingBit(TIM7,TIM_IT_Update);//Clear interrupt flag
}
}
4、Testing Results
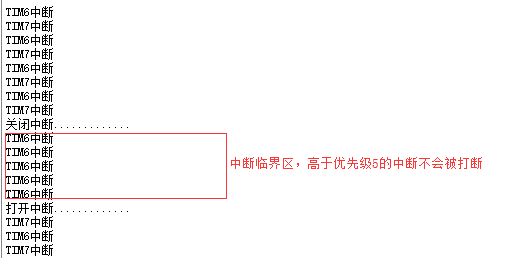
5、Precautions
Do not use the vTaskDelay() function in critical sections, interrupt service functions, or when enabling/disabling interrupts, as this function will yield the CPU for a period of time. If the task does not delay or suspend, lower-priority tasks will not be able to obtain CPU time.