01
1.1 Basics of Programming Languages
C Language: As the cornerstone of embedded development, it is essential to master its various features.
By carefully studying classic books such as “The C Programming Language,” deeply understand the syntax rules, accurately grasp the use of data types, skillfully manipulate pointers as a powerful tool, and be proficient in writing various functions.
It is recommended to manually input and debug code during the learning process to deepen the understanding and memory of knowledge points through practical operations.
For example, while learning pointers, personally writing code to implement pointer arithmetic and operations pointing to arrays, structures, and other complex data types to truly master the essence of pointers.
C++: Building on the foundation of C language, further explore the wonderful world of object-oriented programming.
Learn to create and use classes and objects, understand core concepts such as inheritance and polymorphism, and appreciate their advantages in constructing complex embedded software architectures.
Resources such as online tutorials and open-source projects can be utilized, combined with practical small projects, such as designing a simple graphics drawing program, using classes and objects to organize code, gradually enhancing the ability to use C++.
1.2 Hardware Basics
Digital Circuits: Solidly learn the working principles of logic gate circuits (such as AND gates, OR gates, NOT gates, etc.), deeply study the state transition mechanisms of flip-flops (such as RS flip-flops, JK flip-flops, etc.), as well as the counting principles and application scenarios of counters (synchronous counters, asynchronous counters).
By practically building a digital circuit experimental platform, using breadboards and chips to assemble and test simple circuits, such as creating a simple digital clock circuit, personally experience the operation process of digital circuits to deepen the understanding and mastery of knowledge.
Computer Organization: Comprehensively understand the hardware structure of computers, including the collaborative working methods of components such as CPU, memory, hard disk, motherboard, etc.; deeply learn the encoding rules and execution processes of the instruction system, mastering the hierarchical structure of storage systems and the principles of data storage.
Teaching simulators for computer organization principles can be used to intuitively observe the data flow and instruction execution process within the computer, aiding in the understanding of abstract theoretical knowledge.
1.3 Data Structures and Algorithms
Data Structures: Seriously study the storage methods, operation methods, and characteristics of basic data structures such as arrays, linked lists, stacks, queues, trees (binary trees, balanced trees, etc.), and graphs. For example, for linked lists, deeply understand the principles of dynamic memory allocation and node linking, and be proficient in the code implementation of linked list operations such as insertion, deletion, and search; for tree structures, learn the traversal algorithms of binary trees (pre-order, in-order, post-order traversal) and their application scenarios.
1.4 Algorithms
Be proficient in common sorting algorithms (such as bubble sort, quick sort, merge sort, etc.), search algorithms (linear search, binary search, etc.), as well as the ideas and implementation methods of greedy algorithms.
Through online programming platforms (such as LeetCode, Niuke.com, etc.), practice a large number of algorithm exercises, continuously improve programming thinking ability and algorithm implementation ability, and learn to choose appropriate data structures and algorithms based on different problem scenarios to optimize the performance and resource utilization of embedded software.
1.5 Embedded Operating SystemsBasic Stage
Embedded Linux Operating System: First, master its basic concepts, including the meanings and functions of core elements such as kernel, file system, processes, and threads. Deeply study kernel architecture, understand the modular design of the kernel, process scheduling algorithms (such as Completely Fair Scheduler), and memory management mechanisms (virtual memory, page table management, etc.).
Learn the development methods in the embedded Linux environment, become proficient in using the GCC compiler for code compilation, and learn to write Makefile files to manage the compilation process of projects, achieving modular organization and efficient compilation of code.
References such as “Embedded Linux Application Development Complete Manual” can be used to set up an embedded Linux development environment in a virtual machine and develop some simple applications, such as writing a command-line tool based on Linux to perform file read and write operations, gradually becoming familiar with the development process and technical points of embedded Linux.
02
2.1 Using Development Tools
Integrated Development Environment (IDE): Become proficient in commonly used embedded development IDEs such as Keil and IAR. In Keil, learn to create projects, configure project options (such as selecting chip models, setting compilation optimization levels, etc.), write and debug code; in IAR, understand its unique project organization methods and debugging features (such as hardware breakpoints, real-time variable viewing, etc.). Continuously familiarize yourself with various operational skills of the IDE through actual project development to improve development efficiency.
Hardware Debugging Tools: Learn to use JTAG debuggers for online debugging, understand the connection methods and working principles of their debugging interfaces, and be able to use the JTAG interface to download programs to target chips, perform step-by-step debugging, set breakpoints, etc., to observe the running state of the program and changes in variable values in real-time, quickly locate and solve logical errors and hardware issues in the program. Master the usage of logic analyzers, learn to set trigger conditions, capture and analyze the timing relationships of digital signals, such as analyzing the signal transmission process of communication buses like SPI and I2C, and troubleshoot communication faults.
2.2 Project Practice
During project implementation, not only can you deeply master the hardware interface programming of microcontrollers and sensor data processing skills, but you can also learn how to design reasonable control logic based on actual needs, improving your ability to solve practical problems.
Microcontroller-Based Temperature Control System:Starting from this simple project, choose a suitable microcontroller (such as 51 microcontroller or STM32 microcontroller), learn the interface circuit and driver program writing methods for temperature sensors (such as DS18B20), and implement the collection and processing of temperature data. By controlling relays or PWM signals, achieve automatic adjustment of heating or cooling equipment to reach the set temperature. During the project implementation, learn the entire process of circuit design, PCB drawing, program development, and debugging, accumulating practical hardware development experience.
Embedded Linux-Based Smart Home Control System:After gaining a certain foundation in embedded Linux development, participate in this more complex project. This project involves data collection and fusion from various sensors (such as temperature and humidity sensors, light sensors, human infrared sensors, etc.), and through network communication (such as Wi-Fi, Ethernet), transmits data to a server or mobile APP for remote monitoring and control. Learn how to drive various sensors in the embedded Linux system, how to build a network communication framework (such as using socket programming to implement TCP/IP communication), and how to design a user-friendly mobile APP interface (which can use Android or iOS development platforms). Through this project, comprehensively enhance the overall development capability of embedded systems and deeply understand the technical applications and needs in the smart home field.
Embedded Linux-Based Smart Security Monitoring System:Using an embedded development board with camera interfaces, such as Raspberry Pi, running the embedded Linux operating system.Learn how to drive cameras (such as USB cameras or CSI interface cameras) under Linux to obtain real-time video stream data.
Utilize image processing libraries (such as OpenCV) to analyze and process video images, implementing target detection functions, such as detecting human silhouettes, vehicles, and other moving objects in the frame, and issuing alarm signals when abnormal situations are detected (which can be achieved by connecting a buzzer).
Through network programming, transmit video data to a remote server or mobile client, enabling remote monitoring functions. Users can view monitoring images in real-time through a mobile APP or web interface and perform parameter settings and control operations for the system, such as adjusting the camera’s shooting angle and switching resolution.
This project involves the comprehensive application of multiple technical fields such as hardware drivers, image processing, network communication, and mobile application development under the embedded Linux system, which can greatly enhance the understanding and practical development capabilities of embedded systems, while also keeping up with the technical demands of the popular application field of smart security.
04
4.1 Driver Development
Linux Kernel Driver Model:Deeply study the driver framework of the Linux kernel, including the registration and unregistration mechanisms of device drivers, allocation and management of device numbers, and the interface functions between drivers and the kernel.
Master the development methods of character device drivers to implement basic read and write operations for devices, such as developing a simple character device driver for controlling a custom hardware device (such as GPIO expansion chips);
Learn the principles and development processes of block device drivers, understand the characteristics of data caching and disk I/O operations, and simulate a simple block device driver to implement data storage and retrieval;
Master the key points of network device driver development, understand the sending and receiving processes of network packets, and the interaction methods between drivers and the network protocol stack; try to develop a simple virtual network device driver to implement the transmission and reception of network data.
4.2 Real-Time Operating Systems
VxWorks:Learn its priority-based preemptive task scheduling algorithm, understand the operations for creating, deleting, suspending, and resuming tasks, master the synchronization and communication mechanisms between tasks (such as semaphores, message queues, event flags, etc.), and the processes and mechanisms of interrupt handling.By reading the official documentation and related books of VxWorks, combined with actual development boards for project development, such as developing a real-time data acquisition and processing system to ensure the system completes data acquisition, processing, and transmission tasks within the specified time, meeting real-time requirements.
uC/OS-II:Deeply study the task scheduling principles of its kernel, understand the calculation methods of its minimum task stack and the allocation strategies of task priorities.Learn the various system services it provides (such as time management, memory management, etc.), and master the techniques for multi-task programming under uC/OS-II, such as implementing collaborative work between multiple tasks, and achieving synchronization and communication between tasks through semaphores and message mailboxes.References such as “Embedded Real-Time Operating System uC/OS-II” can be used for practical project development to enhance application capabilities of real-time operating systems.
4.3 Advanced Application Development
Network Programming:Deeply study the principles and applications of the TCP/IP protocol stack, master the usage of socket programming interfaces, and implement reliable data communication between embedded devices and servers or other network devices.Learn concurrent processing techniques in network programming, such as using multi-threading or select/poll/epoll mechanisms to achieve efficient network data processing.
You can develop a network server program and a corresponding embedded client program to achieve bidirectional transmission and interaction of data, such as remotely controlling the functions of embedded devices.
Graphical User Interface Development:Learn the usage of embedded graphical libraries (such as Qt, MiniGUI, etc.), master the creation and layout of basic elements of graphical interfaces (such as windows, buttons, text boxes, etc.), and understand graphical drawing functions and event handling mechanisms.
By designing an embedded application program with a graphical interface, such as a human-computer interaction interface for smart home control terminals, enhance user experience and product usability.
05
5.1 Focus on Industry Trends
Always pay attention to new technologies and trends in the embedded field, such as the application of artificial intelligence in embedded systems, including the application of machine learning algorithms in intelligent sensor data processing, and the embedded implementation of deep learning models in fields such as image recognition and speech recognition.
Regularly read technical articles and analysis reports from industry experts to understand the latest technological developments and changes in market demand.
Actively participate in various industry conferences (such as the Global Embedded Technology Conference, China International Embedded Conference, etc.) and technical seminars to communicate and learn from industry experts and corporate representatives, broadening technical horizons and grasping industry development directions.
5.2 Learn Knowledge in Related Fields
Based on personal interests and career development plans, learn knowledge in related fields such as the Internet of Things, artificial intelligence, and robotics.In the Internet of Things field, learn about sensor network technologies, wireless communication protocols (such as LoRa, NB-IoT, etc.), and the access and application development of IoT cloud platforms; you can develop a simple IoT application project, such as a remote environmental monitoring system, to combine embedded devices with IoT technology for remote data collection and management;
In the field of artificial intelligence, learn the basic algorithms of machine learning (such as linear regression, decision trees, neural networks, etc.) and their optimization and implementation methods on embedded platforms, understanding how to use embedded devices for intelligent data analysis and decision-making;
In the field of robotics, learn the principles of motion control, sensor fusion technology, human-computer interaction technology, etc.; participate in robotics development projects, such as the control system development of small intelligent robots, applying embedded technology to the core control part of robots, enhancing the intelligence level and performance of robots.
Through cross-domain learning and practice, continuously broaden knowledge and enhance comprehensive competitiveness in the embedded field, laying a solid foundation for future career development.
END
Due to recent changes in the WeChat public platform push rules, many readers have reported not seeing updated articles in a timely manner. According to the latest rules, it is recommended to frequently click on “Recommended Reading, Share, Collect,” etc., to become a regular reader.
Recommended Reading:
-
Factory under intensive investigation, BYD angrily responds!
-
Yushu Robot “flips over,” convulsing after falling!
-
Breaking! Nezha Automobile CEO resigns to join a new company
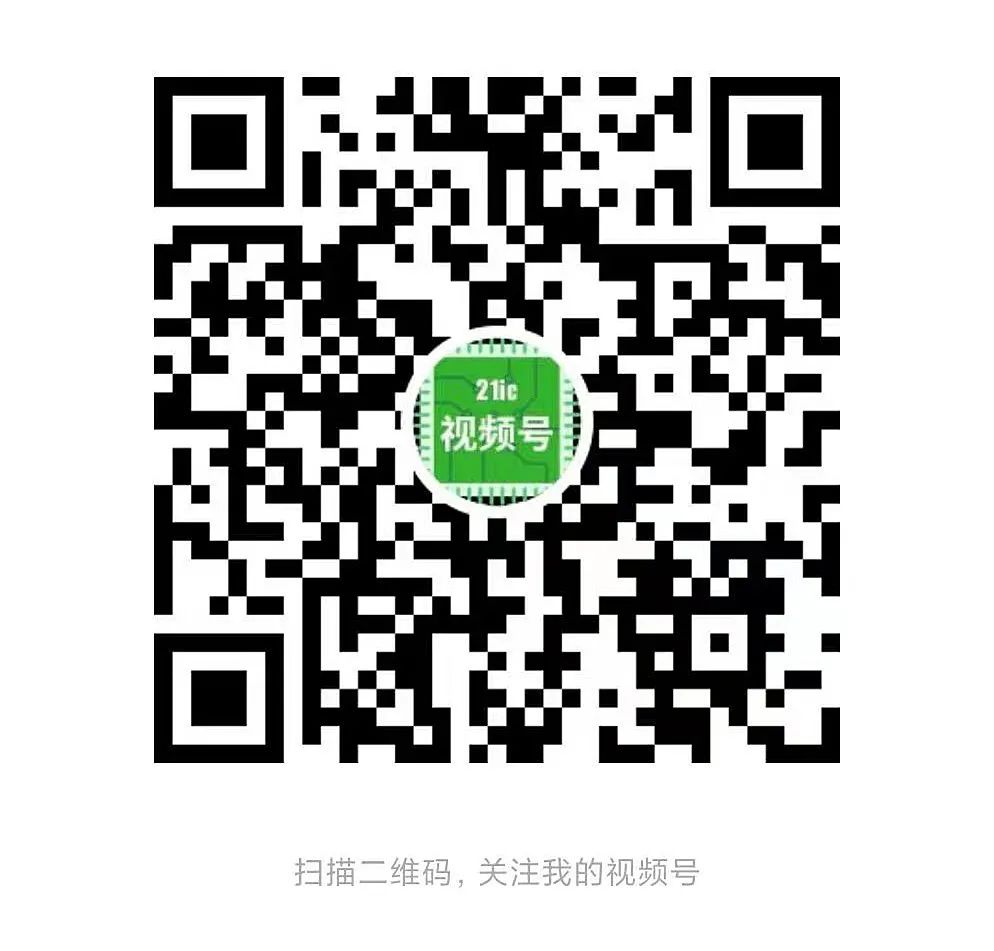
Please click 【See】 to give the editor a thumbs up
