Follow+Star Public Number, don’t miss out on exciting content
Source | Embedded Miscellaneous
Today we will introduce the design of testable software in embedded systems.
What is testability? It means that after you write the software module/function interface, you can conveniently and comprehensively perform self-testing.
Let’s look at a simple example to understand testable software.
There is a calculation function
cal_func
whose calculation depends on dataa
stored in flash memory and an external input datab
.
At this point, there are two implementation methods:
Method One:
int get_a_from_flash(void)
{
int a = 0;
flash_read(&a, sizeof(int));
return a;
}
int cal_func(int b)
{
int res = 0;
int a = get_a_from_flash();
res = a + b;
return res;
}
// Call
cal_func(5);
Method Two:
int get_a_from_flash(void)
{
int a = 0;
flash_read(&a, sizeof(int));
return a;
}
int cal_func(int a, int b)
{
int res = 0;
res = a + b;
return res;
}
// Call
cal_func(get_a_from_flash(), 5);
In practical development, there should be quite a few similar scenarios. Do you usually write code in Method One or Method Two?
From the perspective of testability, Method Two's implementation is more testable
.
In Method One, since one of the data is read from flash memory inside the function, it is not convenient for us to control this data, and we can only control parameter b
. Therefore, when we perform the test call, the results are not very comprehensive, and we cannot flexibly control the test path.
In Method Two, all data dependencies for the calculation are passed through function parameters, allowing us to conveniently test the function and input different data combinations.
Moreover, generally, we will introduce some unit testing frameworks
to uniformly manage our test cases.
Common testing frameworks in embedded systems include:
-
Unity: https://github.com/ThrowTheSwitch/Unity/releases -
cutest: https://sourceforge.net/projects/cutest/ -
embunit: https://sourceforge.net/projects/embunit -
googletest: https://github.com/google/googletest/releases
After using the testing framework, the test code designed for the cal_func
function is as follows:
int ut_cal_func(int argc, char *argv[])
{
if (argc != 3)
{
printf("Param num err\n");
return USAGE;
}
// Expected result
int expected_res = atoi(argv[2]);
// Actual result
int res = cal_func(atoi(argv[0]), atoi(argv[1]));
if (expected_res == res)
{
printf("input %d, %d, test pass!\n", atoi(argv[0]), atoi(argv[1]));
}
else
{
printf("input %d, %d, test failed!\n", atoi(argv[0]), atoi(argv[1]));
}
return 0;
}
We encapsulate it into serial port testing commands:
// Test path 1
ut app ut_cal_func 1 2 3
// Test path 2
ut app ut_cal_func 2 3 5
// ...
Output:
input 1, 2, test pass!
input 2, 3, test pass!
This is a small example of designing testable software. Through this example, everyone should recognize the benefits of testable software.
So, when writing code in the future, it is necessary to think carefully about how this module will be self-tested and what aspects need to be tested.
Software with strong testability allows us to conduct thorough testing during the development phase and resolve as many logical issues as possible, ensuring higher quality software delivery.
Disclaimer: The materials in this article are sourced from the internet, and the copyright belongs to the original author. If there are any copyright issues, please contact me for removal.
———— END ————
● Column “Embedded Tools”
● Column “Embedded Development”
● Column “Keil Tutorial”
● Selected Tutorials in Embedded Column
Follow the public account reply “Join Group” to join the technical exchange group according to the rules, reply “1024” to see more content.
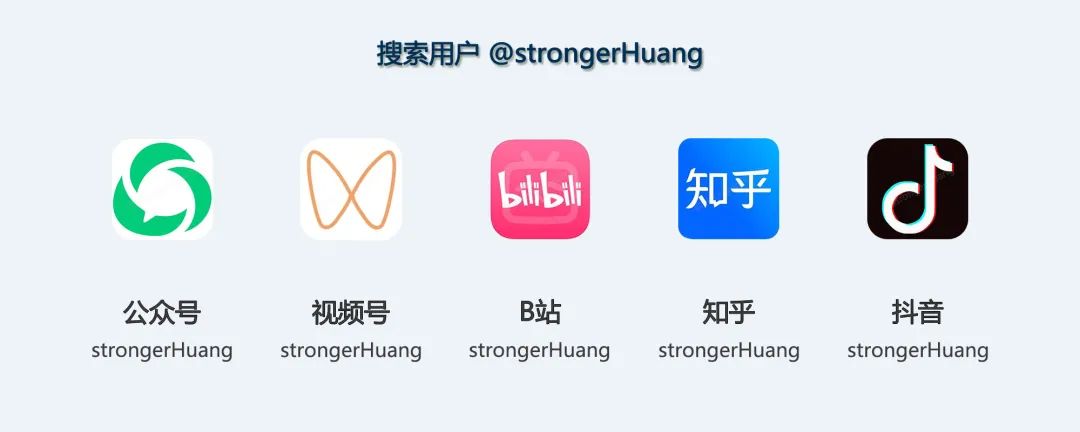
Click “Read the Original” to see more shares.