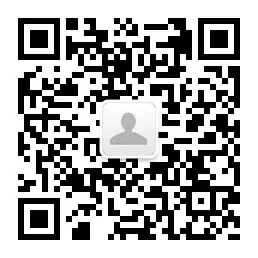
Author丨LYing_
http://www.apkbus.com/blog-685845-76823.html
As a new driver in the programmer community, it’s time to organize some things. Over the past two or three years, I have written code while forgetting it, losing it along the way. Many issues have been forgotten in the hustle and bustle, so I decided to write some notes for myself to reflect during my spare time. Readers in need can also take a glance; if there are mistakes or issues, feel free to point them out, although I may not necessarily correct them, O(∩_∩)O haha~
In daily development, many things are written countless times. I personally do not have the habit of rewriting every time (I wonder if there are any friends who are this straightforward??) Therefore, organizing my utility classes is still necessary. Here I will record a few that I have used more frequently so far.
Common Utility Classes
Here’s also a piece of code for calculating the actual distance between two points based on latitude and longitude. Generally, we directly use the methods included in the integrated third-party map SDK, but I will still provide the code.
/** * Supplement: Calculate the actual distance between two points * * @return meters */ public static double getDistance(double longitude1, double latitude1, double longitude2, double latitude2) { // Latitude double lat1 = (Math.PI / 180) * latitude1; double lat2 = (Math.PI / 180) * latitude2; // Longitude double lon1 = (Math.PI / 180) * longitude1; double lon2 = (Math.PI / 180) * longitude2; // Radius of the Earth double R = 6371; // Distance between the two points in km. To get meters, multiply the result by 1000. double d = Math.acos(Math.sin(lat1) * Math.sin(lat2) + Math.cos(lat1) * Math.cos(lat2) * Math.cos(lon2 - lon1)) * R; return d * 1000; }
Common File Classes
The code for file classes is quite extensive, so I will only provide the code for reading and writing files.
/** * Check if the SD card is available * @return returns true if the SD card is available */ public static boolean hasSdcard() { String status = Environment.getExternalStorageState(); return Environment.MEDIA_MOUNTED.equals(status); } /** * Read the content of the file * <br> * Default utf-8 encoding * @param filePath file path * @return string * @throws IOException */ public static String readFile(String filePath) throws IOException { return readFile(filePath, "utf-8"); } /** * Read the content of the file * @param filePath file directory * @param charsetName character encoding * @return String string */ public static String readFile(String filePath, String charsetName) throws IOException { if (TextUtils.isEmpty(filePath)) return null; if (TextUtils.isEmpty(charsetName)) charsetName = "utf-8"; File file = new File(filePath); StringBuilder fileContent = new StringBuilder(""); if (file == null || !file.isFile()) return null; BufferedReader reader = null; try { InputStreamReader is = new InputStreamReader(new FileInputStream(file), charsetName); reader = new BufferedReader(is); String line = null; while ((line = reader.readLine()) != null) { if (!fileContent.toString().equals("")) { fileContent.append("\n"); } fileContent.append(line); } return fileContent.toString(); } finally { if (reader != null) { try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } } /** * Read text file into a List of strings (default utf-8 encoding) * @param filePath file directory * @return returns null if the file does not exist, otherwise returns a string collection * @throws IOException */ public static List<string> readFileToList(String filePath) throws IOException { return readFileToList(filePath, "utf-8"); } /** * Read text file into a List of strings * @param filePath file directory * @param charsetName character encoding * @return returns null if the file does not exist, otherwise returns a string collection */ public static List<string> readFileToList(String filePath, String charsetName) throws IOException { if (TextUtils.isEmpty(filePath)) return null; if (TextUtils.isEmpty(charsetName)) charsetName = "utf-8"; File file = new File(filePath); List<string> fileContent = new ArrayList<>(); if (file == null || !file.isFile()) { return null; } BufferedReader reader = null; try { InputStreamReader is = new InputStreamReader(new FileInputStream(file), charsetName); reader = new BufferedReader(is); String line = null; while ((line = reader.readLine()) != null) { fileContent.add(line); } return fileContent; } finally { if (reader != null) { try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } } /** * Write data to a file * @param filePath file directory * @param content content to be written * @param append if true, the data will be written to the end of the file instead of the beginning * @return returns true if the write is successful, returns false if it fails * @throws IOException */ public static boolean writeFile(String filePath, String content, boolean append) throws IOException { if (TextUtils.isEmpty(filePath)) return false; if (TextUtils.isEmpty(content)) return false; FileWriter fileWriter = null; try { createFile(filePath); fileWriter = new FileWriter(filePath, append); fileWriter.write(content); fileWriter.flush(); return true; } finally { if (fileWriter != null) { try { fileWriter.close(); } catch (IOException e) { e.printStackTrace(); } } } } /** * Write data to a file<br> * Default writes data to the beginning of the file * @param filePath file directory * @param stream byte input stream * @return returns true if the write is successful, otherwise returns false * @throws IOException */ public static boolean writeFile(String filePath, InputStream stream) throws IOException { return writeFile(filePath, stream, false); } /** * Write data to a file * @param filePath file directory * @param stream byte input stream * @param append if true, the data will be written to the end of the file; if false, the original data will be cleared and written from the beginning * @return returns true if the write is successful, otherwise returns false * @throws IOException */ public static boolean writeFile(String filePath, InputStream stream, boolean append) throws IOException { if (TextUtils.isEmpty(filePath)) throw new NullPointerException("filePath is Empty"); if (stream == null) throw new NullPointerException("InputStream is null"); return writeFile(new File(filePath), stream, append); } /** * Write data to a file * Default writes data to the beginning of the file * @param file specified file * @param stream byte input stream * @return returns true if the write is successful, otherwise returns false * @throws IOException */ public static boolean writeFile(File file, InputStream stream) throws IOException { return writeFile(file, stream, false); } /** * Write data to a file * @param file specified file * @param stream byte input stream * @param append if true, data will be written to the beginning; if false, the original data will be cleared and written from the beginning * @return returns true if the write is successful, otherwise returns false * @throws IOException */ public static boolean writeFile(File file, InputStream stream, boolean append) throws IOException { if (file == null) throw new NullPointerException("file = null"); OutputStream out = null; try { createFile(file.getAbsolutePath()); out = new FileOutputStream(file, append); byte data[] = new byte[1024]; int length = -1; while ((length = stream.read(data)) != -1) { out.write(data, 0, length); } out.flush(); return true; } finally { if (out != null) { try { out.close(); stream.close(); } catch (IOException e) { e.printStackTrace(); } } } }</string></string></string>
Date Utility Class
This one is quite commonly used.
/** * Convert long time to yyyy-MM-dd HH:mm:ss string<br> * @param timeInMillis time long value * @return yyyy-MM-dd HH:mm:ss */ public static String getDateTimeFromMillis(long timeInMillis) { return getDateTimeFormat(new Date(timeInMillis)); } /** * Convert date to yyyy-MM-dd HH:mm:ss string * <br> * @param date Date object * @return yyyy-MM-dd HH:mm:ss */ public static String getDateTimeFormat(Date date) { return dateSimpleFormat(date, defaultDateTimeFormat.get()); } /** * Convert year, month, day int to yyyy-MM-dd string * @param year year * @param month month 1-12 * @param day day * Note: month represents Calendar month, which is one less than actual * No input item checks were made */ public static String getDateFormat(int year, int month, int day) { return getDateFormat(getDate(year, month, day)); } /** * Get HH:mm:ss time * @param date * @return */ public static String getTimeFormat(Date date) { return dateSimpleFormat(date, defaultTimeFormat.get()); } /** * Format date display format * @param sdate original date format "yyyy-MM-dd" * @param format formatted date format * @return formatted date display */ public static String dateFormat(String sdate, String format) { SimpleDateFormat formatter = new SimpleDateFormat(format); java.sql.Date date = java.sql.Date.valueOf(sdate); return dateSimpleFormat(date, formatter); } /** * Format date display format * @param date Date object * @param format formatted date format * @return formatted date display */ public static String dateFormat(Date date, String format) { SimpleDateFormat formatter = new SimpleDateFormat(format); return dateSimpleFormat(date, formatter); } /** * Convert date to string * @param date Date * @param format SimpleDateFormat * <br> * Note: If SimpleDateFormat is null, use the default format yyyy-MM-dd HH:mm:ss * @return yyyy-MM-dd HH:mm:ss */ public static String dateSimpleFormat(Date date, SimpleDateFormat format) { if (format == null) format = defaultDateTimeFormat.get(); return (date == null ? "" : format.format(date)); } /** * Convert string in "yyyy-MM-dd HH:mm:ss" format to Date * @param strDate time string * @return Date */ public static Date getDateByDateTimeFormat(String strDate) { return getDateByFormat(strDate, defaultDateTimeFormat.get()); } /** * Convert string in "yyyy-MM-dd" format to Date * @param strDate * @return Date */ public static Date getDateByDateFormat(String strDate) { return getDateByFormat(strDate, defaultDateFormat.get()); } /** * Convert time string in specified format to Date object * @param strDate time string * @param format format string * @return Date */ public static Date getDateByFormat(String strDate, String format) { return getDateByFormat(strDate, new SimpleDateFormat(format)); } /** * Convert String string to Date according to a certain format<br> * Note: If SimpleDateFormat is null, use the default format yyyy-MM-dd HH:mm:ss * @param strDate time string * @param format SimpleDateFormat object * @exception ParseException date format conversion error */ private static Date getDateByFormat(String strDate, SimpleDateFormat format) { if (format == null) format = defaultDateTimeFormat.get(); try { return format.parse(strDate); } catch (ParseException e) { e.printStackTrace(); } return null; } /** * Convert year, month int to date * @param year year * @param month month 1-12 * @param day day * Note: month represents Calendar month, which is one less than actual */ public static Date getDate(int year, int month, int day) { Calendar mCalendar = Calendar.getInstance(); mCalendar.set(year, month - 1, day); return mCalendar.getTime(); } /** * Calculate the number of days between two dates * * @param strat start date, format yyyy-MM-dd * @param end end date, format yyyy-MM-dd * @return number of days between the two dates */ public static long getIntervalDays(String strat, String end) { return ((java.sql.Date.valueOf(end)).getTime() - (java.sql.Date.valueOf(strat)).getTime()) / (3600 * 24 * 1000); } /** * Get the current year * @return year(int) */ public static int getCurrentYear() { Calendar mCalendar = Calendar.getInstance(); return mCalendar.get(Calendar.YEAR); } /** * Get the current month * @return month(int) 1-12 */ public static int getCurrentMonth() { Calendar mCalendar = Calendar.getInstance(); return mCalendar.get(Calendar.MONTH) + 1; } /** * Get the current day of the month * @return day(int) */ public static int getDayOfMonth() { Calendar mCalendar = Calendar.getInstance(); return mCalendar.get(Calendar.DAY_OF_MONTH); } /** * Get today's date (format: yyyy-MM-dd) * @return yyyy-MM-dd */ public static String getToday() { Calendar mCalendar = Calendar.getInstance(); return getDateFormat(mCalendar.getTime()); } /** * Get yesterday's date (format: yyyy-MM-dd) * @return yyyy-MM-dd */ public static String getYesterday() { Calendar mCalendar = Calendar.getInstance(); mCalendar.add(Calendar.DATE, -1); return getDateFormat(mCalendar.getTime()); } /** * Get the day before yesterday's date (format: yyyy-MM-dd) * @return yyyy-MM-dd */ public static String getBeforeYesterday() { Calendar mCalendar = Calendar.getInstance(); mCalendar.add(Calendar.DATE, -2); return getDateFormat(mCalendar.getTime()); } /** * Get the date a few days before or after * @param diff difference: positive for pushing forward, negative for pushing back * @return */ public static String getOtherDay(int diff) { Calendar mCalendar = Calendar.getInstance(); mCalendar.add(Calendar.DATE, diff); return getDateFormat(mCalendar.getTime()); } /** * Get the date object after adding a certain number of days to a given date. * * @param //date given date object * @param amount number of days to add; use a negative number for days before. * @return Date object after adding a certain number of days. */ public static String getCalcDateFormat(String sDate, int amount) { Date date = getCalcDate(getDateByDateFormat(sDate), amount); return getDateFormat(date); } /** * Get the date object after adding a certain number of days to a given date. * * @param date given date object * @param amount number of days to add; use a negative number for days before. * @return Date object after adding a certain number of days. */ public static Date getCalcDate(Date date, int amount) { Calendar cal = Calendar.getInstance(); cal.setTime(date); cal.add(Calendar.DATE, amount); return cal.getTime(); }
Most of it is code, not that I am lazy (just lazy, come at me), mainly to facilitate new drivers to copy, okay. That’s it for this note.
Recommended↓↓↓
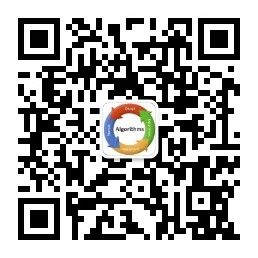
Long
Press
Close
Note
👉【16 Technical Public Accounts】are all here!
Covering: Programmer celebrities, source code reading, programmer reading, data structures and algorithms, hacker technology and network security, big data technology, front-end programming, Java, Python, Web programming development, Android, iOS development, Linux, database development, humorous programmers, etc.
