Hello everyone! I’m Cat Brother, and today I want to take you on an exciting journey to explore a super cool tool: ThingsBoard Gateway! It is an open-source IoT gateway that can easily connect various IoT devices to the ThingsBoard platform. Even better, it is developed using Python, which means we can leverage Python’s powerful features to customize and extend it! Isn’t that exciting? Let’s quickly embark on today’s Python learning journey together!
What is ThingsBoard Gateway?
ThingsBoard Gateway acts like a bridge, connecting various IoT devices to the ThingsBoard platform. It is responsible for collecting device data, performing protocol conversion, and securely transmitting data to the ThingsBoard platform. Imagine you have a bunch of sensors from different manufacturers, each speaking different “languages” (protocols), and ThingsBoard Gateway acts like a translator, converting their messages into a language that the ThingsBoard platform can understand, allowing the platform to manage and analyze this data uniformly!
Installing ThingsBoard Gateway
We need to install ThingsBoard Gateway. Don’t worry, the process is very simple!
1 sudo apt install python3 python3-pip # Install Python3 and pip
2 pip3 install thingsboard-gateway # Install ThingsBoard Gateway
Tip: Make sure your system has Python3 and pip installed. If not, you can use the command above to install them.
Configuring ThingsBoard Gateway
After installation, we need to configure the Gateway to specify which devices and the ThingsBoard platform it should connect to.
1{
2 "connectors": [
3 {
4 "name": "My Connector",
5 "type": "mqtt",
6 "configuration": {
7 "host": "mqtt.example.com",
8 "port": 1883
9 }
10 }
11 ],
12 "security": {
13 "accessToken": "YOUR_ACCESS_TOKEN"
14 }
15}
This configuration uses JSON format. The connectors
section defines the connector, where we use the MQTT protocol as an example. The security
section sets the access token for connecting to the ThingsBoard platform. Remember to replace YOUR_ACCESS_TOKEN
with your own access token!
Tip: ThingsBoard Gateway supports various connector types such as MQTT, Modbus, OPC-UA, etc. You can choose the appropriate connector based on your devices.
Extending ThingsBoard Gateway with Python
The most powerful aspect of ThingsBoard Gateway is its extensibility. We can write custom extensions using Python to handle various special requirements. We could write an extension to preprocess device data or implement a custom communication protocol.
1from thingsboard_gateway.tb_gateway_service import TBGatewayService
2
3class MyExtension:
4 def __init__(self, gateway):
5 self.gateway = gateway
6
7 def process_data(self, data):
8 # Preprocess the data
9 processed_data = data * 2
10 self.gateway.send_to_storage(processed_data)
11
12gateway = TBGatewayService()
13my_extension = MyExtension(gateway)
14gateway.add_extension(my_extension)
15gateway.run()
In this example, we created an extension named MyExtension
that multiplies the data by 2 before sending it to the ThingsBoard platform.
Note: When writing extensions, you need to follow the ThingsBoard Gateway API specifications.
Running ThingsBoard Gateway
Once configured, you can run the ThingsBoard Gateway.
1 thingsboard-gateway -c /path/to/your/config.json
Replace /path/to/your/config.json
with the path to your configuration file.
Conclusion
Today we learned how to install, configure, and extend the ThingsBoard Gateway. It is a powerful tool that can help us easily connect and manage IoT devices. I hope everyone can practice hands-on and experience the charm of ThingsBoard Gateway!
Friends, today’s Python learning journey ends here! Remember to get your hands on the code, and feel free to ask Cat Brother in the comments if you have any questions. Wish you all happy learning, and may your Python skills soar!
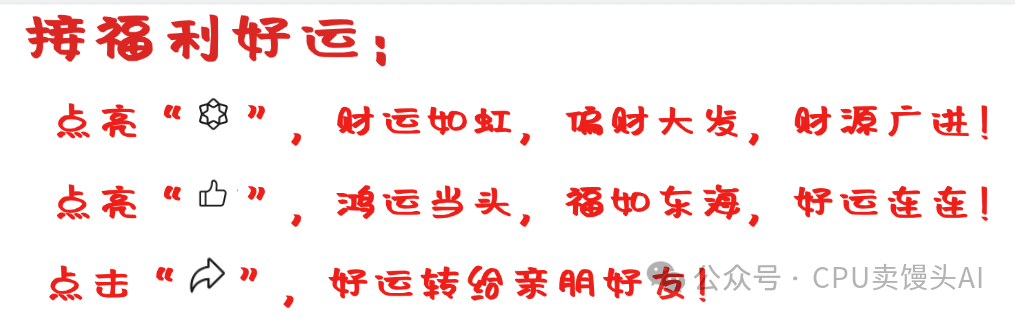