The const keyword in C is crucial, and today we will delve into the definition and practical applications of const so that it is no longer a mystery.
In C, the const keyword is an abbreviation for constant, meaning it remains unchanged. It is usually translated as constant, and when we see the const keyword, we immediately think of constants. This is not precise; more accurately, it should be considered a read-only variable, the value of which cannot be used during compilation because the compiler does not know what it stores at compile time. Thus, the initial purpose of introducing const was to replace preprocessor directives, eliminating their drawbacks while inheriting their advantages.
In fact, const is quite powerful in C; it can modify variables, arrays, pointers, function parameters, etc.
1. Read-only variables modified by const:
Using const to modify a variable in C declares it as a read-only variable, protecting its value from being modified.
For example:
const int Max = 100;
int Array[Max];
You can test this in Visual C++ 6.0 by creating a .c file, and you will see that the compiler will prompt an error. We know that defining an array requires specifying the number of its elements, which indirectly confirms that Max modified by const is still a variable, just with a read-only attribute.
It is also worth noting that when defining a variable, it must be initialized, and it cannot be reassigned.
2. Save space, avoid unnecessary memory allocation, and improve efficiency
The compiler typically does not allocate storage space for ordinary read-only variables modified by const, but instead keeps them in the symbol table, making them a value during compilation without storage and memory read operations, thus enhancing efficiency.
For example:
#define M 3 // Macro constant
const int N = 5; // At this point, N is not placed in memory
int i = N; // Now memory is allocated for N, no further allocation
int I = M; // Macro replacement occurs during pre-compilation, memory allocated
int j = N; // No memory allocation
int J = M; // Macro replacement again, memory allocated
From an assembly perspective, a read-only variable defined by const only provides the corresponding memory address, unlike #define which provides an immediate value. Thus, a read-only variable defined by const has only one backup during program execution (since it is a global read-only variable stored in the static area), while a macro constant defined by #define has several backups in memory. The #define macro is replaced during preprocessing, while the value of the read-only variable modified by const is determined at compile time. The #define macro has no type, while the read-only variable modified by const has a specific type.
3. Modifying general variables
General variables refer to simple types of read-only variables. This type of read-only variable can have the const modifier placed before or after the type specifier during definition.
For example:
int const i = 2; or const int i = 2;
4. Modifying arrays
In C, const can also modify arrays, as shown below:
const int array[5] = {1,2,3,4,5};
array[0] = array[0] + 1; // Error
Array elements, like variables, have read-only attributes and cannot be changed; any attempt to change them will result in an error.
5. Modifying pointers
When using const to modify pointers in C, special attention is needed; there are two forms: one restricts the value pointed to from being modified, while the other restricts the pointer itself from being changed. Here are some examples:
The principle of const modifying who is closest
For example:
const int * p1; // Definition 1, p1 is variable, the object pointed to by p1 is immutable
int * const p2; // Definition 2, p2 is immutable, the object pointed to by p2 is variable
The above defines two pointers, p1 and p2.
In Definition 1, const limits *p1, meaning the value of the pointed space cannot be changed; if we change the value of the pointed space, like *p1 = 20, the program will report an error; however, the value of p1 itself can be changed, such as p1 = &k, which is perfectly fine.
In Definition 2, const limits the pointer p2; if we change p2’s value like p2 = &k, the program will report an error; however, *p2, meaning the value of the pointed space, can be changed, such as *p2 = 80, and the program will execute normally.
6. Modifying function parameters
The const modifier can also modify function parameters when we do not want the parameter value to be accidentally changed within the function body. The limited function parameters can be ordinary variables or pointer variables.
For example:
void fun1(const int i){Other statements……i++; // Modified the value of i, the program reports an errorOther statements}
This tells the compiler that i cannot change within the function body, preventing some unintentional or erroneous modifications by the user.
void fun2(const int *p){Other statements……(*p)++; // Modified the value pointed to by p, the program reports an errorOther statements}
7. Modifying function return values
The const modifier can also modify function return values, making the return value unchangeable.
const int Fun(void);
At this point, I have described the definition and common explanations of const. If you have questions or other thoughts, feel free to communicate!
Previous reviews:
● Illustrated edge alignment, center alignment PWM…
● What? The programmer cannot connect to the STM32 microcontroller, don’t panic, the bootstrap mode will help
● STM32 GPIO Learning Design Chapter
END
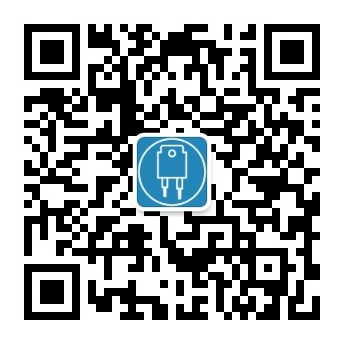
