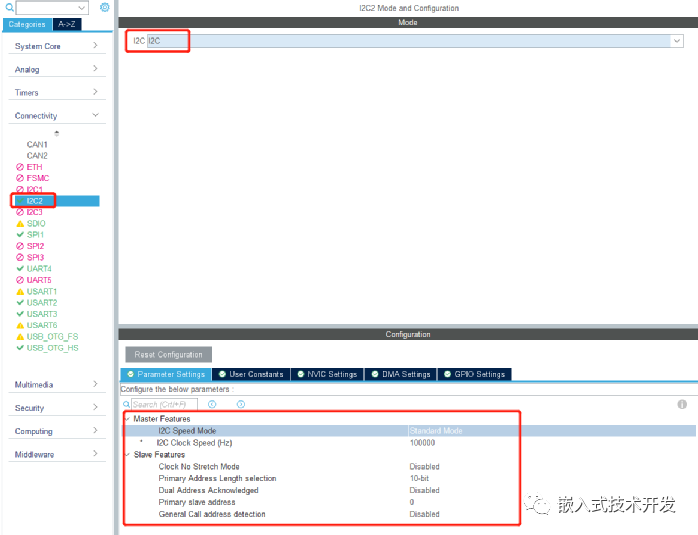
HAL_I2C_Mem_Write
The function parameters can be referenced in the code comments.
#include "at24c64.h"
#include "i2c.h"
#define AT24CXX_ADDR_READ 0xA1
#define AT24CXX_ADDR_WRITE 0xA0
#define PAGE_SIZE 32
/** * @brief Read multiple bytes of data from any address of AT24C64 * @param addr —— Address to read data (0-65535) * @param dat —— Address to store read data * @retval Success —— HAL_OK*/
uint8_t At24cxx_Read_Amount_Byte(uint16_t addr, uint8_t* recv_buf, uint16_t size){
return HAL_I2C_Mem_Read(&hi2c2, AT24CXX_ADDR_READ, addr, I2C_MEMADD_SIZE_16BIT, recv_buf, size, 0xFFFFFFFF);
}
/** * @brief Write multiple bytes of data to any address of AT24C64 * @param addr —— Address to write data (0-65535) * @param dat —— Address to store written data * @retval Success —— HAL_OK*/
uint8_t At24cxx_Write_Amount_Byte(uint16_t addr, uint8_t* dat, uint16_t size){
uint8_t i = 0;
uint16_t cnt = 0;
// Count of bytes written
/* For the starting address, there are two cases to consider */
if(0 == addr % PAGE_SIZE ) {
/* Starting address is exactly the page start address */
/* For the number of bytes to write, there are two cases to consider */
if(size <= PAGE_SIZE) {
// Number of bytes to write is not greater than one page, write directly
return HAL_I2C_Mem_Write(&hi2c2, AT24CXX_ADDR_WRITE, addr, I2C_MEMADD_SIZE_16BIT, dat, size, 0xFFFFFFFF);
}
else {
// Number of bytes to write is greater than one page, write the whole page in a loop
for(i = 0;i < size/PAGE_SIZE; i++) {
HAL_I2C_Mem_Write(&hi2c2, AT24CXX_ADDR_WRITE, addr, I2C_MEMADD_SIZE_16BIT, &dat[cnt], PAGE_SIZE, 0xFFFFFFFF);
HAL_Delay(3);
addr += PAGE_SIZE;
cnt += PAGE_SIZE;
}
// Write the remaining bytes
return HAL_I2C_Mem_Write(&hi2c2, AT24CXX_ADDR_WRITE, addr, I2C_MEMADD_SIZE_16BIT, &dat[cnt], size - cnt, 0xFFFFFFFF);
}
}
else {
/* Starting address deviates from the page start address */
/* For the number of bytes to write, there are two cases to consider */
if(size <= (PAGE_SIZE - addr%PAGE_SIZE)) {
/* Can write all in this page */
return HAL_I2C_Mem_Write(&hi2c2, AT24CXX_ADDR_WRITE, addr, I2C_MEMADD_SIZE_16BIT, dat, size, 0xFFFFFFFF);
}
else {
/* This page cannot be fully written */
// First finish writing this page
cnt += PAGE_SIZE - addr%PAGE_SIZE;
HAL_I2C_Mem_Write(&hi2c2, AT24CXX_ADDR_WRITE, addr, I2C_MEMADD_SIZE_16BIT, dat, cnt, 0xFFFFFFFF);
addr += cnt;
HAL_Delay(3);
// Loop to write whole page data
for(i = 0;i < (size - cnt)/PAGE_SIZE; i++) {
HAL_I2C_Mem_Write(&hi2c2, AT24CXX_ADDR_WRITE, addr, I2C_MEMADD_SIZE_16BIT, &dat[cnt], PAGE_SIZE, 0xFFFFFFFF);
HAL_Delay(3);
addr += PAGE_SIZE;
cnt += PAGE_SIZE;
}
// Write the remaining bytes
return HAL_I2C_Mem_Write(&hi2c2, AT24CXX_ADDR_WRITE, addr, I2C_MEMADD_SIZE_16BIT, &dat[cnt], size - cnt, 0xFFFFFFFF);
} }
}