Abstract:Embedded system design requires not only an understanding of hardware but also an understanding of how software operates and interacts with it. The paradigm needed for hardware design may be completely opposite to that of software design. When transitioning from hardware design to software-inclusive design, hardware and software engineers should keep the following ten tips in mind.
1. Flowchart First, Implement Second
When engineers first step into the field of software development, there is a strong temptation to dive right in and start writing code.
This fixed mindset is akin to trying to design a printed circuit board (PCB) before the circuit logic diagram is complete. It is crucial to suppress the urge to write code when developing software and to first create a software architecture diagram using a flowchart.
This approach allows developers to conceptualize the different parts and components needed for the application, just as a circuit logic diagram can inform engineers of the necessary hardware components. This ensures that the program is built on a solid organization and thoughtful consideration, reducing debugging time and saving time and trouble in the long run.
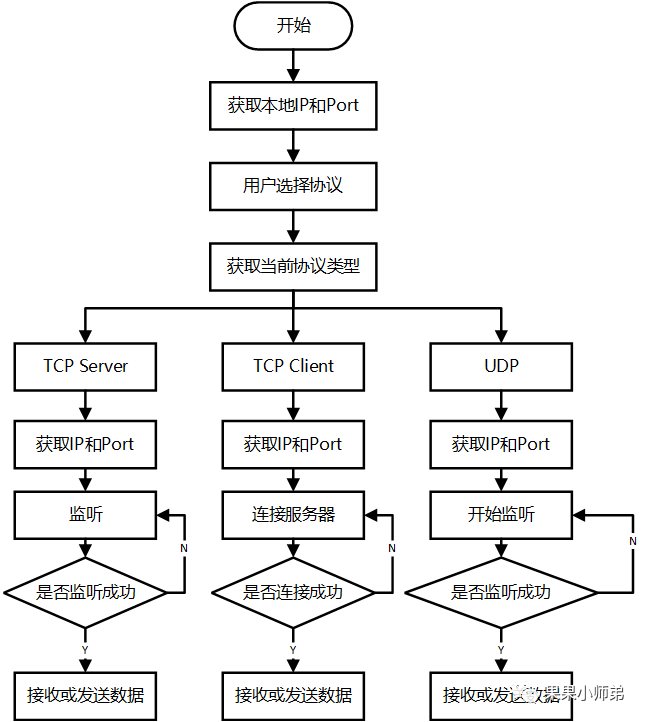
2. Use State Machines to Control Program Flow
State machines are one of the greatest software inventions of the 20th century. An application can often be divided into multiple state machines, each controlling a specific part of the application. These state machines have their own internal states and state transitions, showing how the software interacts with various stimuli.
Designing software with state machines can simplify development, making it modular, maintainable, and easy to understand. There are extensive resources available that demonstrate the theory and algorithms of state machines.
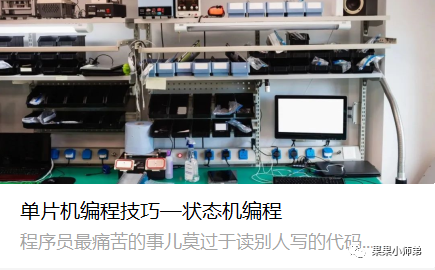
3. Avoid Using Global Variables
In embedded systems, especially in os-less microcontroller programs, the most common mistake is the rampant use of global variables. This phenomenon is common among early assembly-transformed programmers and beginners, who often treat global variables as function parameters.
Defining many chaotic structures in .h documents, externing a bunch of hair-raising global variables, and then assigning values of 123 in this module and judging branches in that module based on 123.
While the importance of global variables is not denied, they must be used with caution; misuse can lead to other more serious structural system issues.
-
It can cause unnecessary constants to be used frequently, especially when this constant is not “renamed” with a macro definition, making the code very difficult to read.
-
It can lead to unreasonable software layering; global variables act as a shortcut, easily blurring the boundaries between the “device layer” and the “application layer.” Lower-level programs can easily become overly concerned with upper-level applications. While this indeed improves efficiency during the early stages of software system construction, it often leads to a pile of bugs and patches in the later stages. Saying that it is difficult to make progress is not an exaggeration.
-
Due to unreasonable software layering, later maintenance, even for small modifications, often requires digging through most modules from top to bottom, while the original code comments are often forgotten to be updated, making the system increasingly resemble a “quagmire,” with comments only serving to add more confusion above the mire.
-
Excessive use of global variables can lead to some variables lingering between interrupts and the main loop program. If not handled properly, system bugs can appear randomly and irregularly, showing early signs of severe issues. Without a big player to save the day, it is doomed to slow death.
Two Principles and Countermeasures
-
Avoid using global variables whenever possible. Except for system states, control parameters, communication handling, and some efficiency-required modules, others can basically rely on reasonable software layering and programming techniques to solve.
-
If unavoidable, hide them as much as possible.
1) If only used in a certain .c file, make it static to that file, along with the structure definition;
2) If only one function uses it, make it static within that function;
3) If it must be exposed for reading, return it via a function, making it read-only;
4) If it must be assigned a value, expose a function interface to pass parameters for assignment;
5) If it must be externed, strictly control the objects that include the .h file, rather than placing it in a public includes.h file for everyone to see, which is embarrassing.
Note: Avoiding use does not mean forbidding it!
4. Leverage the Benefits of Modularity
Regardless of which engineer you ask, which part of the project is most likely to be delayed and exceed budget? The answer is always software. Software is often complex, difficult to develop and maintain, especially when the entire application exists in a single file or loosely associated multiple files. To alleviate maintainability, reusability, and complexity, programmers are strongly encouraged to take full advantage of the modular features of modern programming languages, breaking common functions into modules.
By decomposing code in this way, programmers can begin to build libraries of functions and features that can be reused across applications, improving code quality through continuous testing while also saving time and reducing development costs.
5. Keep Interrupt Service Routines Simple
Interrupt service routines are used to interrupt the processor’s execution of the current code branch to handle the peripheral device that just triggered the interrupt. Whenever an interrupt is executed, a certain amount of overhead is required to save the current program’s state, run the interrupt, and then return the processor to the original program state.
Modern processors are much faster than those from years ago, but this overhead still needs to be considered. Generally speaking, programmers want to minimize interrupt run time to avoid interfering with the main code branch. This means that interrupts should be short and simple.
Functions should not be called within interrupts. Additionally, if an interrupt starts to become too complex or time-consuming, it should only do minimal work when necessary, such as loading data into a buffer and setting a flag, then letting the main branch handle the input data. This ensures that most processor cycles are used to run the application rather than handling interrupts.
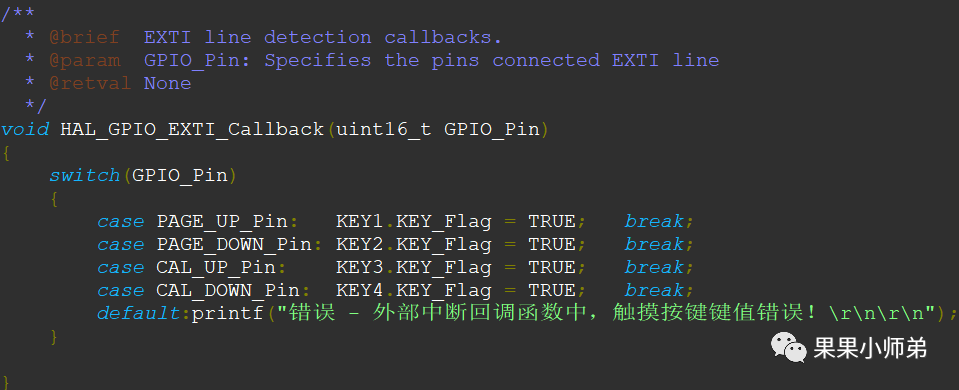
6. Use Sample Code to Experiment with Peripherals
When designing hardware, it is always beneficial to prototype test circuits to ensure engineers have a correct understanding of the circuit before proceeding with PCB layout. This point applies equally to software design. Chip manufacturers often provide sample code to test various parts of the microprocessor, allowing engineers to determine the functionality of that part.
This approach provides insight into how the software architecture should be organized and any potential issues that may arise. Identifying potential obstacles in the early stages of design is better than discovering them in the final hours before product delivery.
This is a great way to pre-test code snippets, but it should be noted that manufacturer code is often not modular and inconvenient for practical applications without significant modifications. This limitation has changed over time, and perhaps one day chip suppliers will provide code suitable for production.
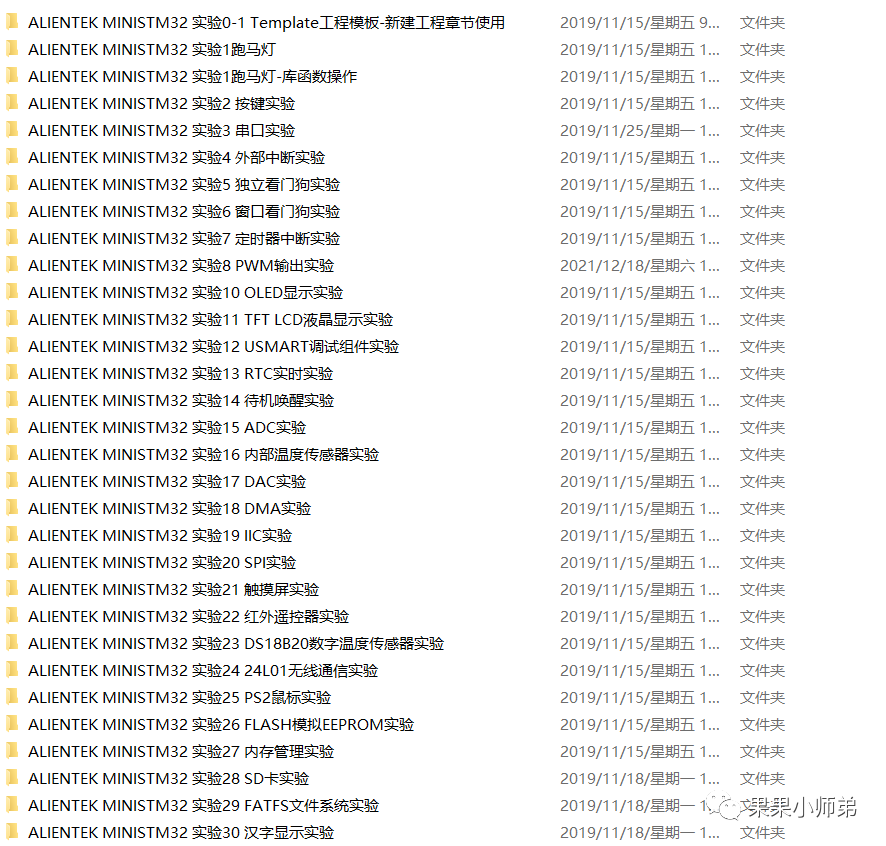
7. Limit Function Complexity
There is an old term in engineering called “KISS” – Keep It Simple and Straightforward. Regardless of the complexity of the task at hand, the simplest approach is to break it down into smaller, simpler, and more manageable tasks. As tasks or functions become more complex, it becomes increasingly difficult to accurately document all the details.
When writing a function, its complexity may seem moderate at the time, but consider how an engineer will review the code six months later. There are many ways to measure function complexity (such as loop complexity). There are now tools available that can automatically calculate the cyclomatic complexity of a function. The rule of thumb suggests that keeping the cyclomatic complexity of a function below 10 is ideal.
Regardless of the complexity of the task at hand, the simplest approach is to break it down into more manageable tasks.
8. Use Source Code Repositories
Humans make mistakes, and errors occur during coding. This is why it is so important for developers to use source code repositories. Source code repositories allow developers to “register” a good version of the code and describe the modifications made to that code. This step not only allows developers to revert to or trace back to an older version of the code but also to compare differences between old versions.
If a series of changes made by a developer breaks the system, restoring the good version of the code is just a click away! Please remember that if code is not submitted frequently, the repository will not serve its intended purpose. If irreversible modifications are made and the code is submitted two weeks later, restoring it will result in significant work and time loss!
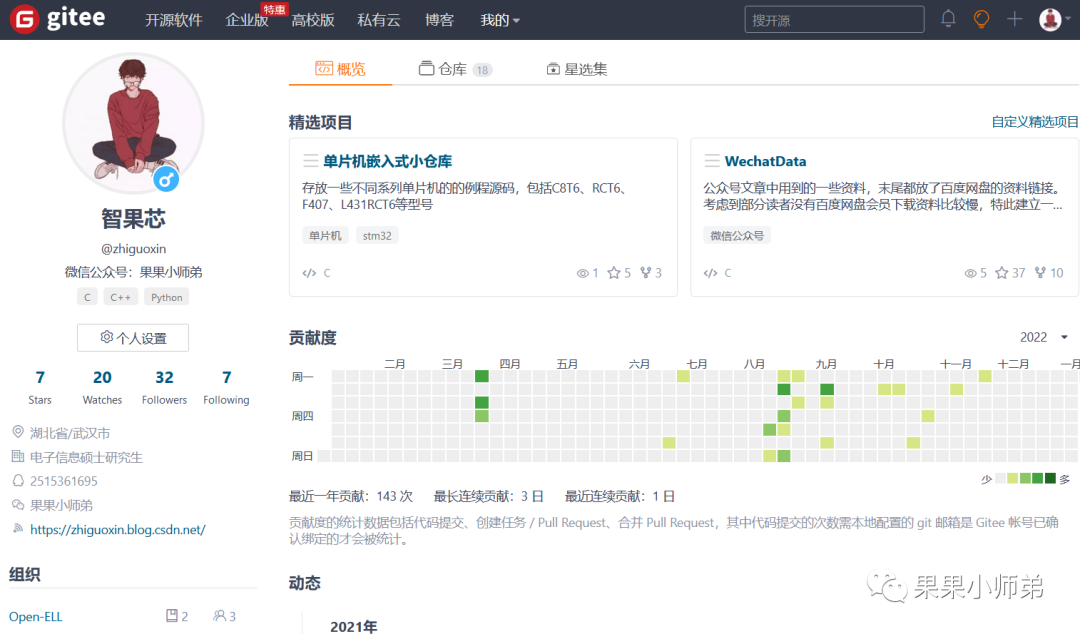
9. Provide Detailed Documentation for Code
In the fierce battle of software development, developers can easily focus on writing code and overlook the need for detailed explanations. Under pressure, documentation work often becomes the last task of the project, as developers perceive it as the final chore.
However, it is crucial to provide detailed explanations while the code is still fresh in your mind, as this allows developers or yourself to understand the comments and how the code works. If a series of changes made by a developer breaks the system, restoring the good version of the code is just a click away!
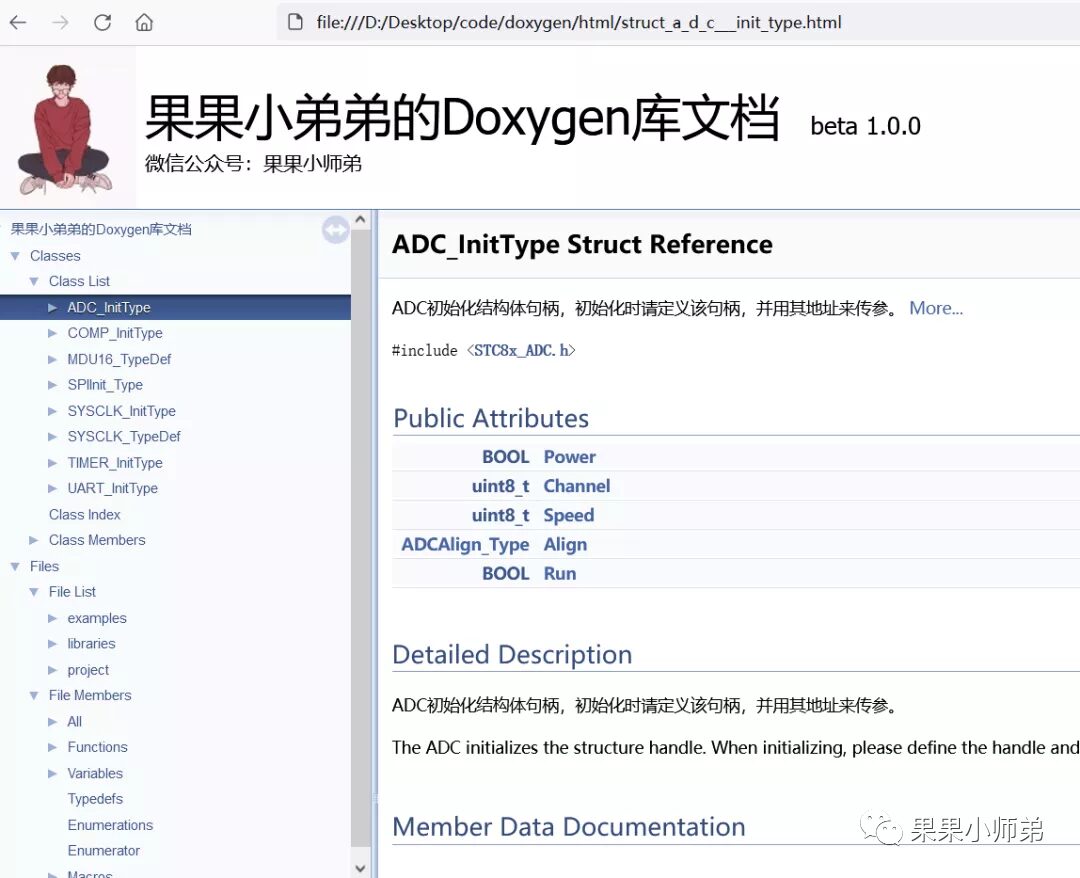
10. Learn Modular Programming and Driver Separation
When a project team undertakes a relatively complex project, it means you are no longer working alone. Instead, team members collaborate, each responsible for a part of the project. For example, you might only be responsible for communication or display.
At this point, you should write your portion of the program as a module, debug it separately, and leave interfaces for other modules to call. Finally, once all team members have completed and debugged their respective modules, the project leader will conduct integration debugging.
In such scenarios, modular programming is essential. The benefits of modularization are numerous; it not only facilitates division of labor but also aids in debugging, helps delineate program structure, and increases readability and portability.
Just remember the following four points:
-
The module is a combination of a .c file and a .h file; the header file (.h) contains declarations for the module’s interfaces; -
External functions and data provided by a module for other modules to call must be declared with the extern keyword in the .h file; -
Functions and global variables within a module must be declared with the static keyword at the beginning of the .c file; -
Never define variables in the .h file!