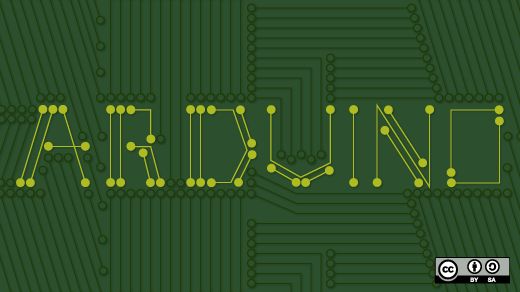
Using open-source hardware and software, a DIY plotter can automatically draw and engrave.
When I was in school, there was a HP plotter hidden in the science department’s closet. Although I could use it often during my school years, I still wanted to have my own plotter. Many years later, stepper motors became easy to obtain, and I was working with electronics and microcontrollers. Recently, I saw someone make a display using acrylic plastic. This inspired me to eventually create my own plotter.
My DIY plotter; see it in action in this video[1].
As a nostalgic person, I really love the original Arduino Uno[2]. Below is a list of other things I used (for reference; some of which I am not very satisfied with):
This is a personal project I designed. If you want to find a ready-made toolkit, you can find MaXYposi[10] from German Make magazine.
Hardware installation
As you can see, I initially made it too large. This plotter does not fit on my desk. But that’s okay; I just wanted to learn it (and I also remade some things; next time I will use a smaller beam).
Plotter base plate with X-axis and Y-axis tracks
The belt is mounted on the side of the track, and it connects some auxiliary wheels and motors:
Belt routing on the motor
I stacked several components on the Arduino. The Arduino is at the bottom, above it is the FabScan shield, then a StepStick protection device mounted on motor slots 1 and 2, and the SilentStepStick is on top. Note that the SCK and SDI pins are not connected.
Arduino stacked configuration (high-resolution image[11])
Be sure to connect the motor wires to the correct pins. If in doubt, check the datasheet or use a multimeter to find out which pair of wires is correct.
Software configuration
Basic part
While software like grbl[12] can interpret G-codes for device movements and other actions, and I can flash it into the Arduino, I am curious to understand better how it works. (My X-Y plotter software can be found on GitHub[13], but I provide no warranty.)
Using StepStick (or other compatible) drivers to drive the stepper motors basically only requires sending a high or low signal to the respective pins. Or using Arduino’s terminology:
digitalWrite(stepPin, HIGH);
delayMicroseconds(30);
digitalWrite(stepPin, LOW);
At the position of stepPin
is the pin number for the stepper motor: 3 is for motor 1, and 6 is for motor 2.
Before the stepper motor can work, it must first be enabled.
digitalWrite(enPin, LOW);
In fact, StepStick can understand three states of the pin:
Once the motor is enabled, its coils have power and are used to hold position. At this point, it is almost impossible to turn its shaft by hand. This ensures good precision, but it also means that both the motor and driver chip are “filled” with power and will therefore heat up.
Finally, and importantly, we need a way to determine the plotter’s direction:
digitalWrite(dirPin, direction);
The table below lists the functions and pins:
Function | Motor 1 | Motor 2 |
---|---|---|
Enable | 2 | 5 |
Direction | 4 | 7 |
Step | 3 | 6 |
Before we use these pins, we need to set their OUTPUT
mode in the code’s setup()
section.
pinMode(enPin1, OUTPUT);
pinMode(stepPin1, OUTPUT);
pinMode(dirPin1, OUTPUT);
digitalWrite(enPin1, LOW);
Once we understand this knowledge, we can easily move the stepper motor around:
totalRounds = ...
for (int rounds =0 ; rounds < 2*totalRounds; rounds++) {
if (dir==0){ // set direction
digitalWrite(dirPin2, LOW);
} else {
digitalWrite(dirPin2, HIGH);
}
delay(1); // give motors some breathing time
dir = 1-dir; // reverse direction
for (int i=0; i < 6400; i++) {
int t = abs(3200-i) / 200;
digitalWrite(stepPin2, HIGH);
delayMicroseconds(70 + t);
digitalWrite(stepPin2, LOW);
delayMicroseconds(70 + t);
}
}
This will make the slider move left and right. This code only manipulates one stepper motor, but for an X-Y plotter, we need to consider two axes.
Command interpreter
I started making a simple command interpreter to use standardized paths, such as:
"X30|Y30|X-30 Y-30|X-20|Y-20|X20|Y20|X-40|Y-25|X40 Y25
Describing relative movements in millimeters (1 millimeter equals 80 steps).
The plotter software implements a continuous mode, which allows a PC to provide it with a large path (many paths) to draw. (In this video[14], it shows how to draw a Hilbert curve)
Designing a good pen holder
In the first image above, the plotter pen is tied to the Y-axis with a thin string. This makes drawing inaccurate and also does not allow for lifting and lowering the pen in the software (as shown by the big black dot in the example).
Therefore, I designed a better, more precise pen holder that uses a servo to lift and lower the pen. You can see this new and improved pen holder in the image below; the Hilbert curve in the video link above was drawn using it.
The close-up in the image shows the servo arm lifting the pen
The pen is secured with a small clamp (the image shows a size 8 clamp, which is generally used to secure cables to walls). The servo arm can lift the pen; when the servo arm is lowered, the pen will be put down.
Driving the servo
Driving the servo is very simple: just provide a position, and the servo will do all the work.
#include <Servo.h>
// Servo pin
#define servoData PIN_A1
// Positions
#define PEN_UP 10
#define PEN_DOWN 50
Servo penServo;
void setup() {
// Attach to servo and raise pen
penServo.attach(servoData);
penServo.write(PEN_UP);
}
I connected the servo connector to motor 4 on the FabScan shield, so I will use analog pin 1.
Lowering the pen is also easy:
penServo.write(PEN_DOWN);
Further extensions
One of my further extensions is to add some limit detectors, but I can also do without them and instead use the TMC2130’s StallGuard mode. These detectors can also be used to implement a home
command.
In the future, I might also add a real Z-axis so it can mill or engrave wood, drill a PCB, or engrave a piece of acrylic, or… (I also thought about using a laser).
This article was originally published on the Some Things to Remember[15] blog and is authorized for redistribution.
via: https://opensource.com/article/18/3/diy-plotter-arduino
Author: Heiko W.Rupp[17] Translator: qhwdw Proofreader: wxy
This article is originally compiled by LCTT and honorably launched by Linux China